Do you want to display form entries on a page within your site to show users completed form submissions publicly? This tutorial will walk you through how to create and implement this functionality on your WordPress site.
By default, only pro users can view form entries from within the WordPress admin based on the Access Controls assigned to their user. However, you can easily create the functionality to display form entries on your site’s frontend.
Creating the Shortcode
First, we’ll provide you a code snippet that will need to be added to your site so that you can easily re-use this same shortcode with the exception of just updating the form ID as you need it.
Just add the following code snippet to your site. If you’re not sure how to do this, please review this tutorial.
/**
* Custom shortcode to display WPForms form entries in table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exists, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<table class="wpforms-frontend-entries">';
echo '<thead><tr>';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<th>';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</th>';
}
echo '</tr></thead>';
echo '<tbody>';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<tr>';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<td>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</td>';
}
echo '</tr>';
}
echo '</tbody>';
echo '</table>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
What this code snippet is going to do is allow you to use this shortcode anywhere on your site that accepts shortcodes such as pages, posts, and widget areas.
Just by using this [wpforms_entries_table id="FORMID"]
, your visitors will see entries submitted for the form you specify in id=”FORMID”.
A form’s ID number can be found by going to WPForms » All Forms and looking to the number in the Shortcode column for each form.
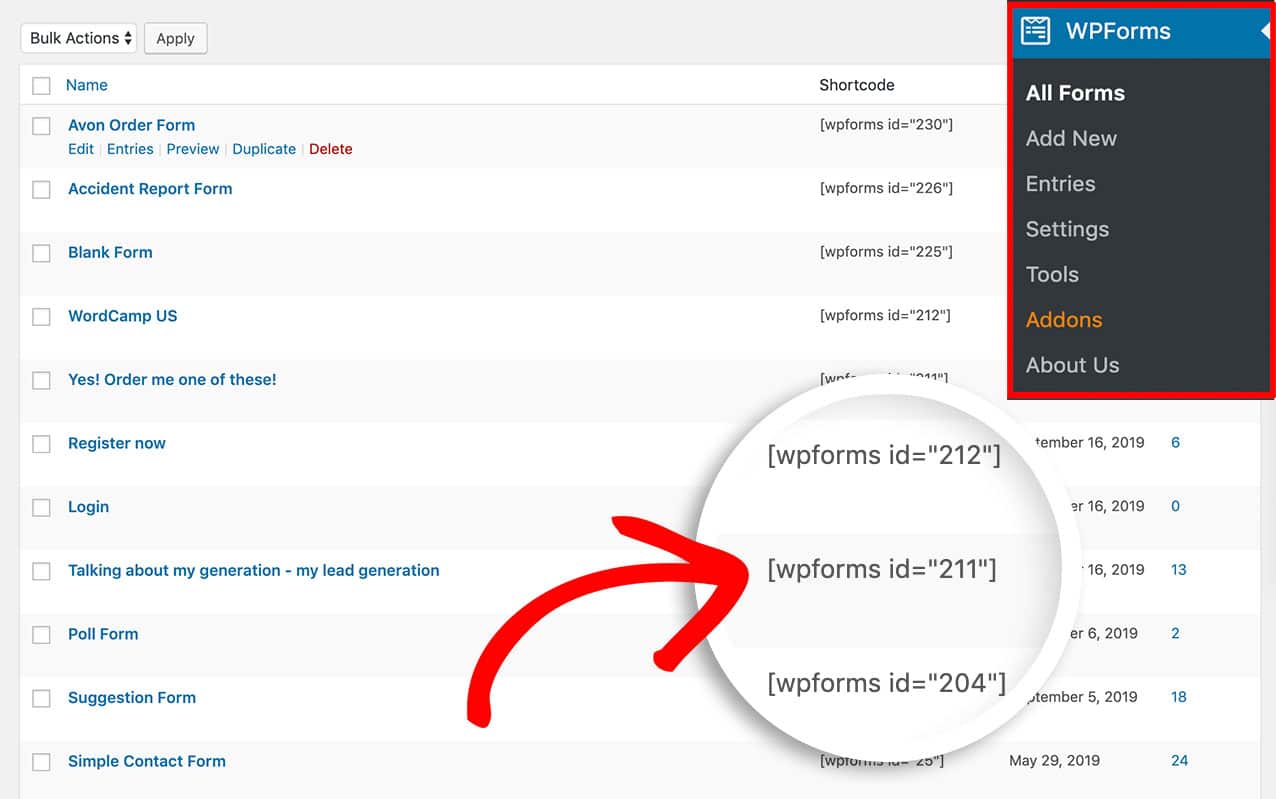
Displaying Form Entries
After creating the shortcode, you’ll need to add it to a page or post on your site to display your entries.
First, you’ll need to determine what form ID you want to display these entries on. In our example, we’re going to display entries for form ID 211 so our shortcode looks like this.
[wpforms_entries_table id="211"]
The only required attribute to use the shortcode is the id, without defining this in your shortcode, nothing will display so the form ID must be listed inside the shortcode.
However, you can filter down your entries even further by defining some of the attributes of the shortcode and we’re going to review each available attribute.
Defining the type Attribute
Using the type attribute can be used if you want to show only starred entries. Here’s an example.
[wpforms_entries_table id="211" type="starred"]
Below are other available type parameters you can use:
- all – using this attribute will display all entry types.
- read – this attribute will only display entries that have been viewed by an admin.
- unread – by using this attribute, you’ll only see entries that haven’t been opened by an admin on your site.
- starred – this attribute will only display entries that have been starred by an admin
Utilizing the user Attribute
If a user is currently logged into your site and you only want them to see the form entries they’ve submitted, use this shortcode.
[wpforms_entries_table id="FORMID" user="current"]
If the user isn’t logged in, all the entries for this form would display.
If the user is logged in but hasn’t completed the form (while logged in) then No results found. would display on the page.
Defining the fields Attribute
To only display certain fields, you’ll first need to find out the IDs for the fields you want to display. To find those field IDs, please review this tutorial.
Once you know what the field IDs are, you’ll use this as your shortcode. In this example, we only want the Name field which is field ID 0 and the Comments field which is field ID 2.
[wpforms_entries_table id="211" fields="0,2"]
Note: Multiple field IDs are separated using a comma in the shortcode.
Using the number Attribute
By default, the shortcode will only display the first 30 entries. However, if you’d like to display a smaller amount, you’ll need to use the number
parameter. For example, if you’d like to show the first 20 entries, add your shortcode like this.
[wpforms_entries_table id="211" number="20"]
The number=”20″ variable passed inside the shortcode will determine how many entries the table will display. If you want to show all entries, change the number to 9999.
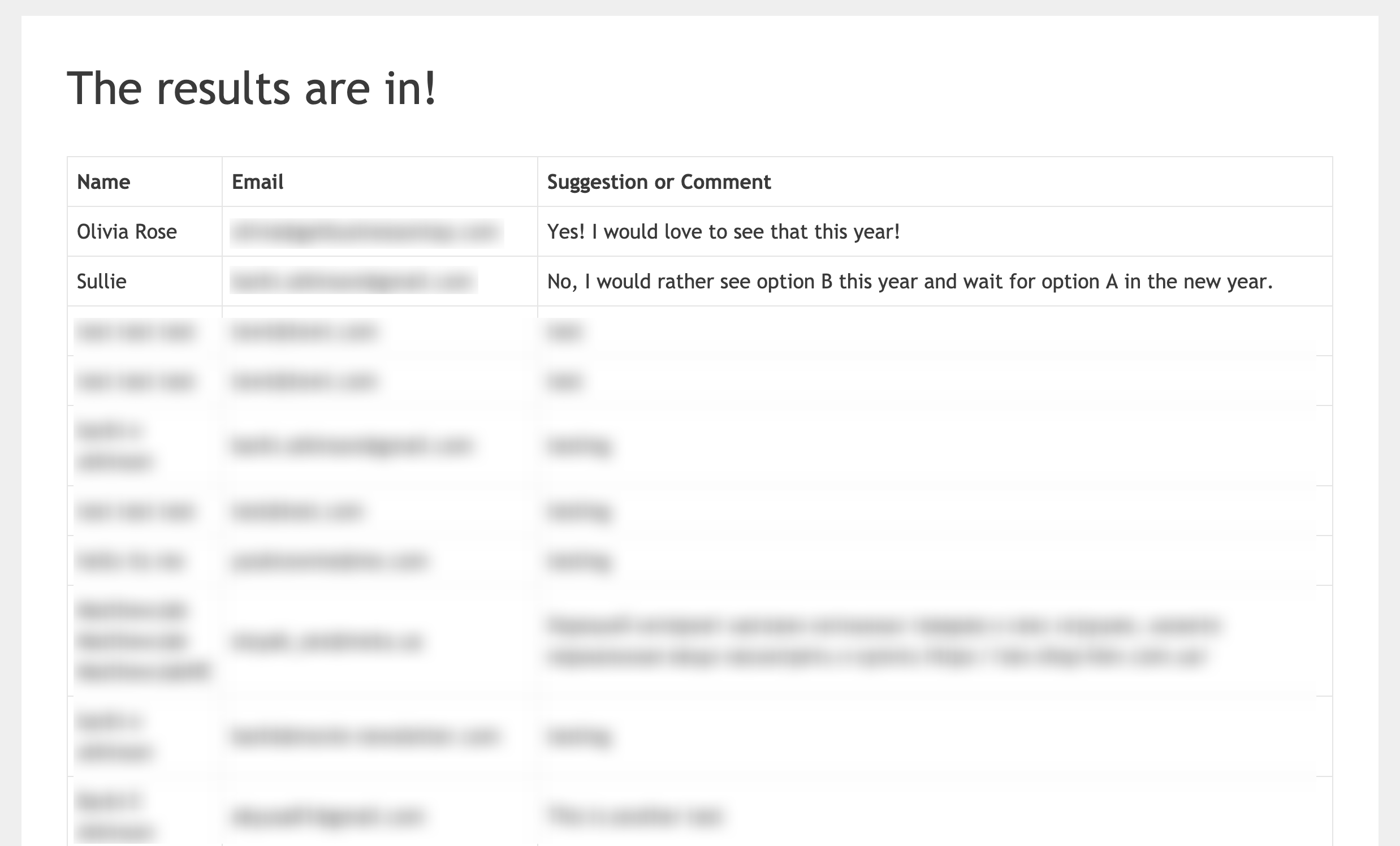
Using the sort and order Attribute
When using your shortcode you can tell the shortcode which field you want to sort the table by and then define if you want it sorted by asc (ascending order) or desc (descending order). An example of that shortcode would look like this.
[wpforms_entries_table id="211" sort="1" order="asc"]
In this example, we’re going to display a table of entries for the form ID 211 and we’re going to sort this table in asc (ascending) order using the field ID 1, which is the field ID for the Name field of the form.
Styling the Table (Optional)
Styling the entries table is completely optional as most themes have default table styling built-in as do browsers as well. However, we wanted to get you started with an example, so if you want to change the default table styling, you’ll want to copy and paste this CSS to your site.
For any assistance on how and where to add CSS to your site, please review this tutorial.
The styling of your entry table will vary depending on the default styling your theme may or may not have for tables.
table {
border-collapse: collapse;
}
thead tr {
height: 60px;
}
table, th, td {
border: 1px solid #000000;
}
td {
white-space: normal;
max-width: 33%;
width: 33%;
word-break: break-all;
height: 60px;
padding: 10px;
}
tr:nth-child(even) {
background: #ccc
}
tr:nth-child(odd) {
background: #fff
}
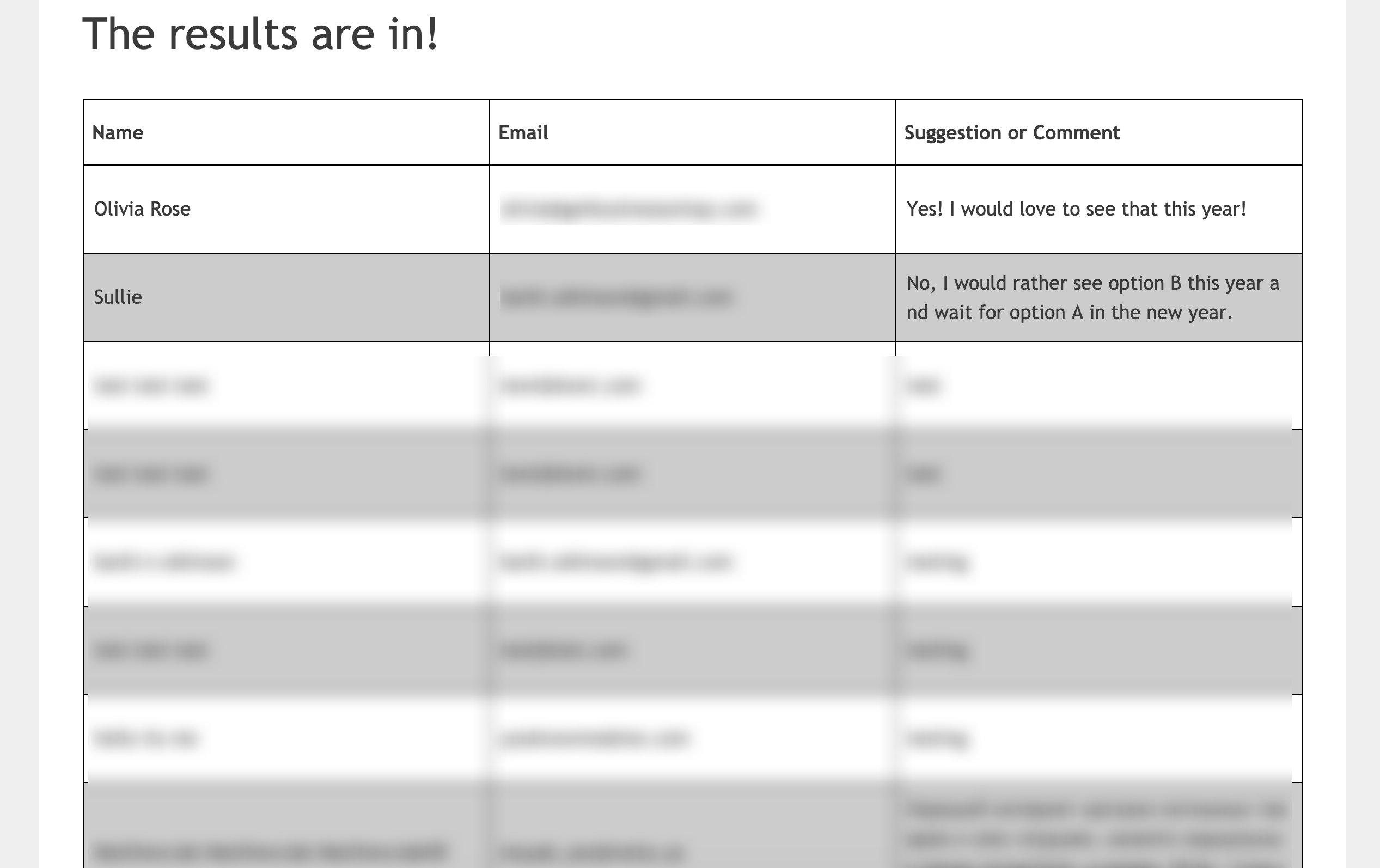
Adding a Non-table View
If you would prefer to not use an HTML table view to display your entries, we’ve got an alternative solution for you as well. Instead of creating your shortcode with the code snippet above, use this code snippet instead.
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="header-row">';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<span class="column-label">';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</span>';
}
echo '</div>';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
However, it’s important to note here that since we’re not using a standard table, there will be no default styling applied when using this option, so the CSS in the next step is vital but we’ll list out the CSS classes used so that you can apply your own styling to this option.
Styling the Non-table
Below is a detailed list of the CSS classes used and what their purpose can be (if needed).
- .wrapper – this is an overall wrapper around the entire block of entries and can be used if you would like to reduce the width of the block so it doesn’t stretch 100% of your page.
- .header-row – this is another overall wrapper around the labels of each column (only the labels) if you would wish to change the background color of label section.>
- .column-label – this wrapper will wrap around each individual label title. For example, Name, Email Address and Suggestion/Comment each has one of these wrappers surrounding them and could be used to target the size of this text.
- .entries – this is an overall wrapper around the entries themselves if you would wish to change the background color of this section to appear differently than the labels.
- .entry-details – this is a overall wrapper around each individual entry and could be useful if you would like to provide a different color background for each entry row.
- .details – each column of information is wrapped in this class so that you can add any additional styling you wish such as font-size, color etc.
You can use the CSS below to add some default styling for your non-table view.
For any assistance on how and where to add CSS to your site, please review this tutorial.
.wrapper {
width: 100%;
clear: both;
display: block;
}
.header-row {
float: left;
width: 100%;
margin-bottom: 20px;
border-top: 1px solid #eee;
padding: 10px 0;
border-bottom: 1px solid #eee;
}
span.details, span.column-label {
display: inline-block;
float: left;
width: 33%;
margin-right: 2px;
text-align: center;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.header-row span.column-label {
text-transform: uppercase;
letter-spacing: 2px;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
float: left;
margin: 20px 0;
padding-bottom: 20px;
}
Note: The CSS provided above is based on only displaying 3 fields from your entry, the Name, Email and Comments field. If you would like to display more fields, please remember to update your CSS accordingly.
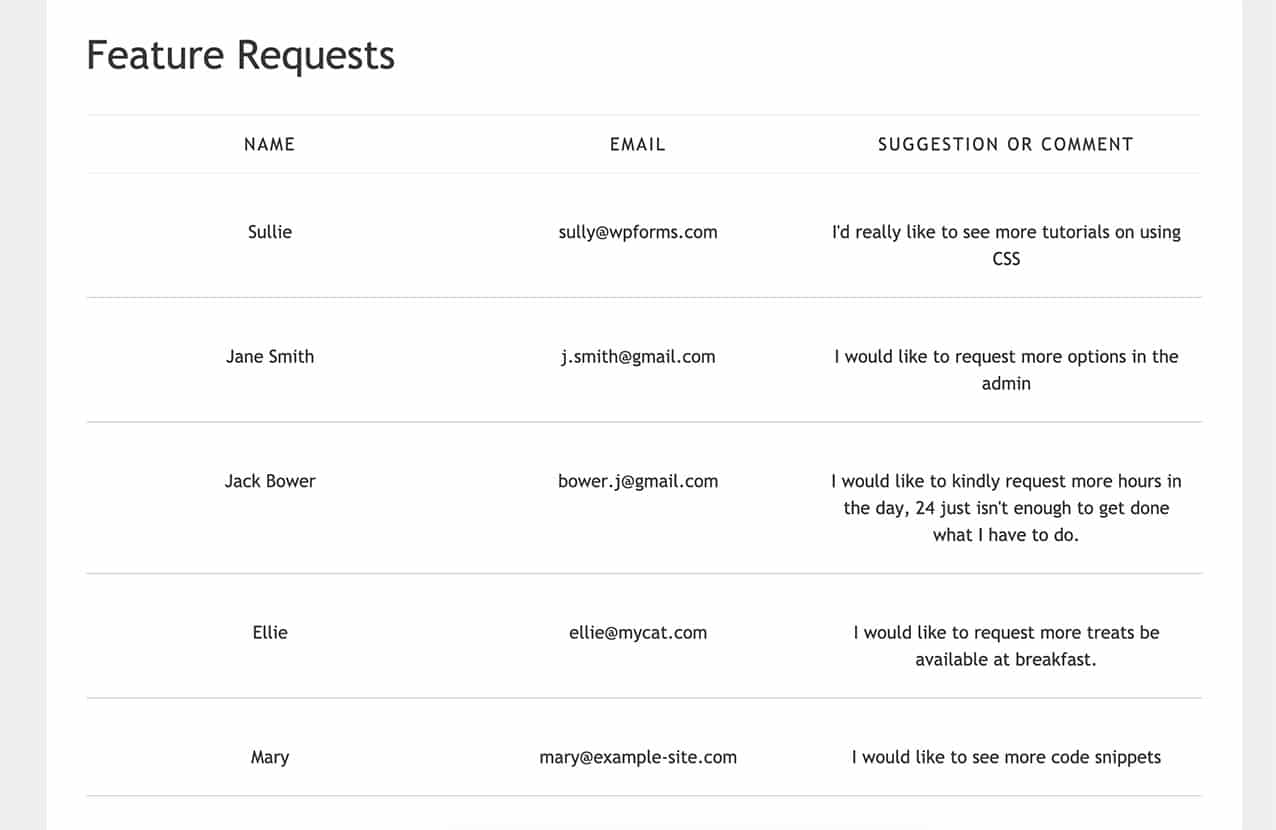
And that’s it, you’ve now created a shortcode that can be added in various places on your site so that you can display your form entries to non-admin users. Would you also like to display how many entries are left on your form when using the Form Locker addon? Try out our code snippet for How to Display Remaining Entry Limit Number.
Reference Filter
FAQ
These are answers to some of the top questions we see about displaying form entries on a page or post in WordPress.
Q: How can I display just a count of unread entries?
A: You would add this snippet to your site.
<?php
/**
* Custom shortcode to display WPForms form entries count for a form.
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
*/
function wpf_dev_entries_count( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'type' => 'all', // all, unread, read, or starred.
],
$atts
);
if ( empty( $atts['id'] ) ) {
return;
}
$args = [
'form_id' => absint( $atts['id'] ),
];
if ( $atts['type'] === 'unread' ) {
$args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$args['starred'] = '1';
}
return wpforms()->entry->get_entries( $args, true );
}
add_shortcode( 'wpf_entries_count', 'wpf_dev_entries_count' );
Then you are free to use this shortcode anywhere on your WordPress site where shortcodes are accepted.
[wpf_entries_count id="13" type="unread"]
You can just change the type to be unread, read or starred.
Q: Why aren’t my newer entries showing?
A: This is likely due to caching either on the server level, site level, or browser level. Please check that your cache is completely clear.
Q: Do I have to have columns at all?
A: No, not at all. You can display your entries by placing the label in front of the field value and having each field on a separate line using this snippet and CSS.
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts['id'] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts['id'] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts['fields'] ) && $atts['fields'] !== '' ? explode( ',', str_replace( ' ', '', $atts['fields'] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data['fields'];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data['fields'][$field_id] ) ) {
$form_fields[$field_id] = $form_data['fields'][$field_id];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field['type'], $form_fields_disallow, true ) ) {
unset( $form_fields[$field_id] );
}
}
$entries_args = [
'form_id' => absint( $atts['id'] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts['user'] ) ) {
if ( $atts['user'] === 'current' && is_user_logged_in() ) {
$entries_args['user_id'] = get_current_user_id();
} else {
$entries_args['user_id'] = absint( $atts['user'] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts['number'] ) ) {
$entries_args['number'] = absint( $atts['number'] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts['type'] === 'unread' ) {
$entries_args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$entries_args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$entries_args['starred'] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key]['fields'] = json_decode($entry['fields'], true);
$entries[$key]['meta'] = json_decode($entry['meta'], true);
}
if ( !empty($atts['sort']) && isset($entries[0]['fields'][$atts['sort']] ) ) {
if ( strtolower($atts['order']) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1['fields'][$atts['sort']]['value'], $entry2['fields'][$atts['sort']]['value']);
});
} elseif ( strtolower($atts['order']) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2['fields'][$atts['sort']]['value'], $entry1['fields'][$atts['sort']]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry['fields'];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
echo '<span class="label">' . esc_html( sanitize_text_field( $form_field['label'] ) ) . ':</span>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field['id'] ) === absint( $form_field['id'] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field['value'] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Use this CSS below for styling the non-table view.
For any assistance on how and where to add CSS to your site, please review this tutorial.
.wrapper {
width: 100%;
clear: both;
display: block;
}
span.details {
display: block;
margin-right: 2px;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
margin: 20px 0;
padding-bottom: 20px;
}
span.label {
font-weight: bold;
margin-right: 5px;
}
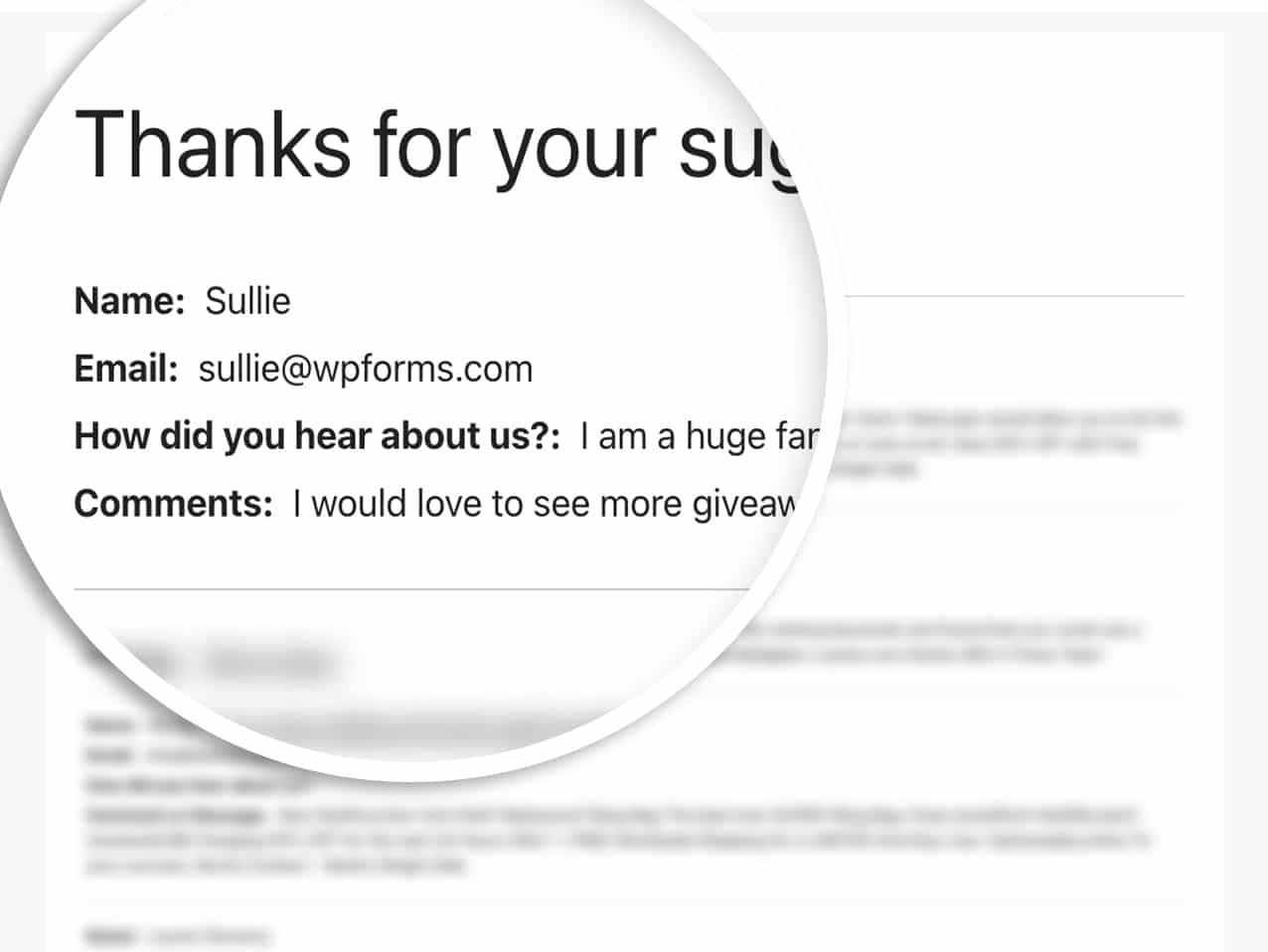