Would you like to set a minimum number of choices for your Checkbox form field? WPForms currently supports limiting the number of choices for the Checkbox field. However, with a custom snippet, you’ll be able to set a minimum number for these choices using a small PHP code snippet.
In this tutorial, we’ll walk you through the steps to set up your Checkbox form field to require a minimum number of selections.
Before getting started, make sure WPForms is installed and activated on your WordPress site and that you’ve verified your license.
Creating the Form
To begin, you’ll need to create a new form or edit an existing one to access the form builder. In the form builder, make sure to include at least one Checkbox field to your form.
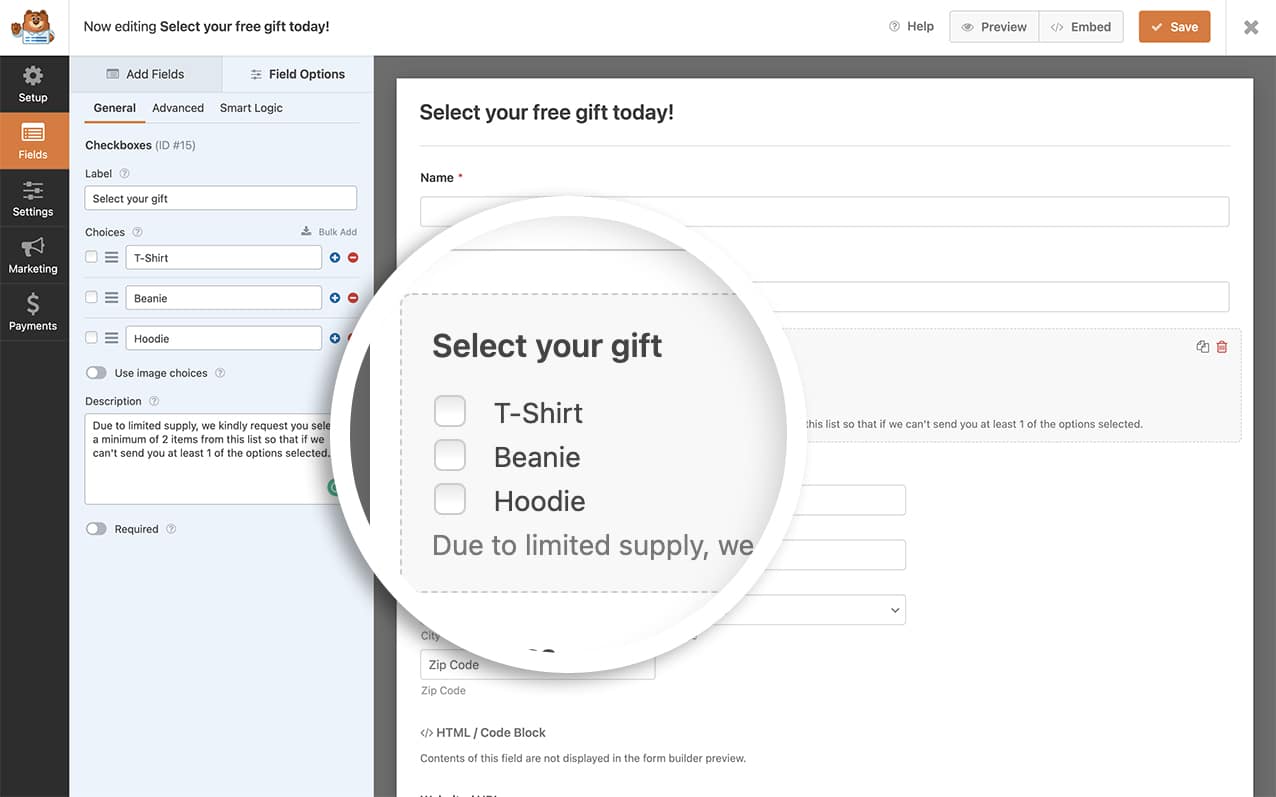
Adding the Snippet to Set a Minimum Number
To set a minimum number of choices, you’ll need to add this code snippet below to your site. If you need any assistance in adding code snippets to your site, please review this tutorial.
/**
* Set minimum number of choices for a checkbox for the field
*
* @link https://wpforms.com/developers/how-to-set-a-minimum-number-of-choices-for-a-checkbox/
*/
function wpf_checkbox_validation( $field_id, $field_submit, $form_data ) {
$field_submit = (array) $field_submit;
// Make sure we have an array of choices and count the number of choices.
$count_choices = is_array( $field_submit ) ? count( $field_submit ) : 0;
if ( $count_choices < 2 ) {
wpforms()->process->errors[ $form_data[ 'id' ] ][ $field_id ] = esc_html__( 'Please select at least 2 options', 'your-text-domain' );
}
}
add_action( 'wpforms_process_validate_checkbox', 'wpf_checkbox_validation', 10, 3 );
In the code above, any checkbox will require a minimum of two choices. If you’d like to update the minimum number, change the value in the if statement.
Note: This snippet will apply to the Checkbox field on all forms on your site.
When a user selects fewer than two choices on your form, a message will be displayed under the Checkbox field when the form is submitted.
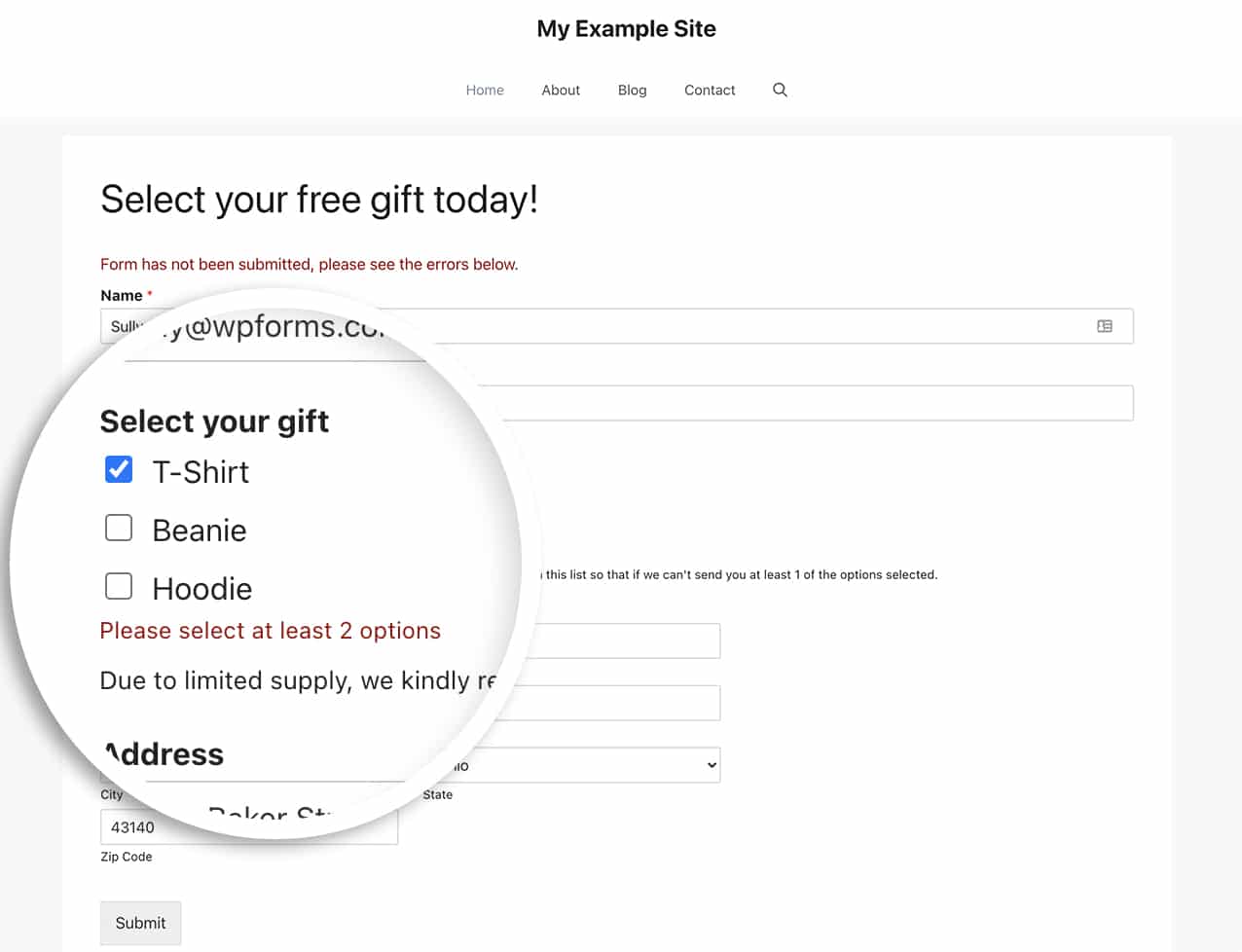
Setting a Limit for Multi-page Forms
When using multi-page forms, you may not want to wait for the form submission before displaying the error.
You can easily show a warning message under the Checkbox field when the Next button is clicked for multi-page forms.
To use this option, simply add this code snippet to your site instead.
/**
* Set minimum number of choices for a checkbox form field
*
* @link https://wpforms.com/developers/how-to-set-a-minimum-number-of-choices-for-a-checkbox-field/
*/
function wpf_dev_min_checkbox() {
?>
<script type="text/javascript">
jQuery(document).ready(function($) {
var checkbox_forms = [
{form_id: 1000, min_count: 2, field_ids: [10,9]},
];
$('[id^=wpforms-] input[type=checkbox]').on('click', function(e){
var this_form_id = $(this).closest('form').data("formid");
var checkbox_form_ids = checkbox_forms.map(form => form.form_id);
if (checkbox_form_ids.includes(this_form_id)){
var checkbox_container = $(this).closest(".wpforms-field-checkbox");
var checkbox_container_field_id = checkbox_container.data("field-id");
checkbox_forms.forEach(function(form) {
if (this_form_id == form.form_id){
var min_value = form.min_count;
var field_ids_array = form.field_ids;
if (field_ids_array.includes(checkbox_container_field_id)){
validate_checkbox_min(e, checkbox_container, min_value);
}
}
});
}
});
function validate_checkbox_min(e, checkbox_container, min){
var minMaxSelection = checkbox_container.find('input[type=checkbox]:checked');
var this_field = $('[id^=wpforms-' + checkbox_container.data("field-id") + '-field_' + checkbox_container.data("field-id") + '-container]');
var warning_id = 'wpforms-' + checkbox_container.data("field-id") + '-warning';
$("#" + warning_id).remove();
if (minMaxSelection.length > 0 && minMaxSelection.length < min) {
e.stopPropagation();
this_field.addClass("wpforms-error");
this_field.removeClass("wpforms-valid");
this_field.attr('aria-invalid', 'true');
checkbox_container.addClass("wpforms-has-error");
checkbox_container.append('<em id="' + warning_id + '" class="wpforms-error" role="alert" aria-label="Error message" for="">Please select at least ' + min + ' options</em>');
} else {
this_field.removeClass("wpforms-error");
this_field.addClass("wpforms-valid");
this_field.attr('aria-invalid', 'false');
checkbox_container.removeClass("wpforms-has-error");
}
}
});
</script>
<?php
}
add_action('wpforms_wp_footer_end', 'wpf_dev_min_checkbox');
This code snippet will target the form ID 1000
and when the Next button is clicked, the snippet will first check to see how many choices are selected for the field ID 10
and 9
, and if it’s less than two, it will show an alert message in the browser.
Frequently Asked Questions
Below, we’ve answered some of the top questions we see about setting a minimum number of choice for the Checkbox field.
Q: Can I use this only a specific form and a specific field ID?
A: Absolutely! To use this code on a specific form, use this code snippet below:
/**
* Set minimum number of choices for a checkbox form field
*
* @link https://wpforms.com/developers/how-to-set-a-minimum-number-of-choices-for-a-checkbox/
*/
function wpf_dev_checkbox_validation( $field_id, $field_submit, $form_data ) {
// Change the number to match your form ID
if ( absint( $form_data[ 'id' ] ) !== 1289 ) {
return;
}
$field_submit = (array) $field_submit;
// Make sure we have an array of choices and count the number of choices.
$count_choices = is_array( $field_submit ) ? count( $field_submit ) : 0;
if ( $count_choices < 2 ) {
wpforms()->process->errors[ $form_data[ 'id' ] ][ $field_id ] = esc_html__( 'Please select at least 2 options', 'your-text-domain' );
}
}
add_action( 'wpforms_process_validate_checkbox', 'wpf_dev_checkbox_validation', 10, 3 );
If you’d like to target a specific form and a specific field ID, use this code snippet.
/**
* Set minimum number of choices for a checkbox form field
*
* @link https://wpforms.com/developers/how-to-set-a-minimum-number-of-choices-for-a-checkbox/
*/
function wpf_dev_checkbox_validation( $field_id, $field_submit, $form_data ) {
// Change the number to match your form ID
if ( absint( $form_data[ 'id' ] ) !== 1289 ) {
return $field_id;
}
// Change this number to match the field ID
if (absint($field_id) === 15 ) {
$field_submit = (array) $field_submit;
// Make sure we have an array of choices and count the number of choices.
$count_choices = is_array( $field_submit ) ? count( $field_submit ) : 0;
if ( $count_choices < 2 ) {
wpforms()->process->errors[ $form_data[ 'id' ] ][ $field_id ] = esc_html__( 'Please select at least 2 options', 'your-text-domain' );
}
}
}
add_action( 'wpforms_process_validate_checkbox', 'wpf_dev_checkbox_validation', 10, 3 );
Note: If you need any help in finding your form and field IDs, please review this tutorial.
Q: Can this work for payment fields as well?
A: Absolutely! To use this for Payment Checkbox Items form field, use this snippet.
/**
* Set minimum number of choices for a payment checkbox form field
*
* @link https://wpforms.com/developers/how-to-set-a-minimum-number-of-choices-for-a-checkbox/
*/
function wpf_dev_limit_payment_field( $field_id, $field_submit, $form_data ) {
$form_id = 731; // Limit to form ID: Use 0 to target all forms
$fieldID = 21; // Limit to field ID: Use 0 to target all checkbox fields
$min = 2; // Change the minimum amount
$max = 5; // Change the maximum amount
// Limit to specific form if {$form_id} is set
if ( absint( $form_data[ 'id' ] ) !== $form_id && !empty( $form_id ) ) {
return;
}
// Limit to specific field ID if {$fieldID} is set
if ( absint( $field_id ) !== $fieldID && !empty( $fieldID ) ) {
return;
}
// Make sure we have an array of choices and count the number of choices.
$count = is_array( $field_submit ) ? count( $field_submit ) : 0;
// Process error if choices count is less than {$min} or greater than {$max}
if( count( $field_submit ) < $min ) {
wpforms()->process->errors[ $form_data[ 'id' ] ] [ $field_id ] = esc_html__( 'Please select a minimum of ' . $min .' choices.', 'your-text-domain' );
} elseif ( count( $field_submit ) > $max ) {
wpforms()->process->errors[ $form_data[ 'id' ] ] [ $field_id ] = esc_html__( 'Please select a maximum of ' . $max . ' choices.', 'your-text-domain' );
}
}
add_action( 'wpforms_process_validate_payment-checkbox', 'wpf_dev_limit_payment_field', 10, 3 );
That’s it! You’ve now learned how to set a minimum number of choices on your checkboxes.
Next, would you like to customize the look of these checkboxes? Take a look at our guide on customizing the Checkbox and Multiple Choice fields to look like buttons.
Related
Action references: