Would you like to send metadata to Stripe when payments are processed through your form? This is particularly helpful if you’d like to add some tags that you can easily identify in your Stripe payments.
In this tutorial, we’ll show you the PHP snippet you need to send metadata as part of the payment through WPForms.
Before getting started, make sure WPForms is installed and activated on your site and that you’ve verified your license.
Creating a Payment Form
First, you’ll need to create a new payment form or edit an existing one to access the form builder. In the form builder, go ahead and add the Stripe Credit Card field to your form.
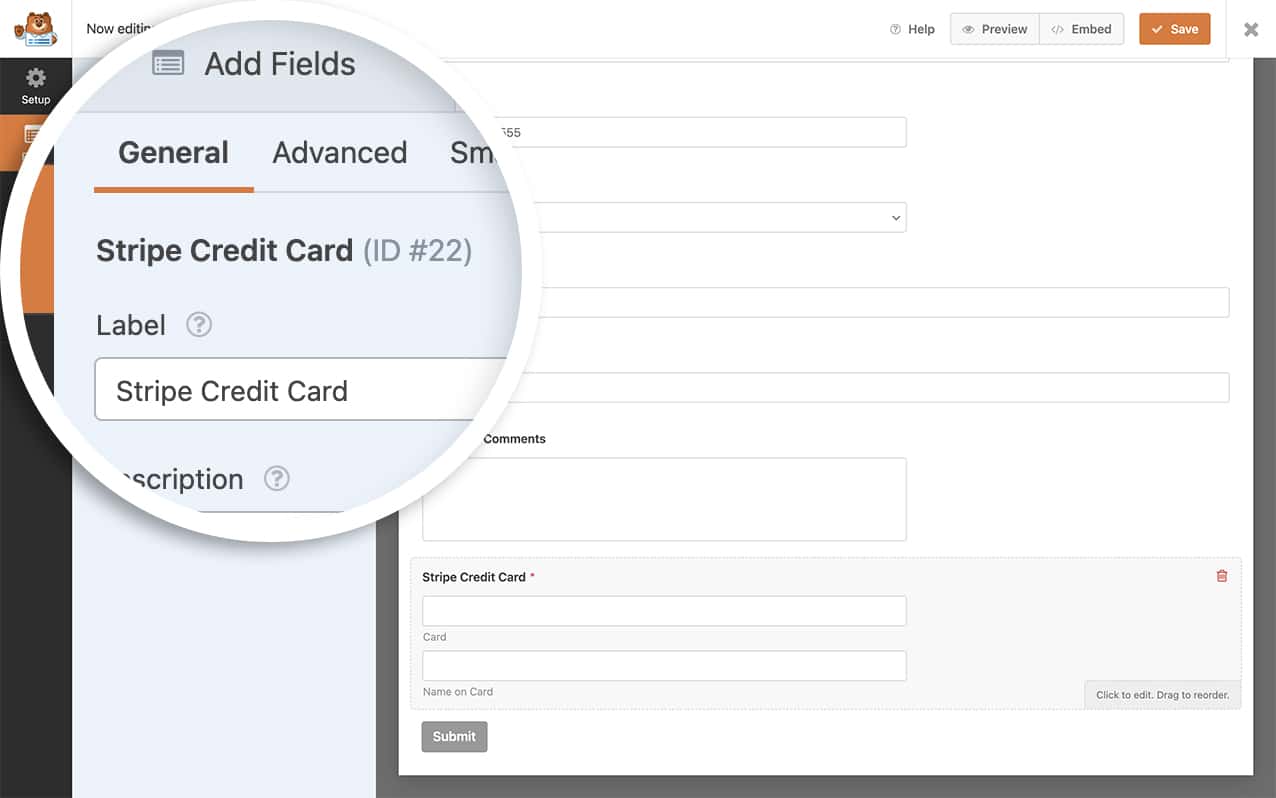
You’ll also need to set up your form to accept Stripe payments. If you need assistance in setting up Stripe on your form, please review this documentation.
Adding the Snippet to Send Metadata to Stripe
Now it’s time to add the snippet to your site that will send through the metadata when Stripe payments are processed.
If you need any assistance with adding snippets to your site, please see this tutorial.
Adding for Both Single Payments and Initial Subscription Payments
/**
* Send metadata to Stripe when payment is processed for single payments and initial subscription payments.
*
* @link https://wpforms.com/developers/how-to-send-metadata-to-stripe-payments/
*/
function wpf_stripe_single_payment_and_initial_subscription_payment_custom_metadata( $fields, $form_data, $entry_id, $payment ) {
if ( ! ( $payment instanceof \WPForms\Vendor\Stripe\PaymentIntent ) && ! ( $payment instanceof \WPForms\Vendor\Stripe\Charge ) ) {
return;
}
// Check if the field with id equals 3 value exists and assign it.
if ( isset( $fields[3][ 'value' ] ) ) {
$payment->metadata[ 'something' ] = $fields[3][ 'value' ];
}
// Assign fixed value (no additional checks required).
$payment->metadata[ 'key' ] = 'some value for single payment';
$payment->save();
}
add_action( 'wpforms_stripe_process_complete', 'wpf_stripe_single_payment_and_initial_subscription_payment_custom_metadata', 10, 4 );
In the above code snippet, we’re sending through two forms of metadata. One is assigned from a specific form field with $fields[3][ 'value' ]
. This means that whatever value is entered in the form field that has the ID of 3, we’ll grab that and send it to Stripe.
The metadata[ 'key' ] = 'some value for single payment';
is also being sent but rather than grab some value from the form the user filled in, we’re actually setting this ourselves in the code itself.
You’ll need to remember to change each of those to match what you need in your snippet. For example, you’ll need to change the field ID 3 to match the field ID you want to send, as well as the text of some value for single payment.
Note: If you need help in where to find your field IDs or if you want to target a specific form and need help finding the form ID, please review this tutorial.
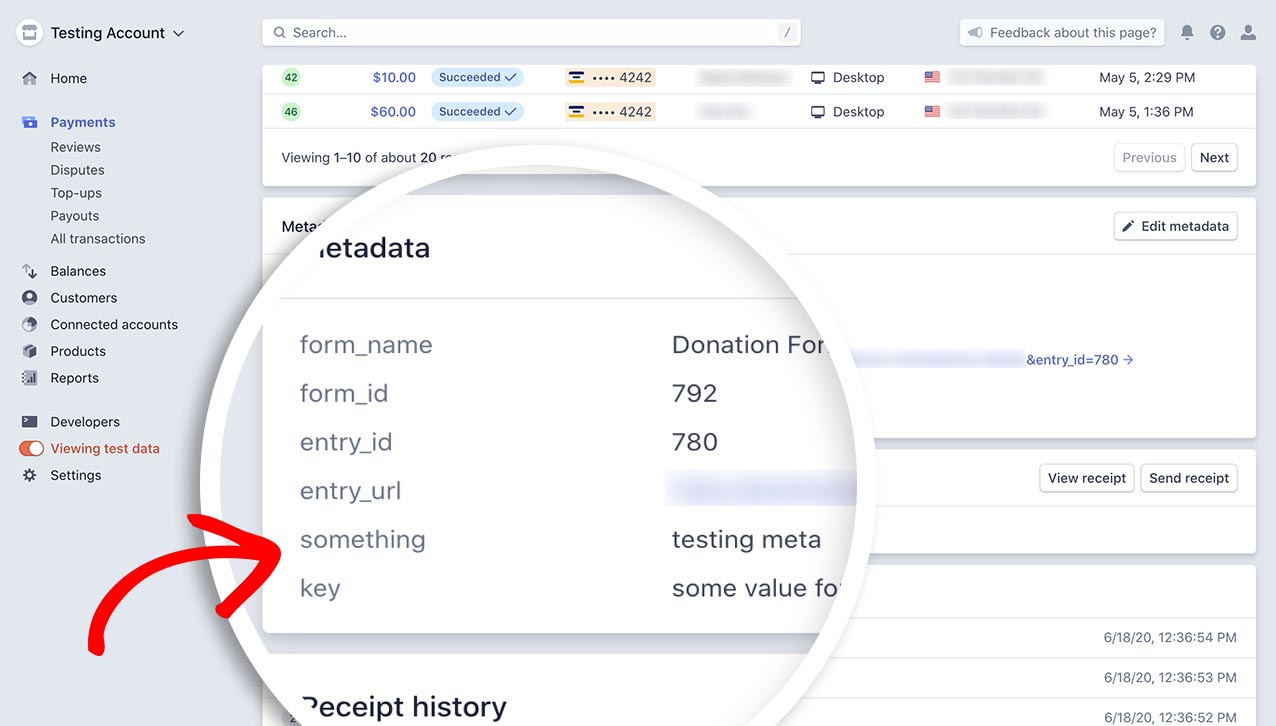
Adding for Single Payments Only
/**
* Send metadata to Stripe when payment is processed for single payments only.
*
* @link https://wpforms.com/developers/how-to-send-metadata-to-stripe-payments/
*/
function wpf_stripe_single_payment_custom_metadata( $fields, $form_data, $entry_id, $payment, $subscription ) {
if ( ! empty( $subscription->id ) ) {
return;
}
if ( ! ( $payment instanceof \WPForms\Vendor\Stripe\PaymentIntent ) && ! ( $payment instanceof \WPForms\Vendor\Stripe\Charge ) ) {
return;
}
// Check if the field with id equals 3 value exists and assign it.
if ( isset( $fields[3][ 'value' ] ) ) {
$payment->metadata[ 'something' ] = $fields[3][ 'value' ];
}
// Assign fixed value (no additional checks required).
$payment->metadata[ 'key' ] = 'some value for single payment';
$payment->save();
}
add_action( 'wpforms_stripe_process_complete', 'wpf_stripe_single_payment_custom_metadata', 10, 5 );
Adding for Subscription Payments Only (Excluding Single Payments or Initial Subscription Payments)
/**
* Send metadata to Stripe when payment is processed for subscription payments (excluding single payments and initial subscription payments).
*
* @link https://wpforms.com/developers/how-to-send-metadata-to-stripe-payments/
*/
function wpf_stripe_subscription_custom_metadata( $fields, $form_data, $entry_id, $payment, $subscription ) {
if ( ! ( $subscription instanceof \WPForms\Vendor\Stripe\Subscription ) ) {
return;
}
// Check if the field with id equals 3 value exists and assign it.
if ( isset( $fields[3][ 'value' ] ) ) {
$subscription->metadata[ 'something' ] = $fields[3][ 'value' ];
}
// Assign fixed value (no additional checks required).
$subscription->metadata[ 'key' ] = 'some value for subscription';
$subscription->save();
}
add_action( 'wpforms_stripe_process_complete', 'wpf_stripe_subscription_custom_metadata', 10, 5 );
Frequently Asked Questions
These are answers to some of the top questions we see about using metadata with Stripe payments.
Q: How can I send multiple fields over and target a specific form?
A: To target one form and send multiple fields of metadata over, use the code snippet below.
/**
* Send metadata to Stripe when payment is processed based on form ID.
*
* @link https://wpforms.com/developers/how-to-send-metadata-to-stripe-payments/
*/
function wpf_stripe_single_payment_and_initial_subscription_payment_custom_metadata_multiple( $fields, $form_data, $entry_id, $payment ) {
// Only run this code on form ID 2614
if ( absint( $form_data[ 'id' ] ) !== 2614 ) {
return;
}
if ( ! ( $payment instanceof \WPForms\Vendor\Stripe\PaymentIntent ) && ! ( $payment instanceof \WPForms\Vendor\Stripe\Charge ) ) {
return;
}
// Capture the value listed in the field ID 0
if ( isset( $fields[0][ 'value' ] ) ) {
$payment->metadata[ 'Name' ] = $fields[0][ 'value' ];
}
// Capture the value listed in the field ID 1
if ( isset( $fields[1][ 'value' ] ) ) {
$payment->metadata[ 'Email' ] = $fields[1][ 'value' ];
}
// Capture the value listed in the field ID 2
if ( isset( $fields[2][ 'value' ] ) ) {
$payment->metadata[ 'Phone' ] = $fields[2][ 'value' ];
}
// Capture the value listed in the field ID 3
if ( isset( $fields[3][ 'value' ] ) ) {
$payment->metadata[ 'Address' ] = $fields[3][ 'value' ];
}
$payment->save();
}
add_action( 'wpforms_stripe_process_complete', 'wpf_stripe_single_payment_and_initial_subscription_payment_custom_metadata_multiple', 10, 4 );
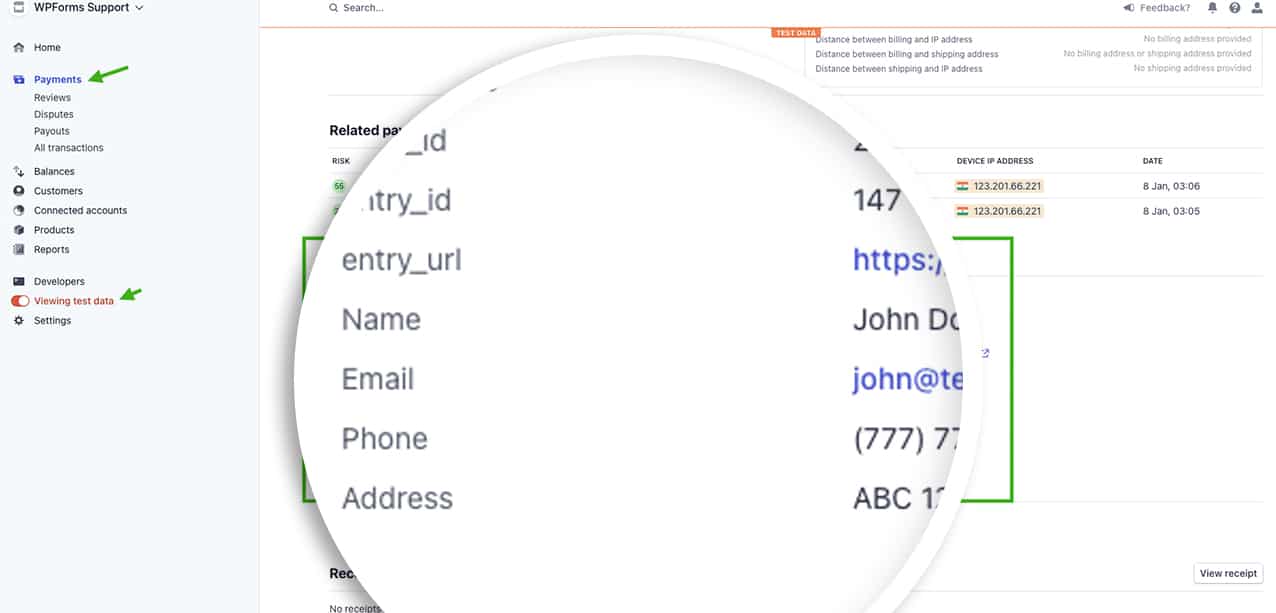
Note: Make sure to change the form and field ID numbers to match your own IDs.
That’s it! You’ve now learned how to successfully send metadata to Stripe from WPForms.
Next, would you like to change the sublabels on the Stripe Credit Card field? Be sure to check our guide to learn how to change sublabels for the Stripe Credit Card field.
Related
Action Reference: wpforms_stripe_process_complete