Would you like to customize the date time field date options in WPForms? The Date / Time field includes two format options for the date selector: Date Picker, which provides the user with a calendar, or Date Dropdown.
With the release of version 1.6.3, WPForms gives you options inside the form builder to Limit Days and even Disable Past Dates as well as Limit Hours. If this is all you need to do, please review this documentation.
This tutorial will focus more on customizing the Date Dropdown field and calculating dates blocks for the Date Picker.
It’s important to remember that if you are using any of the code snippets in this doc, the form builder options for limiting should be turned off. To find out more about the built-in options for limiting the dates, please check out this documentation.
Creating the form
Before we add any snippets, you’ll need to create your form and add your fields. Since this tutorial is based on the Date Dropdown field, you’ll need to add at least one Date field.
If you need any assistance in creating your form, please see this tutorial.
Once you’ve added your date fields, make sure you’ve selected the Type to Date Dropdown. You can set the Date Type by clicking into the Advanced options of the form field.
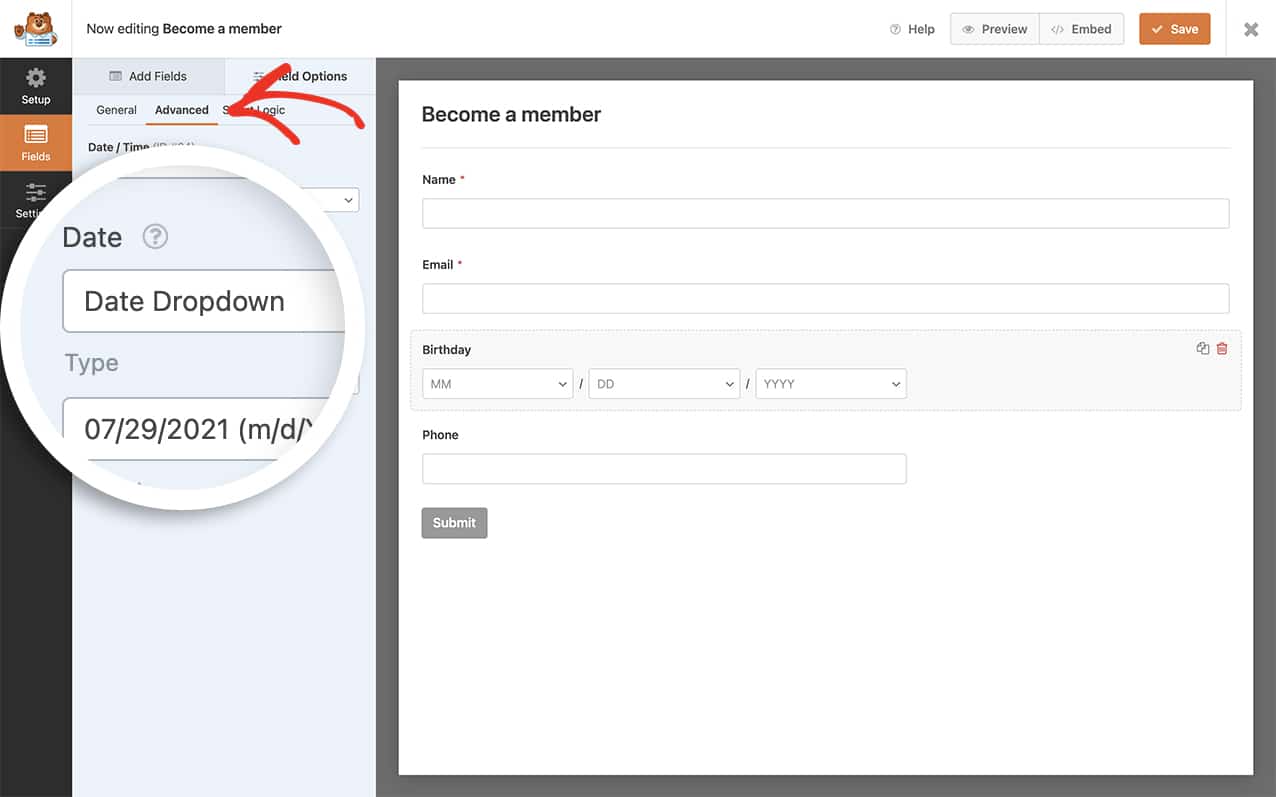
Customizing the Date field
In order to customize the Date field, you’ll need to add one of the code snippets below to your site.
If you need any help in learning how to add code snippets to your site, please review this tutorial.
Some of the code snippets below will require the use of specific form ID and field ID information.
Using snippets on the Date Dropdown
Limiting the number of days in the dropdown to match the month
In order to have the Days field coincide with the amount of days available for that particular month, you’d use this snippet.
/**
* Limit the days dropdown to match how many days are available for the selected month.
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_days_dropdown_match_month( ) {
?>
<script type="text/javascript">
var date = new Date();
var month = date.getMonth();
var year = date.getFullYear();
var totalDays = 31; // Default day amount
jQuery(function($){
$( 'select[id$="-month"]' ).on( 'change', function(){
month = $(this).val();
totalDays = daysInMonth(month, year);
$(this).parent().find( 'select[id$="-day"] option:not(:first-child)').each(function(){
$(this).remove();
});
for (var i = 1; i <= totalDays; i++) {
$(this).parent().find( 'select[id$="-day"]' ).append($( "<option></option>" ).attr( "value", i).text(i));
}
});
$( 'select[id$="-year"]' ).on( 'change', function(){
year = $(this).val();
totalDays = daysInMonth(month, year);
$(this).parent().find( 'select[id$="-day"] option:not(:first-child)').each(function(){
$(this).remove();
});
for (var i = 1; i <= totalDays; i++) {
$(this).parent().find('select[id$="-day"]').append($( "<option></option>" ).attr( "value", i).text(i));
}
});
});
function daysInMonth (month, year) {
return new Date(year, month, 0).getDate();
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_days_dropdown_match_month', 30 );
Limiting months, days and years inside the date dropdown
The snippets below will update the dropdown fields to only display 5-12 (May-December) for the available months, 20-31 for the available days, and 2020-2021 for the available years. You can modify the fields as needed.
There are two different ways this can be applied:
- All date dropdown fields inside a specific form
- A specific date dropdown inside a specific form.
All date dropdown fields inside a specific form
The snippet below will only apply to form ID 10.
/**
* Filters labels and options within the date field’s dropdown format.
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_datetime_date_dropdowns( $args, $form_id, $field ) {
$form_id = (int) $form_id;
// Only apply if the form's ID is 10
if ( $form_id === 10 ) {
// Set the lower and upper limits for each date dropdown
$args[ 'months' ] = range( 5, 12 );
$args[ 'days' ] = range( 20, 31 );
$args[ 'years' ] = range( 2024, 2025 );
}
return $args;
}
add_filter( 'wpforms_datetime_date_dropdowns', 'wpf_dev_datetime_date_dropdowns', 10, 3 );
Targeting a specific date dropdown inside a specific form
Below will apply to the date dropdown with field ID 3 inside form ID 10.
/**
* Filters labels and options within the date field’s dropdown format.
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_datetime_date_dropdowns( $args, $form_id, $field ) {
$form_id = (int) $form_id;
// Only apply if the form's ID is 10 and field ID 3
if ( $form_id === 10 && $field['id'] == 3) {
// Set the lower and upper limits for each date dropdown
$args[ 'months' ] = range( 5, 12 );
$args[ 'days' ] = range( 20, 31 );
$args[ 'years' ] = range( 2024, 2025 );
}
return $args;
}
add_filter( 'wpforms_datetime_date_dropdowns', 'wpf_dev_datetime_date_dropdowns', 10, 3 );
Starting the date dropdown from ‘today’
In your Date Dropdown, you can have the Month, Day and Year automatically default to start with today’s month, day and year using this snippet.
/**
* Select from today's date going forward within the range limit specified below
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_datetime_date_dropdowns( $args, $form_id, $field ) {
if( $form_id != 143 ) {
return $args;
}
// Set the lower and upper limits for each date dropdown
$args[ 'months' ] = range( date('m'), 12 );
$args[ 'days' ] = range( date('d'), 31 );
$args[ 'years' ] = range( date('Y'), 2030 );
return $args;
}
add_filter( 'wpforms_datetime_date_dropdowns', 'wpf_dev_datetime_date_dropdowns', 10, 3 );
Using snippets on the Date Picker
Disabling past dates and only displaying next 31 days
In this snippet, we’re going to disable any past date and only allow for selection up to 31 days from the current date for the form ID 1137
and the field ID 14
. Remember to change these IDs to match your own form and field ID. If you’re not sure where to find these IDs, please review this tutorial.
/**
* Disable past dates, allow for selection for today + the next 31 days
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_limit_date_picker() {
?>
<script type="text/javascript">
var d = new Date();
window.wpforms_1137_14 = window.wpforms_1137_14 || {};
window.wpforms_1137_14.datepicker = {
disableMobile: true,
// Don't allow users to pick dates less than 1 days out
minDate: d.setDate(d.getDate()),
maxDate: d.setDate(d.getDate() + 31),
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_limit_date_picker', 10 );
Show dates from the yesterday, today, and tomorrow
Using the new Date().fp_incr(-1) you can easily control how many days minDate and maxDate display by just increasing the number. Using the negative symbol (-) in front of the number means these will be days in the past.
Because we only want our form to start from yesterday, we’ll start with the minDate only allowing the (-1) and end with the (1) (so it will include today and tomorrow) for the maxDate
/**
* Allow date picker yesterday, today, and tomorrow
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
disableMobile: true,
// Don't allow users to pick past dates
minDate: new Date().fp_incr(-1), // 1 day in the past
// Don't allow users to pick any more than today
maxDate: new Date().fp_incr(1) // 1 day in the future
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Show dates from the next day and onwards for the date picker
You can easily use this code snippet that will exclude any past days (including today).
Simply change the +1), to be however many days out you wish to restrict the picker.
/**
* Show dates from the next day and onwards
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_limit_date_picker() {
?>
<script type="text/javascript">
var d = new Date();
window.wpforms_datepicker = {
disableMobile: true,
// Don't allow users to pick dates less than 1 days out
minDate: d.setDate(d.getDate() + 1),
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_limit_date_picker', 10 );
Block out specific date ranges for the date picker
To block out specific date ranges inside the date picker, use this snippet.
/**
* Limit the dates available in the Date Time date picker: Block out certain date ranges
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_limit_date_picker() {
?>
<script type="text/javascript">
var d = new Date();
window.wpforms_datepicker = {
disableMobile: true,
// Don't allow users to pick specific range of dates
disable: [
{
from: "2024-12-24",
to: "2024-12-26"
},
{
from: "2024-12-31",
to: "2025-01-01"
}
]
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_limit_date_picker', 10 );
Limit how far out users can pick the dates inside the date picker
If you want to limit the future date users can select inside the date picker, use this code snippet.
/**
* Don't allow date to be selected after maxDate
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
disableMobile: true,
// Don't allow users to pick past dates
minDate: new Date(),
// Don't allow users to pick dates after Dec 31, 2025
maxDate: new Date( '2025-12-31' )
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Exclude all past days and Mondays inside the date picker
To limit all past days and future Mondays, use this snippet.
/**
* Don't allow users to pick Mondays
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
disableMobile: true,
// Don't allow users to pick past dates
minDate: new Date(),
// Don't allow users to pick Mondays
enable: [
function(dateObj){
return dateObj.getDay() !== 1;
}
]
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Display message when current date is selected
For this example, we’re going to display a pop up message when the current date is selected.
/**
* Display pop up message when current date is selected
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_check_date() {
?>
<script type="text/javascript">
// Only display the message if the form ID is 323 and the field ID is 4
window.wpforms_323_4 = window.wpforms_323_4 || {};
window.wpforms_323_4.datepicker = {
mode: "single",
onClose: function(selectedDates, dateStr, instance) {
var today = new Date();
if (selectedDates[0].toLocaleDateString("en-US") == today.toLocaleDateString("en-US")) {
alert( 'Custom Message: You have selected todays date' );
this.clear();
}
}
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_check_date', 10 );
Disable a specific day of the week
For this example, we want to completely disable Sundays from our date picker using this snippet.
/**
* Disable a specific day of the week
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_limit_date_picker_disable_specific_weekday() {
?>
<script type="text/javascript">
var d = new Date();
// Only apply to the form ID 420 and the field ID 1
window.wpforms_420_1 = window.wpforms_420_1 || {};
window.wpforms_420_1.datepicker = {
disableMobile: true,
// Don't allow users to pick specific day of the week
disable: [
function(date) {
// return true to disable
// 1 = Monday; 2 = Tuesday; 3 = Wednesday; 4 = Thursday; 5 = Friday; 6 = Saturday; 0 = Sunday
return (date.getDay() === 0);
}
]
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_limit_date_picker_disable_specific_weekday', 10 );
In the snippet, the return (date.getDay() === 0); disables only Sundays. Each day is represented by a specific number.
- 1 = Monday
- 2 = Tuesday
- 3 = Wednesday
- 4 = Thursday
- 5 = Friday
- 6 = Saturday
- 7 = Sunday
Just find the numerical representation for your specific day and update the snippet to match your desired day.
The window.wpforms_420_1 represents the form ID (420) and the field ID for our Date Picker (_1). You’ll need to update these two IDs to match your own form ID and field ID.
For assistance in finding your form and field ID numbers, please see this tutorial.
Change the calendar’s start day
To change the date picker day to show that starts the week, you’ll use this snippet. Keep in mind that Monday represents the number one and the days of the week continue on in numeric order.
/**
* Change the start day of the week
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_set_start_day() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
disableMobile: true,
"locale": {
// Enter the number for the day you want the week to start
// 1 = Monday; 2 = Tuesday; 3 = Wednesday; 4 = Thursday; 5 = Friday; 6 = Saturday; 7 = Sunday
"firstDayOfWeek": 1 // start week on Monday
}
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_set_start_day', 10 );
Disabling future dates
Using this snippet will set the maxDate as today’s date (current) so user’s wouldn’t be able to select future dates.
/**
* Disable future dates
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
disableMobile: true,
// Don't allow users to pick past dates
maxDate: new Date(),
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Target specific form ID and field ID inside the date picker
When using the Date Picker, you can target a single form or a specific Date field inside a specific form.
In the example snippets below, you’ll see window.wpforms_21_1. Where as the _21 represents the form ID and the _1 represents the field ID.
If you need help in finding your form and field ID numbers, please see this tutorial.
Here are a couple of code snippets. The first one will allow for a selection of only the past 30 days:
/**
* Only allow for a selection of only the past 30 days
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_21_1 = window.wpforms_21_1 || {};
window.wpforms_21_1.datepicker = {
disableMobile: true,
// Don't allow users to pick more than 30 days in the past and exclude future dates
minDate: new Date().fp_incr(-30)
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
This next code snippet will allow for a selection of the past 30 days and “today”:
/**
* Only allow for a selection of the past 30 days and "today"
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_21_1 = window.wpforms_21_1 || {};
window.wpforms_21_1.datepicker = {
disableMobile: true,
// Don't allow users to pick more than 30 days in the past
minDate: new Date().fp_incr(-30),
maxDate: "today"
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Limit to a single day for the current month only
In this example, we’re only going to accept appointments for a single day in the current month.
/**
* Limit to 1 single day for the current month only.
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_one_day_current_month( ) {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
disableMobile: true,
disable: [
function(date) {
var currentDate = new Date();
var currentMonth = currentDate.getMonth();
var currentYear = currentDate.getFullYear();
var selectedMonth = date.getMonth();
var selectedYear = date.getFullYear();
if (selectedMonth !== currentMonth || selectedYear !== currentYear) {
return false;
}
var day = date.getDay();
var firstDayOfMonth = new Date(selectedYear, selectedMonth, 1).getDay();
var offset = (day >= firstDayOfMonth) ? (day - firstDayOfMonth) : (day + 7 - firstDayOfMonth);
var secondThursday = new Date(selectedYear, selectedMonth, offset + 8);
if (date.getDate() === secondThursday.getDate() && day === 4) {
return false;
}
return true;
}
]
};
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_one_day_current_month', 30 );
In this example, we’re dynamically setting a minimum date for a second date selection field, ensuring it is at least 21 days after the first selected date.
/**
* Add 21 days to the first date selection to block these days from the second selection
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options/
*/
function wpf_dev_block_days_date_selection( ) {
?>
<script type="text/javascript">
jQuery(document).ready(function($) {
$( "#wpforms-1277-field_19" ).flatpickr({
dateFormat: "m/d/Y",
onClose: function( selectedDates, dateStr, instance ) {
if ( selectedDates.length > 0 ) {
var minSecondDate = new Date( selectedDates[0] );
// Adding 21 days to the selected 1st date
minSecondDate.setDate( minSecondDate.getDate() + 21 );
$( "#wpforms-1277-field_20" ).flatpickr({
minDate: minSecondDate,
dateFormat: "m/d/Y"
});
}
}
});
});
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_block_days_date_selection', 30 );
Translating Date and Time Sublabels
To translate the “Date” and “Time” sublabels in the Date/Time field, you can use this code snippet:
``/**
* Translate Date and Time sublabels in the Date/Time field
*
* @link https://wpforms.com/developers/customize-the-date-time-field-date-options
*/
function custom_translate_date_time_sublabels( $properties, $field, $form_data ) {
// Check if the field is of type 'date-time'
if ( 'date-time' === $field['type'] ) {
// Change the sublabel for the date field
if ( isset( $properties['inputs']['date']['sublabel']['value'] ) ) {
$properties['inputs']['date']['sublabel']['value'] = __( 'Your Translated Date Label');
}
// Change the sublabel for the time field
if ( isset( $properties['inputs']['time']['sublabel']['value'] ) ) {
$properties['inputs']['time']['sublabel']['value'] = __( 'Your Translated Time Label');
}
}
return $properties;
}
add_filter( 'wpforms_field_properties', 'custom_translate_date_time_sublabels', 10, 3 );
To use this snippet:
- Replace ‘Your Translated Date Label’ with your desired translation for “Date”
- Replace ‘Your Translated Time Label’ with your desired translation for “Time”
For example, to translate the labels to Spanish:
$properties['inputs']['date']['sublabel']['value'] = __( 'Fecha');
$properties['inputs']['time']['sublabel']['value'] = __( 'Tiempo');
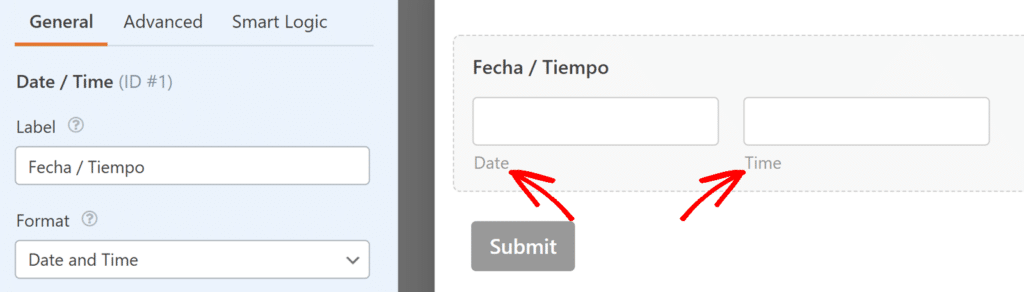
One final reminder, please remember that if you are using any of the code snippets in this doc, the form builder options for limiting should be turned off. To find out more about the built-in options for limiting the dates, please check out this documentation.
And that’s all you need to customize the Date Dropdown or Date Picker! Would you like to customize the date picker to allow for a range of dates or multiple dates? Try out our tutorial on How to Allow Date Range or Multiple Dates in Date Picker.