Would you like to customize the Date Picker field to allow for a date range or multiple dates to be selected inside your form? When you allow for multiple bookings in one form, you may need to give your visitors the ability to select a range of dates or multiple dates. By default, only a single date can be selected within this calendar.
In this tutorial, we’ll show you how to use PHP to provide a date range or multiple date selections for your WPForms.
Note: When using snippets for the Date Picker, it’s best turn off any of the built-in features WPForms provides in the form builder for further restricting dates. For more information, please see this documentation.
Creating the form
First you’ll need to create your form and add at least one Date/Time form field and set the field to Date Picker.
If you need any assistance in creating a form, please review this documentation.
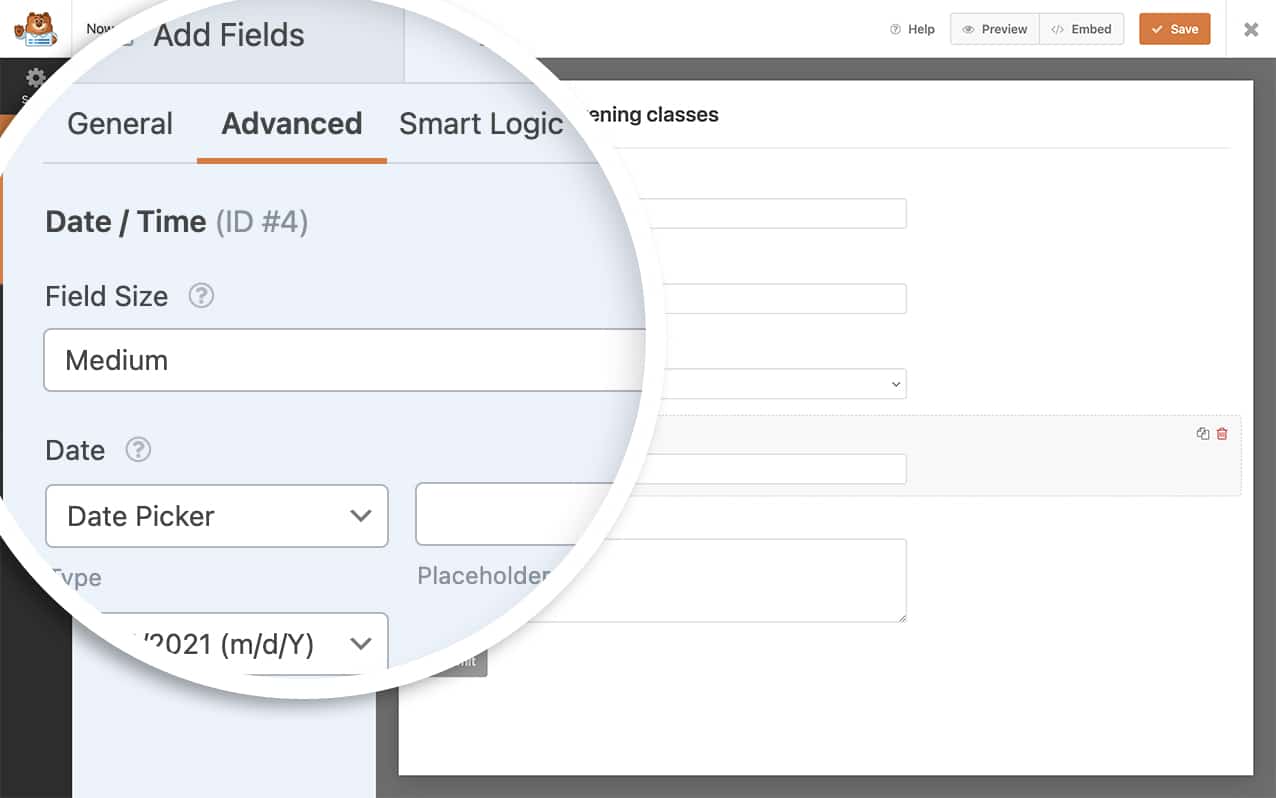
Allowing for a date range or multiple dates
Once you’re form is created, choose if you’d like to allow for a date range or to allow for multiple dates to be selected in your form and add that snippet to your site.
If you need any help in how to add snippets to your site, please see this tutorial.
Note: Please note that if you edit these entries, the entry screen doesn’t support date range or multiple dates. This snippet is only for the frontend users of your forms.
Date Range
This particular snippet will allow for all date pickers to select a range of dates for all forms.
/**
* Modify WPForms Date/Time field date picker to accept a range of dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_date_picker_range() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
mode: "range"
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_date_picker_range', 10 );
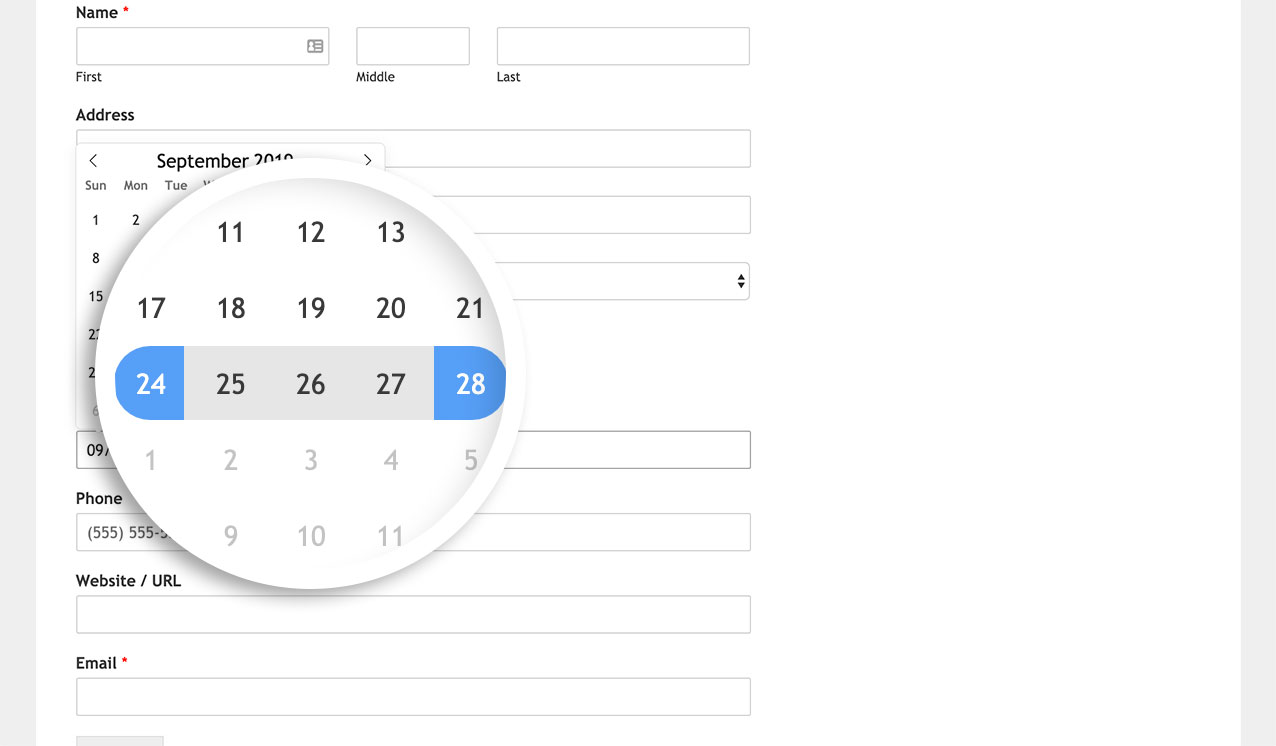
Within the submission entry, the start and end dates will be noted.
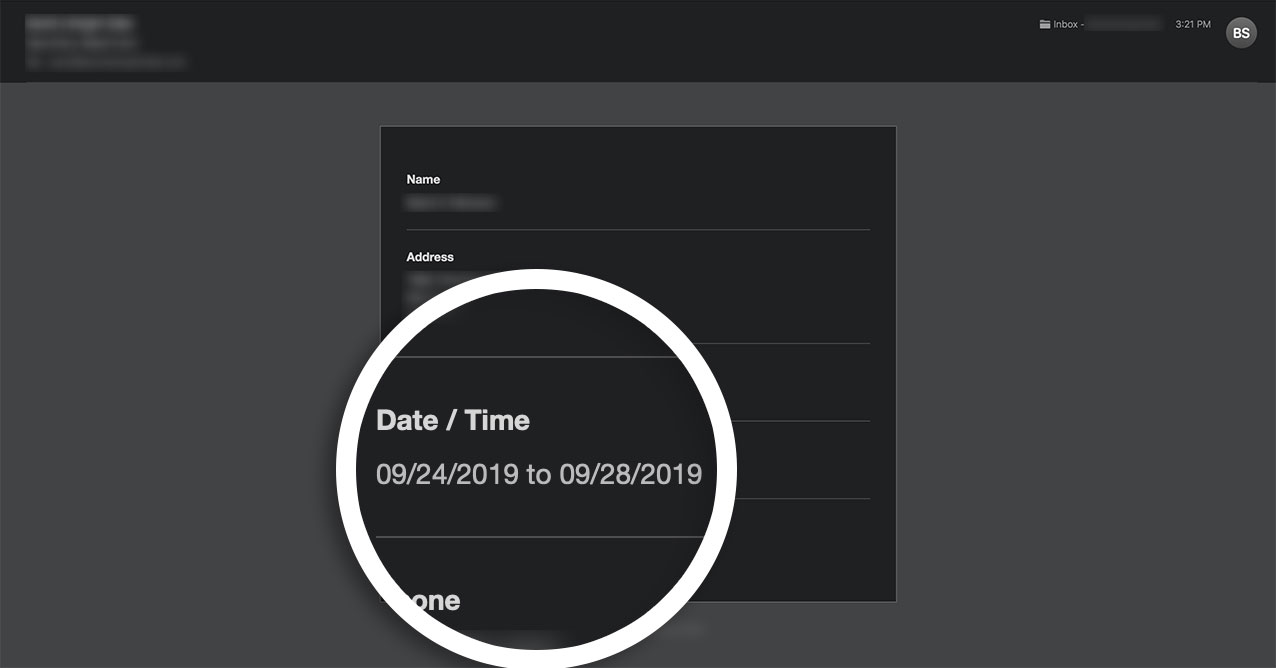
Multiple Dates
Alternatively, you can use this snippet that would allow for multiple dates to be selected for every date picker on your forms created with WPForms.
/**
* Modify WPForms Date/Time field date picker to accept multiple dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_date_picker_multiple() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
mode: "multiple"
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_date_picker_multiple', 10 );
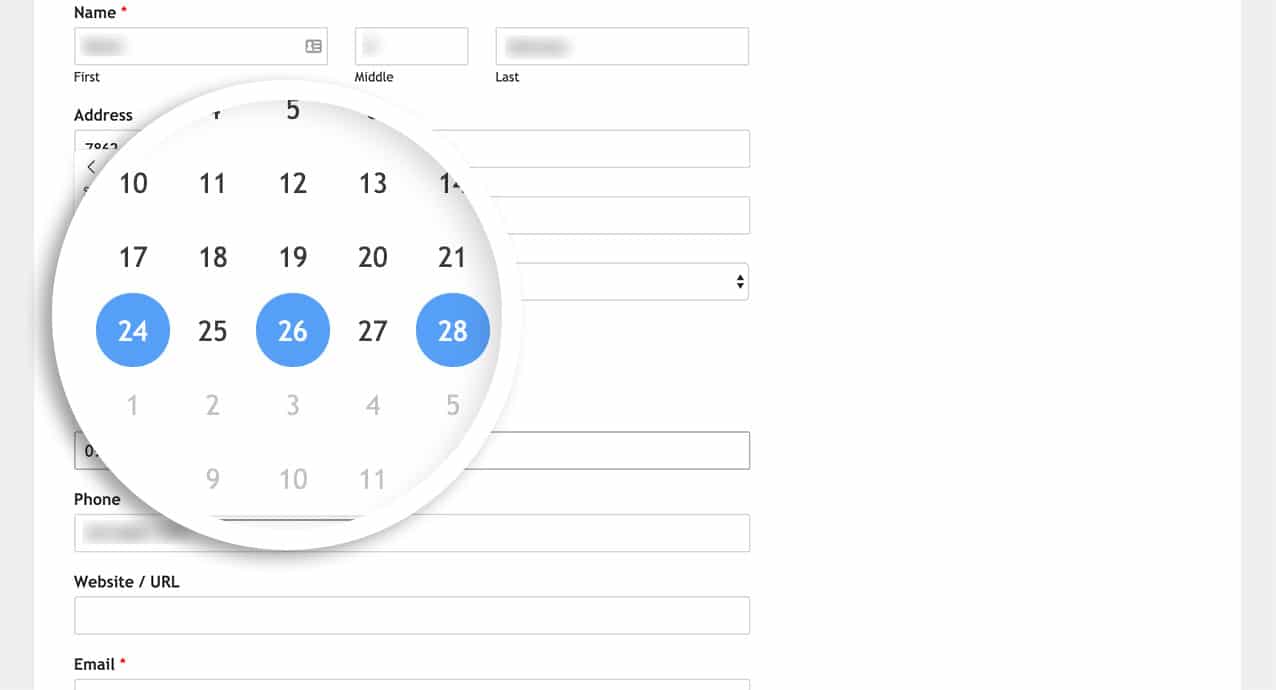
Within the entry, any selected dates will be separated by a comma.
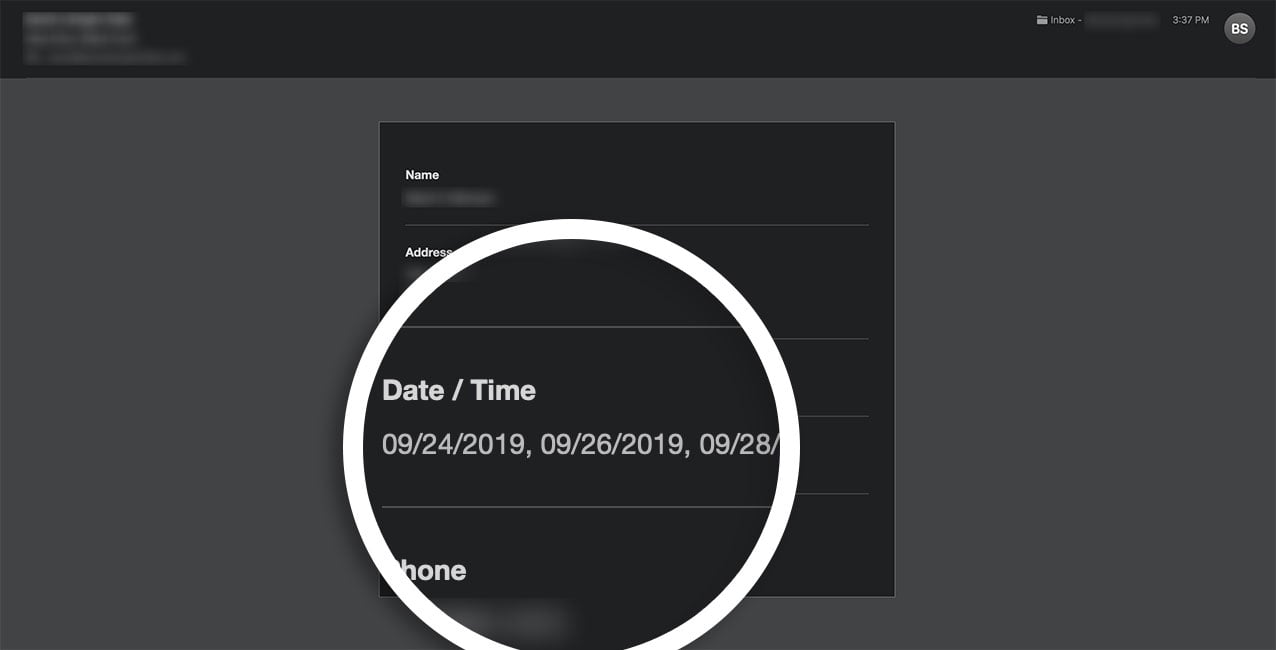
Note: If using third-party integrations, these date formats may not be accepted by those services. Be sure to test any customizations before using on a live form.
And that’s it! You’re now able to select a range of dates or multiple dates on your WPForms. Would you like to create more options for your Date field? Try out the article on How to Create Additional Formats for the Date Field.
Reference Action
FAQ
Q: How do I target a single form or a single date picker inside a specific form?
A: To target a specific form for the date range as an example, you would use this snippet.
/**
* Modify WPForms Date/Time field date picker to accept a range of dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_1049 = window.wpforms_1049 || {};
window.wpforms_1049.datepicker = {
mode: "range",
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Where as the 1049 inside the window.wpforms_1049 is the form ID.
If you have multiple date pickers inside a specific form, you could target a specific field ID with this example.
/**
* Modify WPForms Date/Time field date picker to accept a range of dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_1049_11 = window.wpforms_1049_11 || {};
window.wpforms_1049_11.datepicker = {
mode: "range",
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
Just as with the first example, we’re targeting the form ID 1049, however with this example we’re also only targeting the date picker with the field ID of 11.
If you need help in finding your form and field ID, please see this tutorial.
Q: Can the form use the date range and still limit the dates?
A: Absolutely! You can use the date range, not allow past dates, and only allow a number of days to be picked in advance. In the code snippet below, we’re targeting form ID 1049 and the form’s date picker will:
- Not allow past dates to be selected
- Use the date range functionality
- Allow only up to 14 days to be selected
/**
* Modify WPForms Date/Time field date picker to accept a range of dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
window.wpforms_1049 = window.wpforms_1049 || {};
window.wpforms_1049.datepicker = {
minDate: new Date(),
mode: "range",
maxDate: new Date().fp_incr(14)
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10 );
If you need help in finding your form and field ID, please see this tutorial.
Q: Can I change the separator for the date range?
A: Absolutely! To use a different date separator on the date range, use this code snippet instead of the above.
/**
* Modify WPForms Date/Time field date picker to accept a range of dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_date_picker_range() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
mode: "range",
locale: {
rangeSeparator: 'sep-text-goes-here'
}
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_date_picker_range', 10 );
Just remember to change rangeSeparator: ‘sep-text-goes-here’ to match what you want the separator to be. By default, you will see a dash (-) as a separator.
Q: Can I require a minimum range of days to be selected?
A: Absolutely, to use the range and require a minimum number of days, just use this code snippet.
/**
* Modify WPForms Date/Time field date picker to accept a range of dates.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_date_picker_range() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
mode: "range",
onClose: function(selectedDates, dateStr, instance) {
var daysInRange = document.getElementsByClassName('inRange');
var totalDays = daysInRange.length+2;
if(totalDays<=7) {
alert("Min 7 Days Required");
this.clear();
}
}
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_date_picker_range', 10 );
Q: Can I target date pickers using CSS classes instead of window objects?
A: Yes, you can target date pickers using CSS classes instead of window objects. This approach allows for more flexibility in targeting specific elements without extensive customization.
Here’s an example using jQuery class selectors:
/**
* Modify WPForms Date/Time field date picker to accept a range of dates using jQuery.
*
* @link https://wpforms.com/developers/allow-date-range-or-multiple-dates-in-date-picker/
*/
function wpf_dev_limit_date_picker() {
?>
<script type="text/javascript">
jQuery(document).ready(function($) {
if (typeof flatpickr !== 'undefined') {
$('.travel-form-date-range').each(function() {
var inputField = $(this).find('input.flatpickr-input');
if (inputField.length) {
flatpickr(inputField[0], {
mode: "range",
disableMobile: true
});
}
});
}
});
</script>
<?php
}
add_action('wpforms_wp_footer_end', 'wpf_dev_limit_date_picker', 10);
In this example, we’re targeting date pickers with the CSS class travel-form-date-range
. This method allows you to apply the date range functionality to specific date pickers based on their class, rather than using form or field IDs.
Remember to replace travel-form-date-range
with the actual CSS class you’re using for your date picker fields.
Can I show multiple months at once in the date picker calendar?
Yes! You can display more than one month at a time using the showMonths
option from the Flatpickr library. This is helpful if you want users to see more dates at a glance when selecting a range.
function wpf_dev_date_picker_range() {
?>
<script type="text/javascript">
window.wpforms_datepicker = {
mode: "range",
showMonths: 2
}
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_date_picker_range', 10 );
In the example above, the calendar will show 2 months side by side when selecting dates. You can change showMonths
to any number depending on how many months you want to display.