Would you like to process Smart Tags in the Checkbox field options? By default, Smart Tags will not be processed if placed within the individual choices of the Checkbox field. However, using a small PHP code snippet you can easily allow this.
In this tutorial, we’ll walk you through how to process Smart Tags in Checkbox field options.
Smart Tags are a great way to pull and display data dynamically within your form. WPForms also lets you create custom Smart Tags that can be used to extend this functionality even further.
Creating the Form
For this tutorial, we will add some fields to our membership form that will confirm the full name or display name of the person completing the form. This will be pulled from the WordPress profile as well as a form field for How did you hear about this offer? which will also be pulled from a Smart tag.
If you need help with creating a form, please check out this helpful guide.
Using Smart Tags in Checkbox Field Choices
We can use the Smart Tags, so we’ll enter {user_full_name}
in the label for the first option and {user_display}
for the second option.
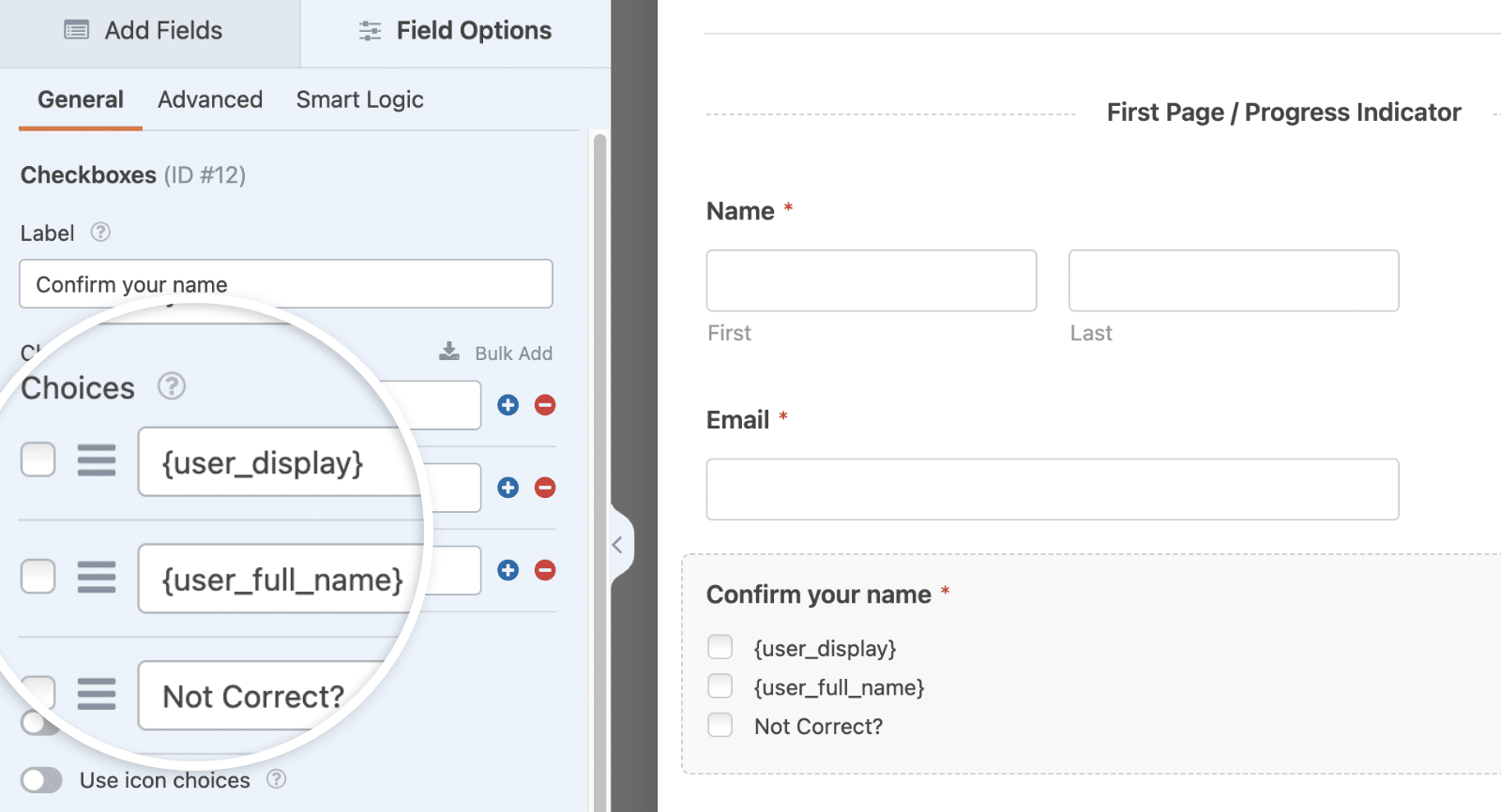
We will also add a third option that, when selected, will conditionally show a Single Text Line form field for the visitor to enter the correct name if the profile records are incorrect.
Next, we will add another Checkbox field to ask the visitor to complete the How did you hear about this offer? which will include the {url_referer}
Smart Tag to try and pull the URL the visitor was just referred from.
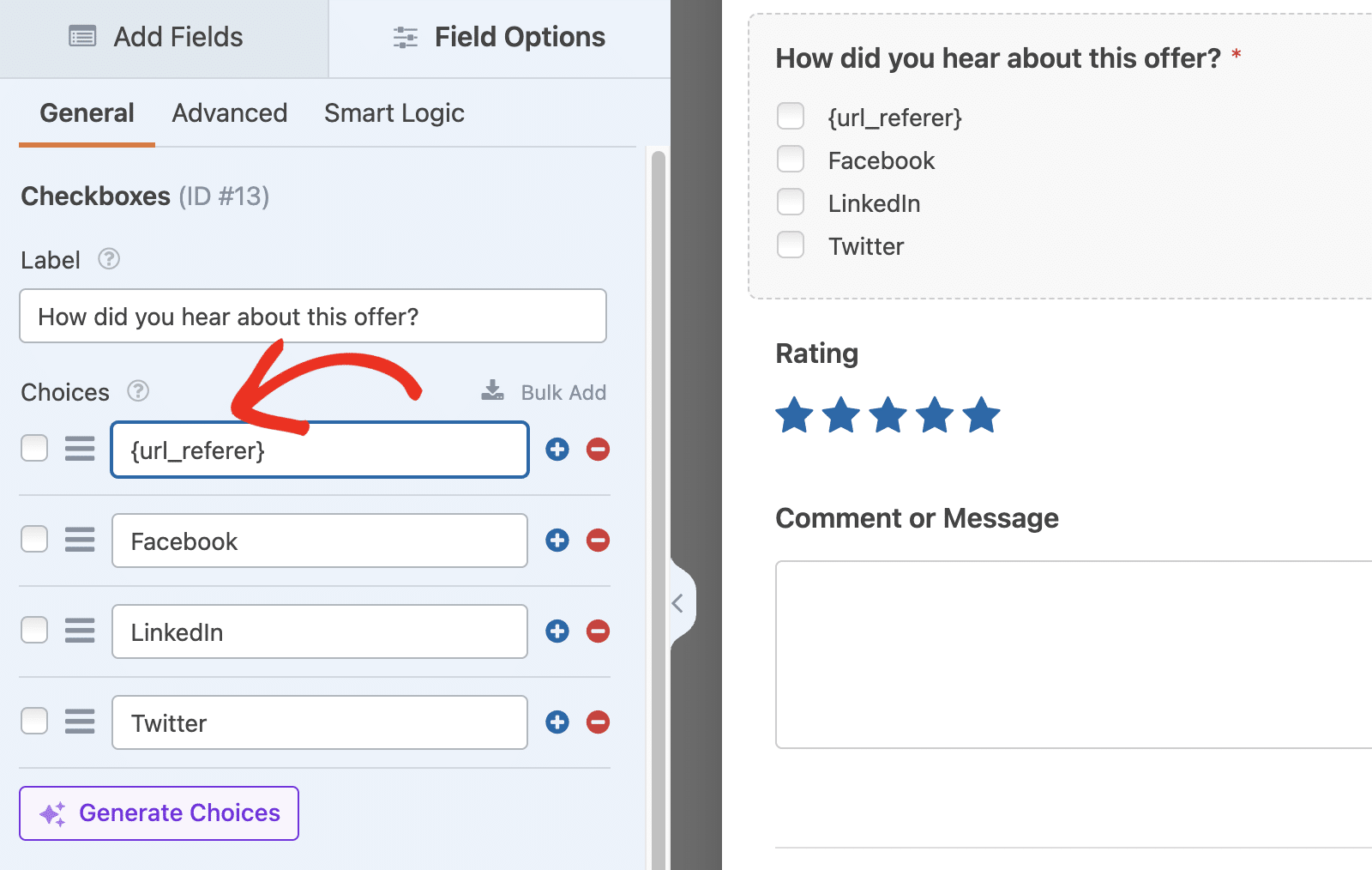
Adding the Code Snippet
To process any Smart Tags included for the Checkbox field choices, you’ll need to copy this code snippet to your site.
If you need help adding code snippets, please review this tutorial.
Note: This snippet won’t pull user input values (for example, {field_id="3"}
) to populate other form fields on the form.
/**
* Using Smart Tags in Checkboxes.
*
* @link https://wpforms.com/developers/process-smart-tags-in-checkbox-labels/
*/
function wpf_dev_checkbox_choices_process_smarttags( $field, $deprecated, $form_data ) {
foreach ( $field[ 'choices' ] as $key => $choice ) {
if ( ! empty( $choice[ 'label' ] ) ) {
$field[ 'choices' ][ $key ][ 'label' ] = apply_filters( 'wpforms_process_smart_tags', $choice[ 'label' ], $form_data );
}
}
return $field;
}
add_filter( 'wpforms_checkbox_field_display', 'wpf_dev_checkbox_choices_process_smarttags', 10, 3 );
The code above tells the checkbox options to process the filter for Smart Tags with apply_filters( ‘wpforms_process_smart_tags’,. This is what allows you to use Smart Tags as labels in the Checkboxes field.
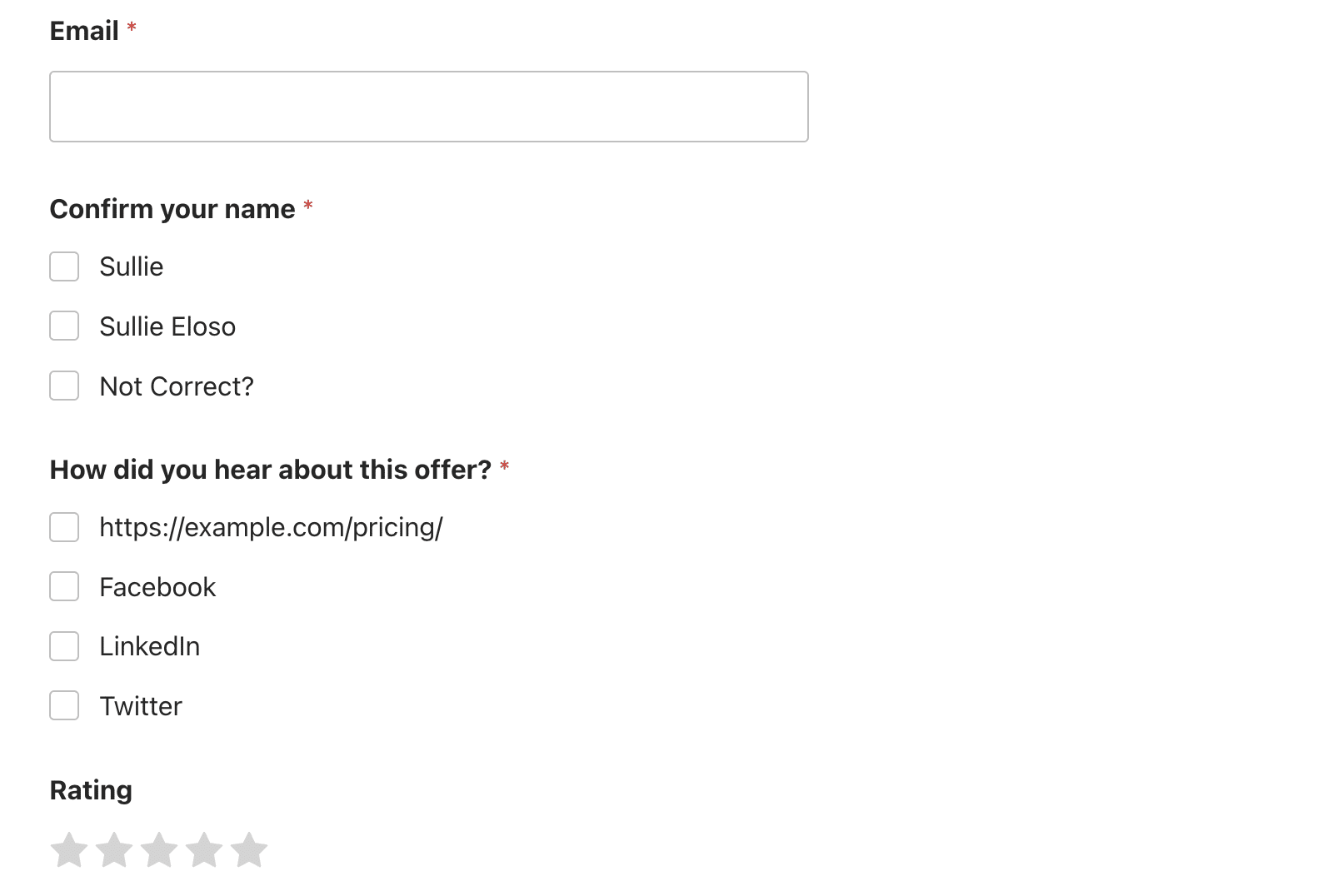
Frequently Asked Questions
These are answers to some of the top questions we see about making Smart Tags usable on Checkbox field labels.
Q: Can I use this for Dropdown and Multiple Choice fields too?
A: Absolutely! Use this snippet to process Smart Tags inside the field labels of the Dropdown and Multiple Choice form fields.
/**
* Using Smart Tags in Multiple Choice and Dropdown choices.
*
* @link https://wpforms.com/developers/process-smart-tags-in-checkbox-labels/
*/
function wpf_dev_select_radio_choices_process_smarttags( $field, $deprecated, $form_data ) {
foreach ( $field[ 'choices' ] as $key => $choice ) {
if ( ! empty( $choice[ 'label' ] ) ) {
$label = apply_filters( 'wpforms_process_smart_tags', $choice[ 'label' ], $form_data );
if ( ! empty( $label ) ) {
$field['choices'][ $key ][ 'label' ] = $label;
} else {
// Remove empty option.
unset( $field['choices'][ $key ] );
}
}
}
return $field;
}
add_filter( 'wpforms_radio_field_display', 'wpf_dev_select_radio_choices_process_smarttags', 10, 3 );
add_filter( 'wpforms_select_field_display', 'wpf_dev_select_radio_choices_process_smarttags', 10, 3 );
That’s it! You now know how to use Smart Tags in the Checkbox field labels.
Next, would you like to also use Smart Tags in the HTML / Code form field? Take a look at our tutorial on processing Smart Tags in HTML fields.
Related
Filter References: