Would you like to skip page breaks in your form when the conditional logic isn’t met? When using conditional logic with page breaks, the next page is shown even if the conditions aren’t satisfied, leaving users with an empty page. With a simple JavaScript snippet, you can easily skip those unnecessary pages.
In this tutorial, we’ll show you how to skip empty pages with a simple JavaScript snippet.
Creating the Form
First, you’ll need to create a new form or edit an existing one to access the form builder. We’ve created a quote form that has multiple page breaks.
On the first page, we’re gathering some basic information about users. We’ve also added a Multiple Choice field to ask them if they would like to schedule a free in-person quote at the time.
If they select Yes, we’ll display the second page and ask them for some personal information. However, if they select No, we want to skip the second page entirely and jump straight to the final page of the form.
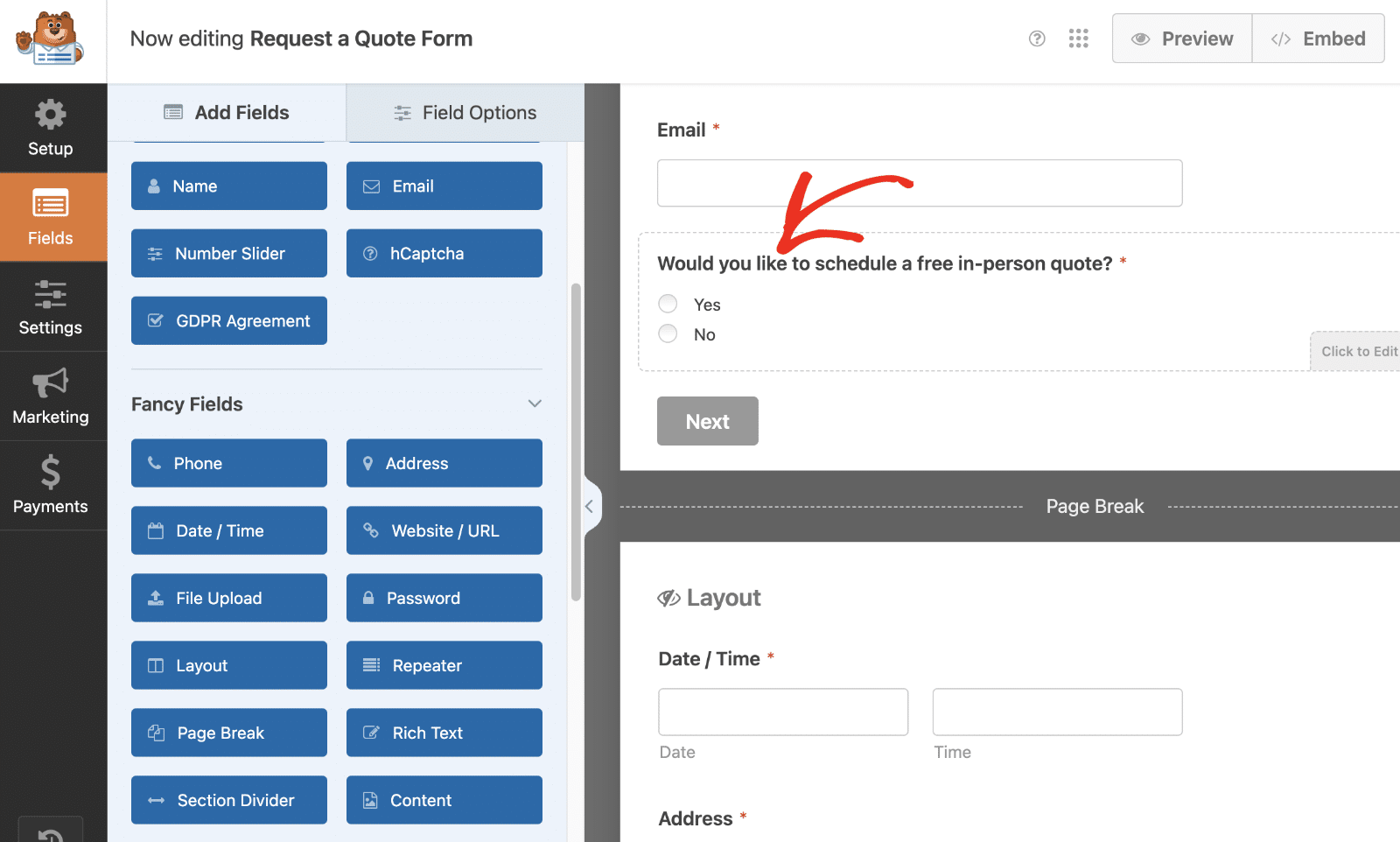
Setting Up Conditional Logic
For our example, we only want to show the Date / Time and Address fields on the second page if they have selected on the first page that they are interested in receiving a free in-person quote. This will save our visitors time if they are not interested, so we can skip this page entirely if they don’t wish to visit.
The two fields on the second page will all have the same logic set. To set up the logic, select each field and click Smart Logic. Next, toggle the switch to Enable Conditional Logic.
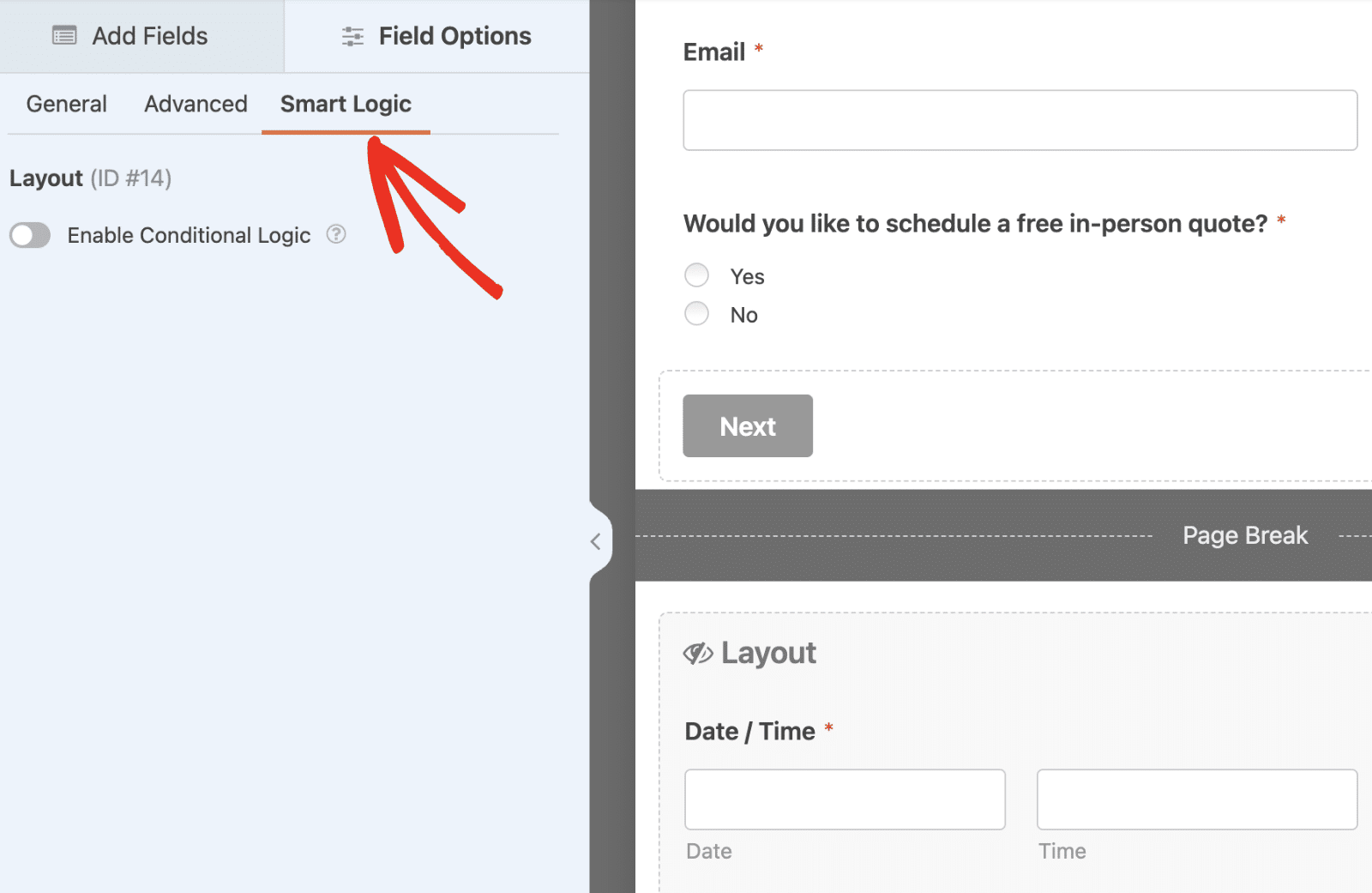
From the dropdown, select the question you based your fields on. For this tutorial, our question was a Multiple Choice field that asks Would you like to schedule a free in-person quote?.
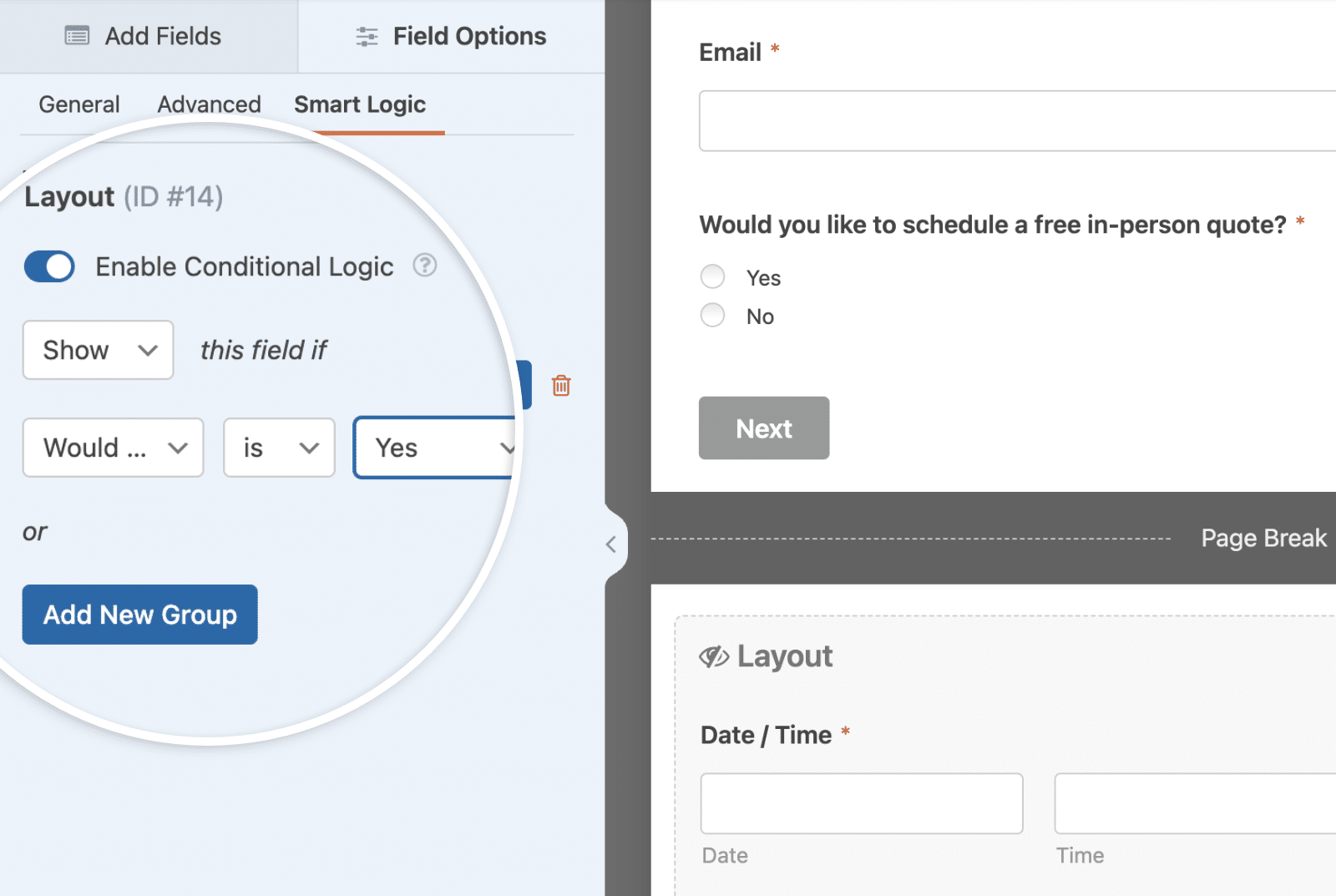
Our condition is that if the answer to the Multiple Choice field is Yes, then show the field. If it’s No, hide the field.
Each field on the second page will have the same logic, so you’ll repeat this step for each field. Alternatively, you can use the Layout field to group similar fields and then enable conditional logic for the Layout field.
Adding the Snippet
Now, you’ll need to add the snippet below to your site. If you need any help in how and where to add snippets to your site, please review this tutorial.
This snippet will loop through each page of your form to determine if any is empty. If there’s an empty page, the script will skip that page completely and move on to the next. This will improve the overall experience of your form.
Frequently Asked Questions
Below, we’ve answered some of the top questions about using conditional logic on multi-page forms.
Q: Will this snippet work in a modal window like an Elementor popup?
A: Not at this time.
That’s it! You’ve now learned how to skip pages if they are hidden on your form due to conditional logic.
Would you like to conditionally show or hide the Submit button on a form based on one of your form fields? Check out our tutorial on How to Conditionally Show the Submit Button.