Would you like to use your WPForms Smart Tags in your WordPress rewrite rules? You may have a custom post type that you’d like to pass through an email address captured from your WPForms submissions to display in your rewrite rules and in this tutorial, we’ll show you how to use a small PHP snippet you can easily achieve this.
A URL rewrite rule will take a standard URL and modify its appearance. It won’t change the location of the page, it just changes how the URL appears in your visitor’s browser window.
Understanding URL Rewrite Rules
URL rewrite rules modify how URLs appear in the browser without changing the actual page location. They help create more user-friendly and SEO-friendly URLs. For detailed information about rewrite rules, check out WordPress.org’s documentation on URL rewriting.
Example Scenario
In this guide, we’ll create a photography portfolio system where photographers submit images through a WPForms upload form. Each photographer gets a unique portfolio page, and the URL structure uses their email address for identification.
Setting Up the Rewrite Rule
First, we’ll create our custom rewrite rule:
add_rewrite_rule(
'portfolio/vendor/1/([a-zA-Z0-9]+)/?$',
'index.php?pagename=portfolio-vendor&email_address=$matches[1]',
'top' );
Creating Your Form
Now it’s time to set up our form. Since, in this tutorial, we’re accepting uploads, we’re going to create a new post submission form using WPForms Post Submissions addon.
We need to pass the Email Smart Tag inside a query string to build our rewrite rule. To do this, click on the Advanced tab of the Email form field and inside the Default Value, add the Smart Tag {query_var key="email-address"}
.
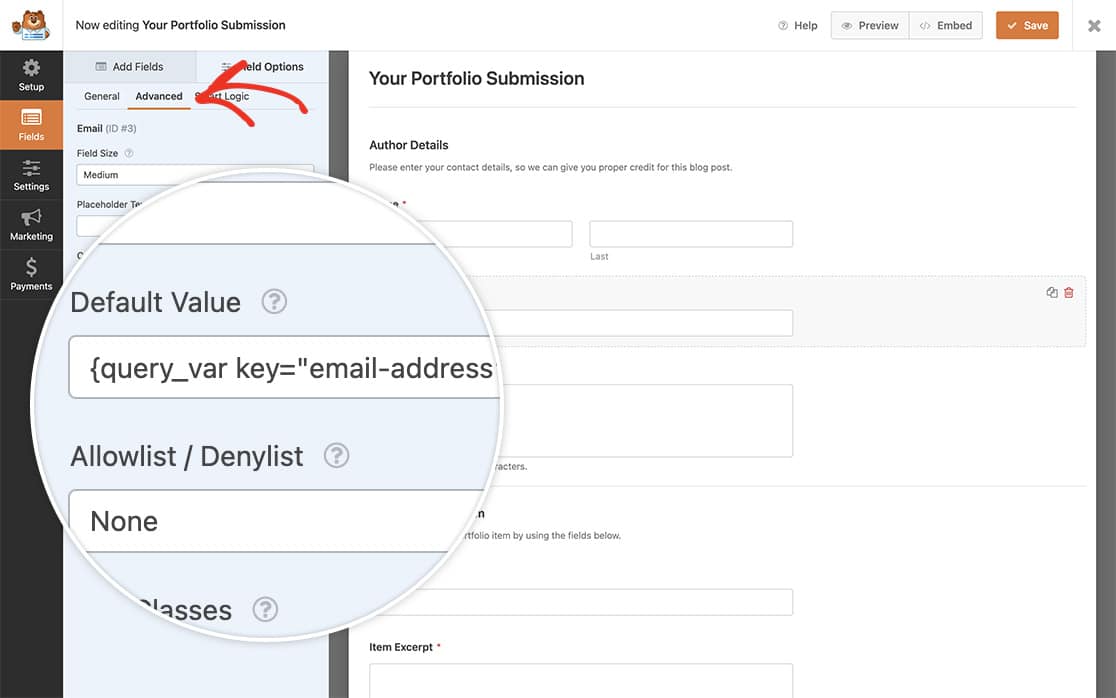
Adding the Smart Tag Processing Code
Now it’s time to add the code snippet to your site.
If you’re not sure how to add custom code, please see our guide on how to add code snippets.
Now instead of your URL showing in the browser like this https://example-site.com/?pagename=portfolio-vendor&[email protected]
it will now appear as https://example-site.com/portfolio/vendor/1/[email protected]/
The code registers a custom query variable for the email address, processes Smart Tags to work with the rewrite rules, and maintains clean URLs while preserving functionality.
And that’s it! You are now able to use a Smart Tag inside your WordPress rewrite rules. Would you like to create a custom Smart Tag? Take a look at our tutorial on creating custom smart tags for more details.