Would you like to localize the Date Picker strings within WPForms Date / Time field? The default Date Picker language is English. However, with a third-party JavaScript library, you can translate the Date Picker strings into other supported languages.
In this tutorial, we’ll guide you through the fundamentals of localizing Date Picker strings using JavaScript.
Understanding How WPForms Date Picker Works
WPForms utilizes the Flatpickr date script to generate its Date Picker. Flatpickr constructs the date picker using JavaScript. With over 25 languages available, Flatpickr offers extensive language support, making it easy to localize Date Picker strings.
Localizing the Date Picker Strings
Localization allows you to adapt your WordPress site and its plugins to different languages, ensuring a seamless experience for users worldwide. Below, we’ll cover the steps to translate Date Picker strings in WPForms.
1. Finding the URL and Language Code
To find the URL for the language you’ll need for this snippet, navigate to the Flatpickr GitHub Repository and locate the language file corresponding to your preferred language, such as es
for Spanish or fr
for French. Once you’ve found the appropriate language file, construct a URL using the following format:
https://npmcdn.com/[email protected]/dist/l10n/{language_code}.js
Replace {language_code}
with the code of your chosen language. For example, if you’re localizing into French, your URL would be:
https://npmcdn.com/[email protected]/dist/l10n/fr.js
This URL will provide the necessary JavaScript file containing the localized Date Picker strings for your chosen language.
Note: If your language code isn’t listed in Flatpickr’s GitHub Repository, then your language isn’t currently supported.
2. Loading the Date Picker Locale
After getting the URL to localize the wpforms-datepicker-locale
, the next step is to add the code to your site. For this, you’ll need to add some custom JavaScript snippets to your site.
Note: If you need any assistance on how and where to add custom snippets, see our tutorial on adding custom snippets in WordPress for a detailed walkthrough.
For this tutorial, we’ll use French, so in the snippet below, you’ll notice that we’re enqueuing the script https://npmcdn.com/[email protected]/dist/l10n/fr.js
. You’ll need to update the script to match the language you want to localize.
The snippet above checks if there is any Date / Time field in the forms on your site, and then modifies the default WPForms Date Picker locale to match the one you specified.
3. Applying the Localization
The second step is to apply the localization of the Date Pickers to the page, which is done via JavaScript. Proceed to add the code snippets below to your site.
When users open the date picker in your form, the strings will be translated into the language you specified.
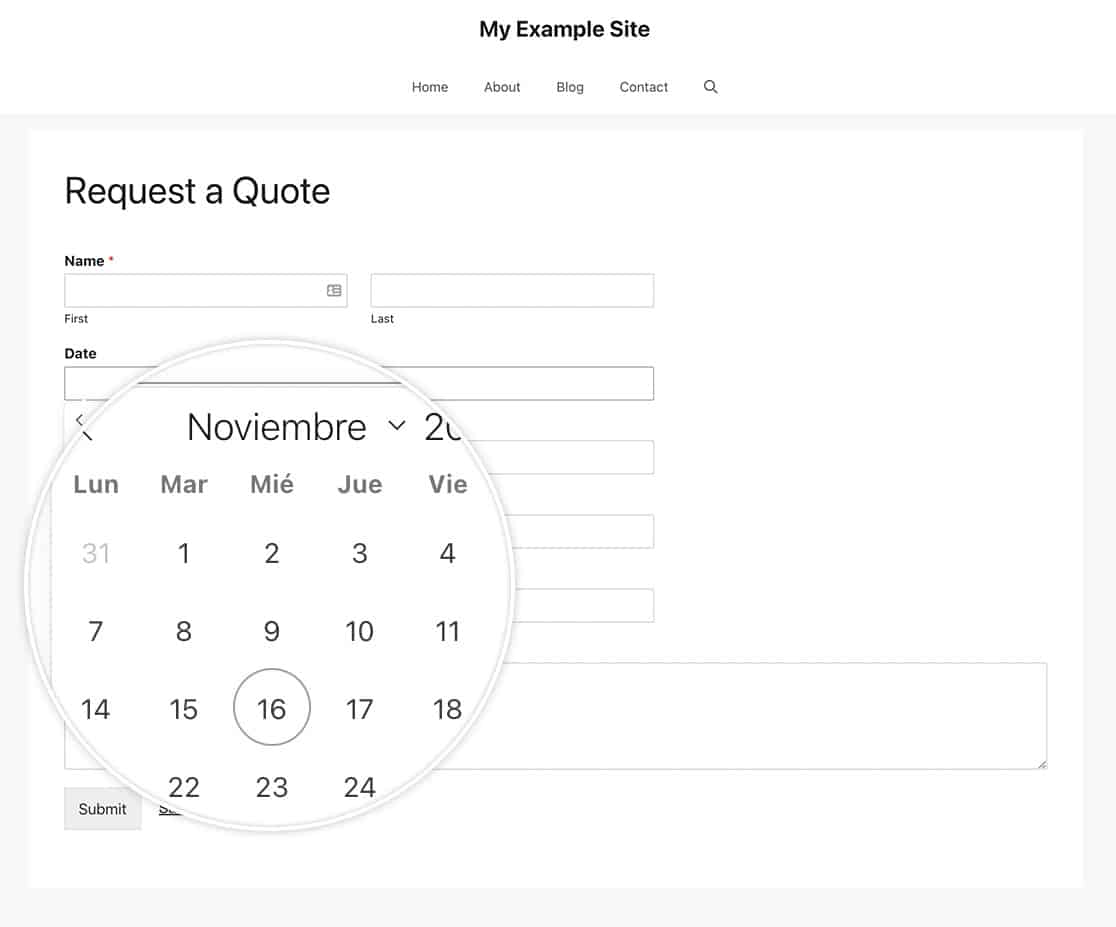
Adding Conditional Logic (optional)
If you are using the WPML plugin on your site, you’ll be able to conditionally load the date picker localization module. This way the date picker strings only change if the user is viewing that specific locale. The example below will only apply the localization if the user has specified that they want to view the Spanish version of the page (es).
Frequently Asked Questions
Below, we’ve answered some common questions about translating Date Picker strings in WPForms.
Q: When I select the date, why doesn’t it show the correct format?
A: When using this snippet, you only localize the Month and Days in the date picker. If you wish to change the format of how the date appears, please check out this tutorial instead.
That’s it! You’ve successfully localized the text for the Date Picker.
Would you like to customize the Date / Time form field? Have a look at our tutorial on How to Customize the Date Time Field Date Options.