Would you like to prevent users from entering numbers in your Single Line Text fields? While you can use input masks to restrict fields to alpha characters only, this guide will show you how to create a more flexible solution that allows both uppercase and lowercase letters while blocking numbers.
In this tutorial, we’ll show you the necessary code snippet to restrict numbers in these fields.
Setting Up the Form
First, create a new form and add a Single Line Text field that you want to restrict. For our example, we’ll use it as a Username field. If you need help creating a form, check out our guide on creating your first form.
Make note of your form ID and field ID as you’ll need these for the code. If you’re not sure how to find these IDs, please review our guide on how to find form and field IDs.
Adding the Validation Code
This code will prevent form submission if numbers are detected in the field. If you need help adding code to your site, please check out our guide on adding custom code to WordPress site.
Replace the following values in the PHP code:
- On line 11: Replace 1000 with your form ID
- On line 17: Replace 25 with your field ID
- On line 22: Replace 25 with your field ID again
If the Username field contains any numbers at all when the form is submitted, an error message will appear and the form will not submit.
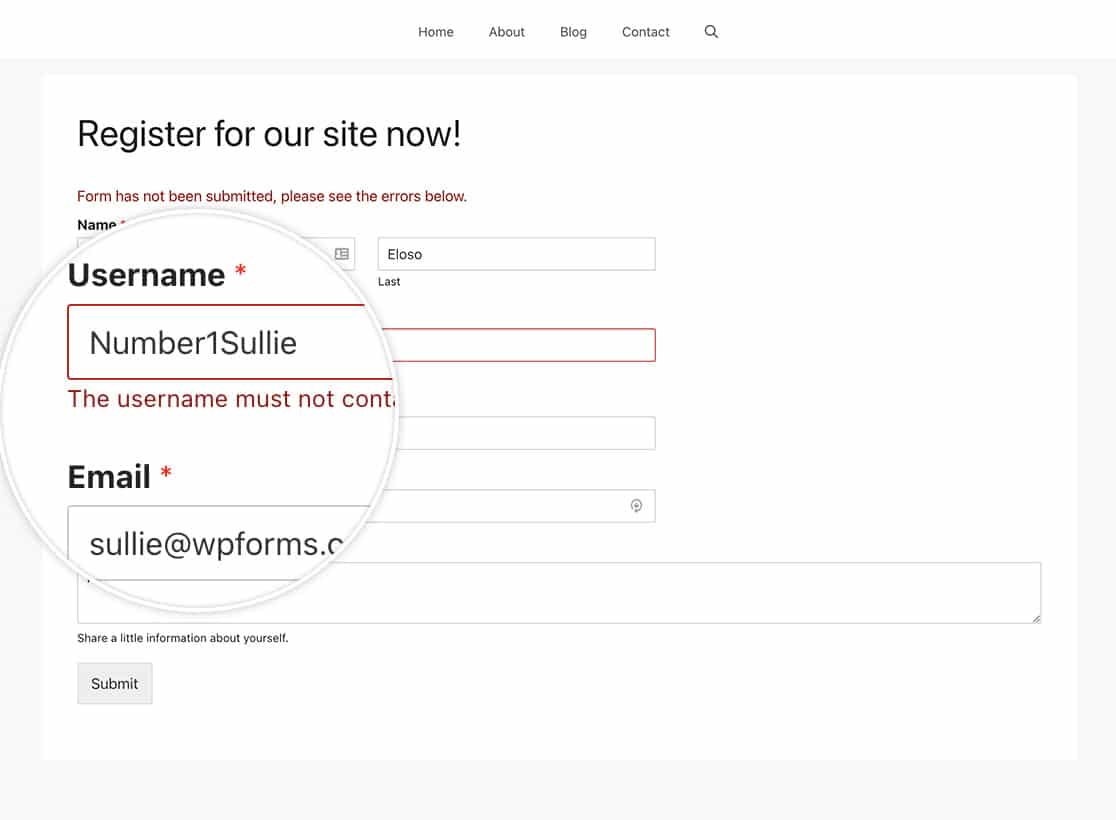
Real-Time Validation Option
If you prefer to prevent users from entering numbers before form submission, you can implement real-time validation. Here’s how:
To begin, edit the form and with the Single Line Text field selected, click the Advanced tab under Field Options and add no-numbers to the CSS Classes field.
Then, add this JavaScript code to your site:
Once the snippet is added, an immediate error message will display alerting the visitor to the validation error when they tab or click out of the field.
Reference Action
Would you also like to prevent special characters from being entered into a Single Line Text form field? Check out the tutorial on How to Restrict Special Characters From a Form Field.