Overview
Would you like to overwrite entries from users who have already submitted a form? In some cases, you wish to overwrite entries so that the user can only submit one entry on each form. With a small PHP snippet, you can allow this on your site for just one single form or even for all forms.
Setup
By default, unless you are using the Form Locker addon, all users can submit as many entries as they wish to all of your forms.
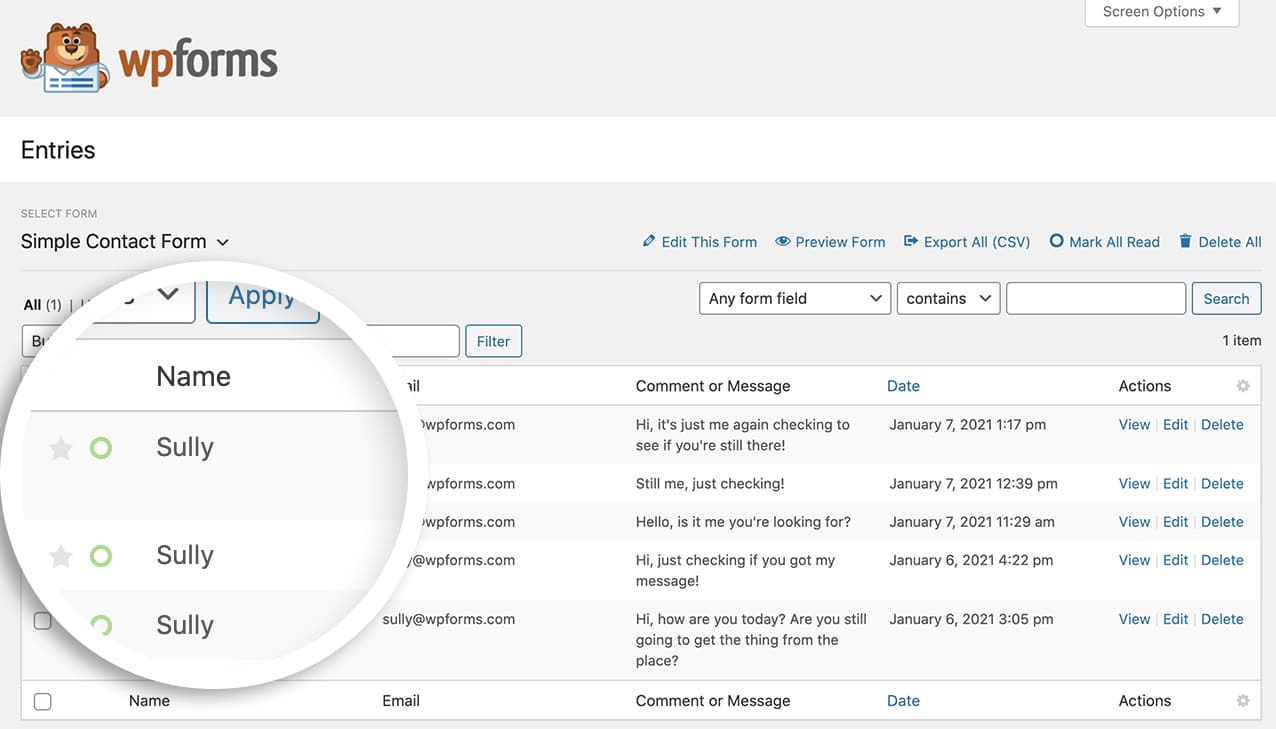
Using this snippet below, this code will look for any previous entries on this form from the user ID and overwrite their entries with the latest entry so each of your members will only have one form entry for each form you’ve created.
If you need any help on adding code snippets to your site, please review this tutorial.
Note: If the user isn’t logged in, this code snippet will not run. This code snippet can only run if a user is logged in when submitting any form entries.
When this user submits a new entry, that entry will overwrite all previous entries on this form.
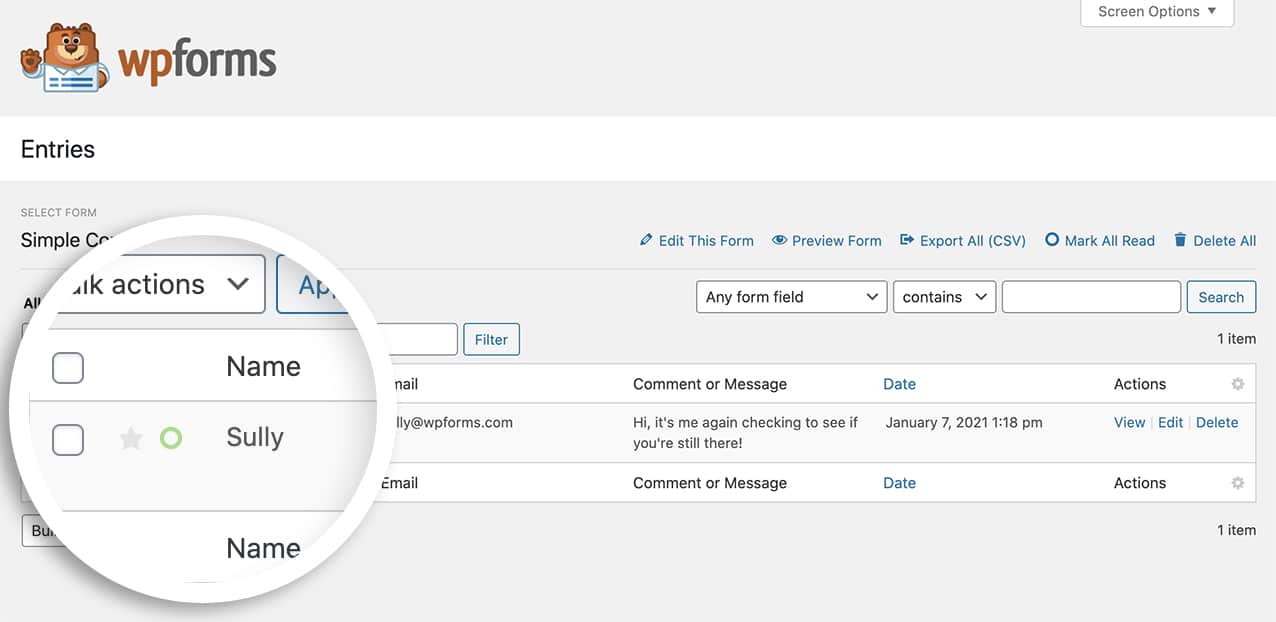
That’s it! You’ve now learned how to overwrite entries previously submitted by a particular user ID.
Next, would you like to learn how to display a message to your visitors if they are already logged in? Take a look at our tutorial on displaying a message when the user is already logged in.
Related
Action Reference: wpforms_process_entry_save