Would you like to limit the range allowed in the Numbers Field with WPForms? There are several ways to set minimum and maximum values for numeric input.
This tutorial will show you the available methods to implement range limits in your forms.
Setting Up the Form
Let’s kick things off by crafting our form and incorporating the Numbers field into it. If you require any guidance on creating your form, please consult this documentation for step-by-step instructions.
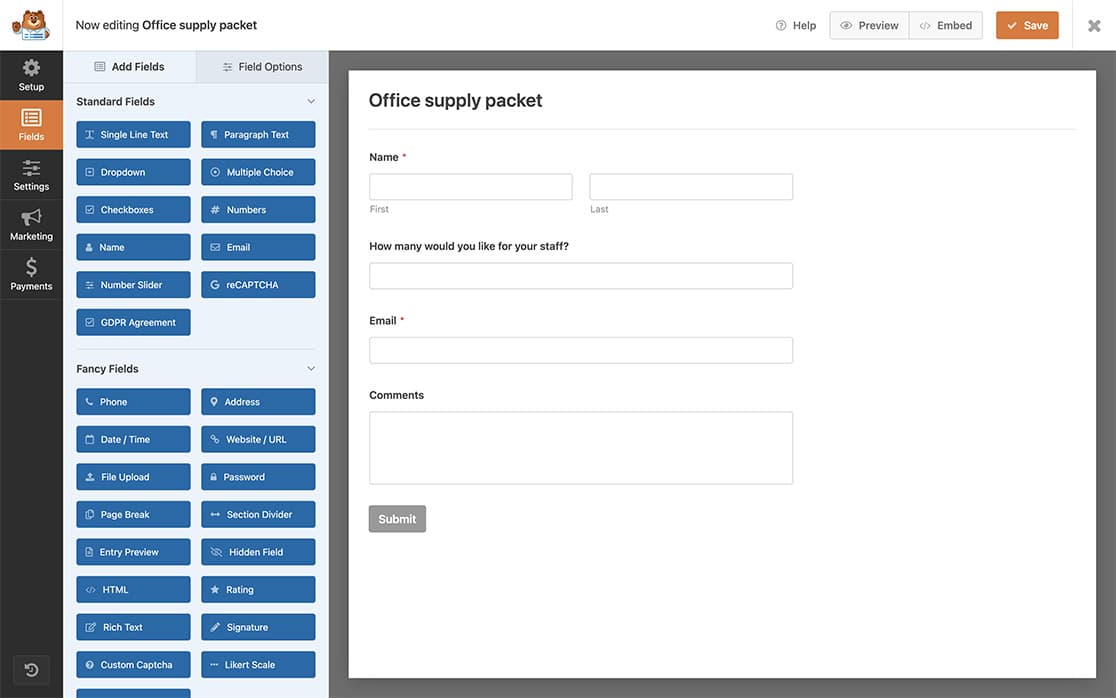
Setting Up Basic Range Limits
The Numbers field includes range controls in the Field Options panel. Simply enter your minimum and maximum values in the Range fields to restrict user input.
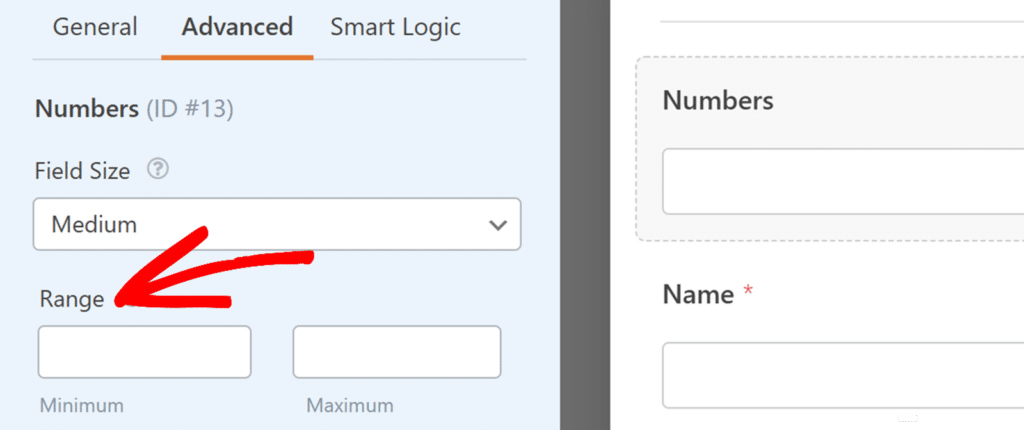
For more details about the Numbers field’s features, check out our guide on using the Numbers field.
Implementing Advanced Range Controls
For specialized requirements such as enforcing even numbers or custom validation messages, you can use JavaScript to implement custom range controls. If you need guidance on adding code snippets, please refer to this guide on adding custom code in WordPress.
Adding the CSS class
First, we’re going to add a CSS class to the field to make sure that anytime there is a Numbers field with this particular class, this snippet would be called into action. To add a CSS class to a field, open the form builder, click the Numbers to open the Field Options panel. Then click Advanced and in the CSS Classes, add wpf-num-limit
.
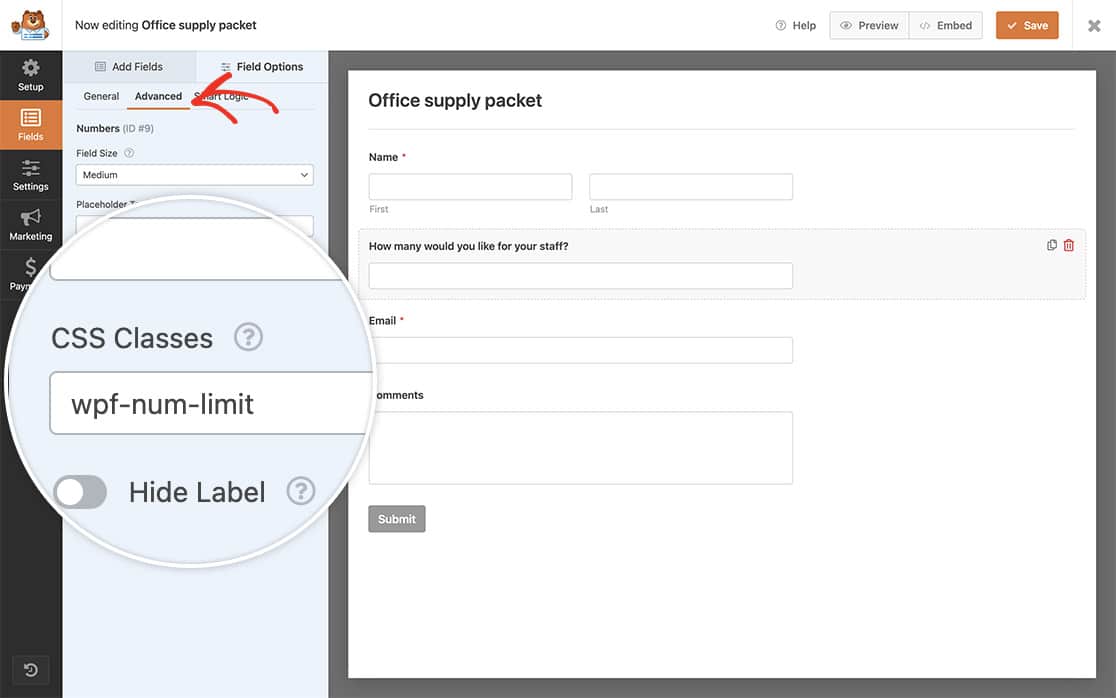
Adding the Code Snippet
Now, let’s integrate the snippet.
This particular snippet enforces a minimum value of 5 and a maximum value of 20 for any Numbers field featuring a CSS class labeled wpf-num-limit
.
/**
* Limit number range allowed for a Numbers field
* Apply the class "wpf-num-limit" to the field to enable.
*
* @link https://wpforms.com/developers/how-to-limit-range-allowed-in-numbers-field/
*/
function wpf_dev_num_limit() {
?>
<script type="text/javascript">
jQuery(function(){
// Enter 5 minimum (5) and maximum (20) amount for the number field
jQuery( '.wpf-num-limit input' ).attr({ 'min':5, 'max':20 } );
});
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_num_limit', 30 );
After saving the form, users will encounter a validation error if they attempt to input a number falling outside the defined range in the code.
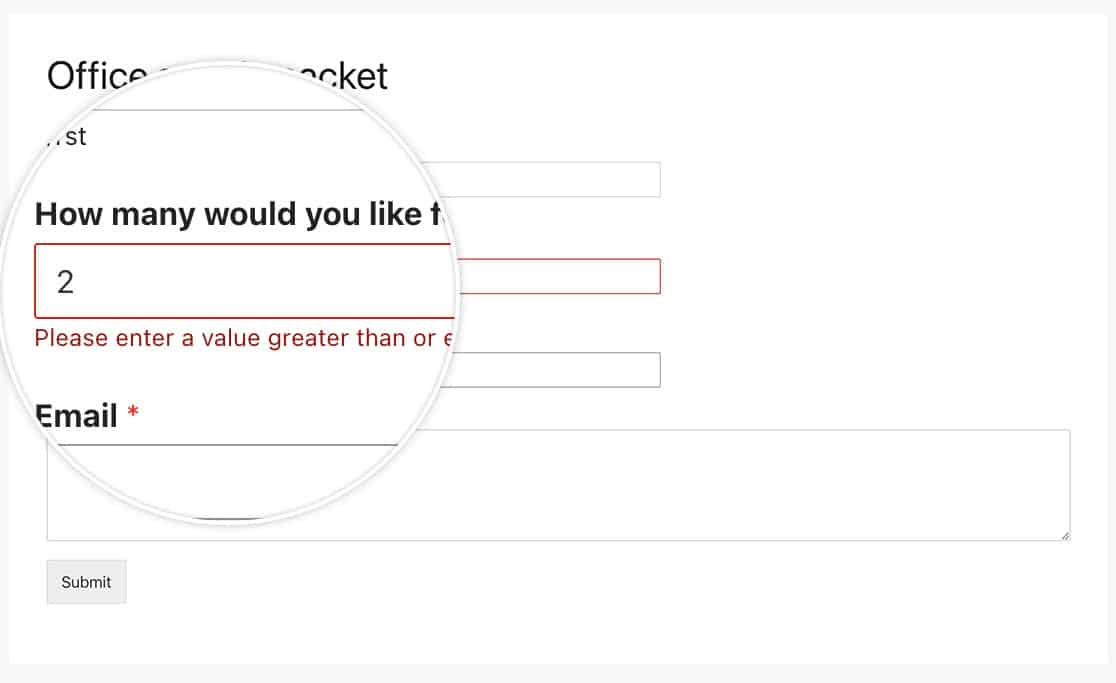
Limiting Even Numbers Only
To add a step value to enforce selection of even numbers only, use the following code snippet:
/**
* Limit number range in multiples of 2
* Apply the class "wpf-num-limit" to the field to enable.
*
* @link https://wpforms.com/developers/how-to-limit-range-allowed-in-numbers-field/
*/
function wpf_dev_num_limit() {
?>
<script type="text/javascript">
jQuery(function(){
// Minimum accepted number is 20
// Maximum accepted number is 40
// Only accept multiples of 2
jQuery( '.wpf-num-limit input' ).attr( { 'min':20, 'max':40, 'step': 2 } );
});
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_num_limit', 30 );
Working with Multiple Forms
Use the following snippet when using different CSS classes to set unique ranges for different forms:
In this example, we’re using the wpf-num-limit-max-under–twenty and wpf-num-limit-max-under-fifty.
/**
* Two different CSS classes in the same function
* Apply the class "wpf-num-limit" to the field to enable.
*
* @link https://wpforms.com/developers/how-to-limit-range-allowed-in-numbers-field/
*/
function wpf_dev_num_limit() {
?>
<script type="text/javascript">
jQuery(function(){
// Minimum set to 0, maximum set to 20
jQuery( '.wpf-num-limit-max-under-twenty input' ).attr({ 'min':0, 'max':20 });
// Minimum set to 21, maximum set to 50
jQuery( '.wpf-num-limit-max-under-fifty input' ).attr({ 'min':21, 'max':50 });
});
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_num_limit', 30 );
Customizing the Error Message
Use the following code snippet to change the default error message that appears when users enter invalid numbers.
/**
* Limit number range allowed for a Numbers field
* Apply the class "wpf-num-limit" to the field to enable.
*
* @link https://wpforms.com/developers/how-to-limit-range-allowed-in-numbers-field/
*/
function wpf_dev_num_limit() {
?>
<script type="text/javascript">
jQuery(function(){
// Minimum 5, maximum 20
jQuery( '.wpf-num-limit input' ).attr({ 'min':5, 'max':20 } ); // Define number limits
});
// Message to be displayed if the min and or max is not met
jQuery.extend(jQuery.validator.messages, {
max: jQuery.validator.format( "We apologize, there can only be a max allowance of {0}." ),
min: jQuery.validator.format( "We apologize, there needs to be a minimum allowance of {0}." )
});
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_num_limit', 30 );
That concludes the process! You have successfully set both a minimum and maximum value permitted for your Numbers field. If you wish to customize the required field indicator, you can explore our article on How to Change the Required Field Indicator for further details.