Would you like to customize the Dynamic Choices options for your Checkbox, Multiple Choice, and Dropdown fields? If you’re using Dynamic Choices but want to exclude specific posts, pages, or categories, then this is the tutorial for you!
The Multiple Choices, Checkboxes, and Dropdown fields can be configured to dynamically display post or page titles as Dynamic Choices.
By default when Dynamic Choices is enabled, all published items of that specific post type or taxonomy will display.
For example, when Dynamic Choices is set to Post Type, and the Dynamic Post Type Source is set to Posts, all published posts will be displayed as field choices.
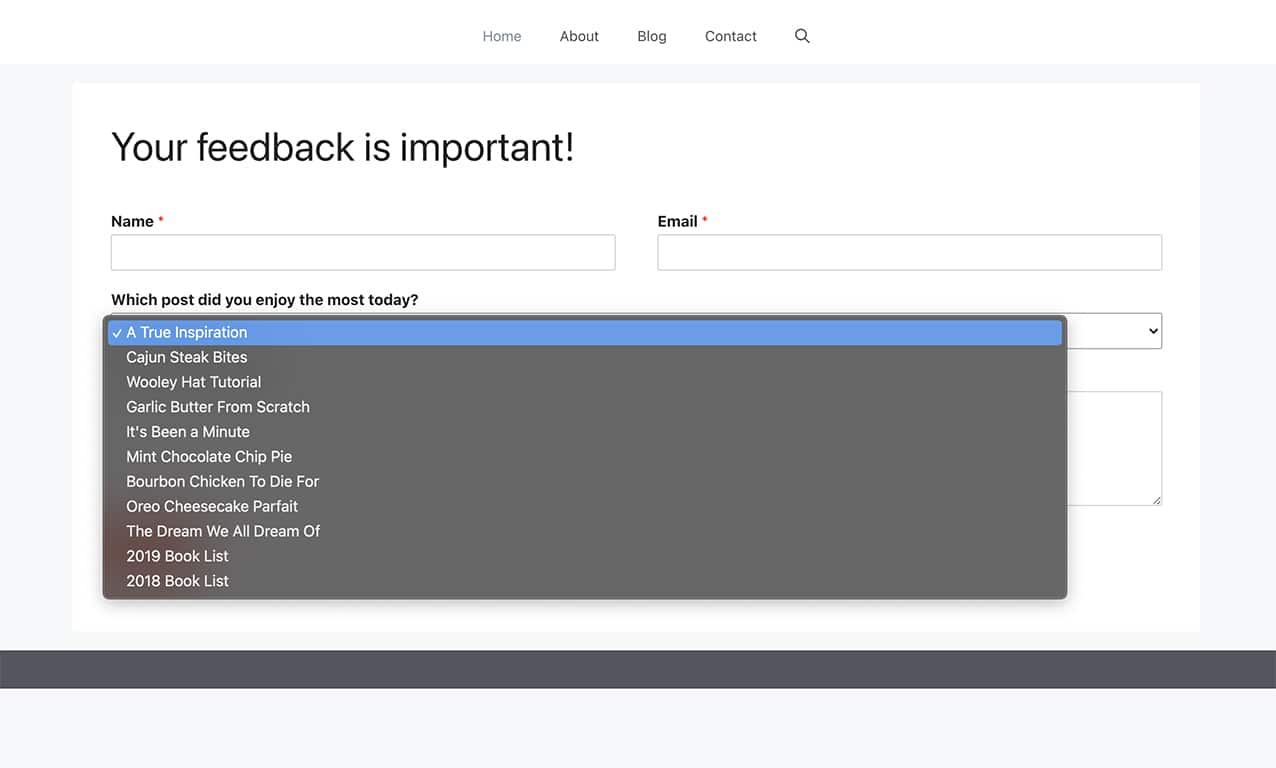
For our tutorial, we’ll display a dropdown of dynamically generated post titles from our site.
Creating the Form
To begin, we’re going to create a form that captures the name, email, the ability for the visitor to select which post they liked best, and then a comments section as well.
For this reason, we’ll add a Name, Email, Dropdown, and Paragraph Text form fields to our form.
Once you’ve added the Dropdown field, click the Advanced Options and in the Dynamic Choices dropdown click Post Type. This will bring up another dropdown for Dynamic Post Type Source. When you see this second dropdown, select Posts from the dropdown and click Save on the form.
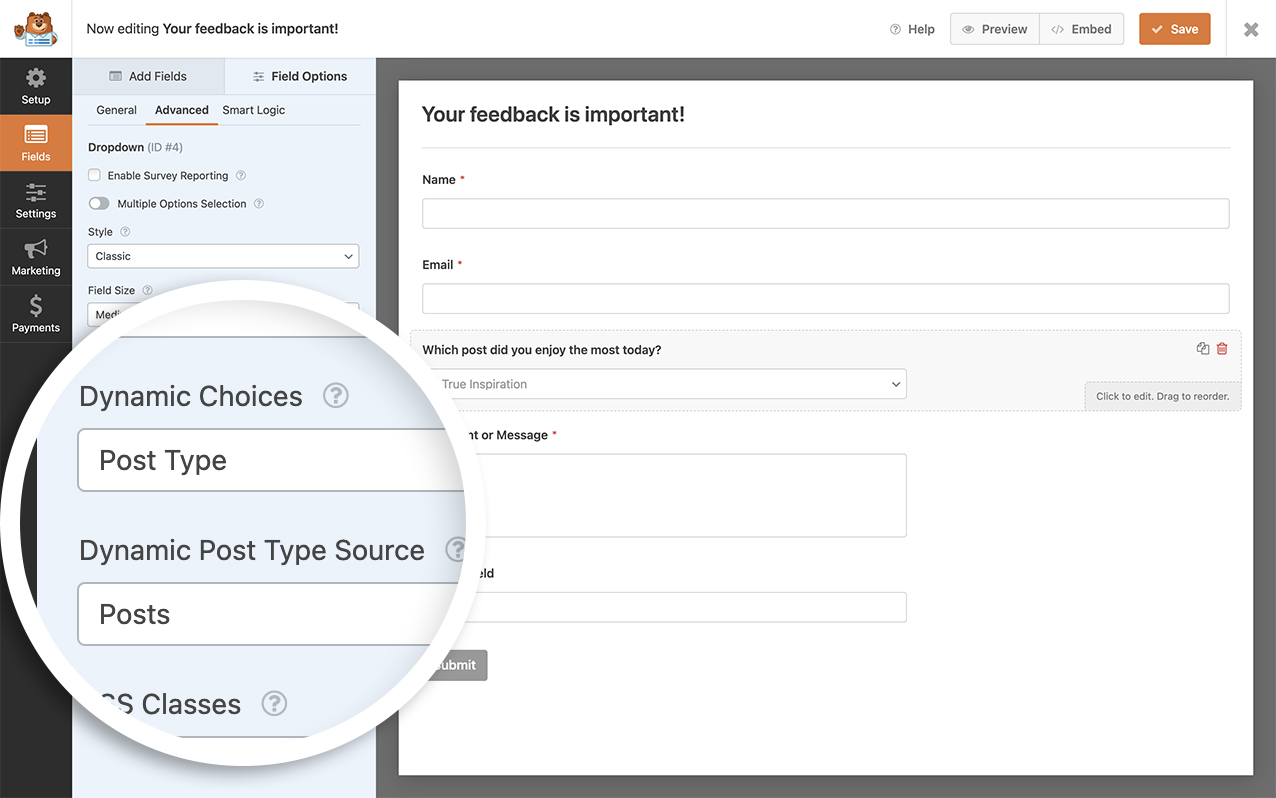
Adding the Snippet to Include Posts from a Single Category
You can even customize these options further by using code snippets. You can only include posts from a particular category or even exclude posts from a particular category.
For instance, in our tutorial, we want to only show posts from a specific category, so our code snippet will be added that will only populate our Dropdown with posts from category 11.
For help in adding code snippets to your site, please see this tutorial.
/**
* Limit posts or pages displayed by category.
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dynamic_choices_categories( $args, $field, $form_id ) {
// For field #10 in form #851, only show entries in category #37
if ( '851' == $form_id && '10' == $field[ 'id' ] ) {
$args[ 'category' ] = '22';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_dynamic_choices_categories', 10, 3 );
Remember to change your form and field ID in your code snippets to match your form and field ID number. If you need help finding your form and field ID numbers, please review this tutorial.
Limiting Multiple Forms for Multiple Category IDs
Did you know that you can also have this code snippet for various forms and fields?
The code below limits dynamic options within two different forms:
- Field ID
1
of form ID363
to display only posts with the category ID11
- Field ID
20
of form ID225
to display only posts from category ID5
/**
* Limit posts or pages displayed by category.
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dynamic_choices_categories( $args, $field, $form_id ) {
// For field #1 in form #363, only show entries in category #11
if ( '363' == $form_id && '1' == $field[ 'id' ] ) {
$args[ 'category' ] = '11';
// For field #20 in form #225, only show entries in category #5
} elseif ( '225' == $form_id && '20' == $field['id'] ) {
$args[ 'category' ] = '5';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_dynamic_choices_categories', 10, 3 );
Limiting by Taxonomy
A taxonomy is a term used in WordPress to define things like Categories and Tags.
If the Dynamic Choices is set to Taxonomy, and the Dynamic Taxonomy Source is set to Categories, all categories will be used for the field choices.
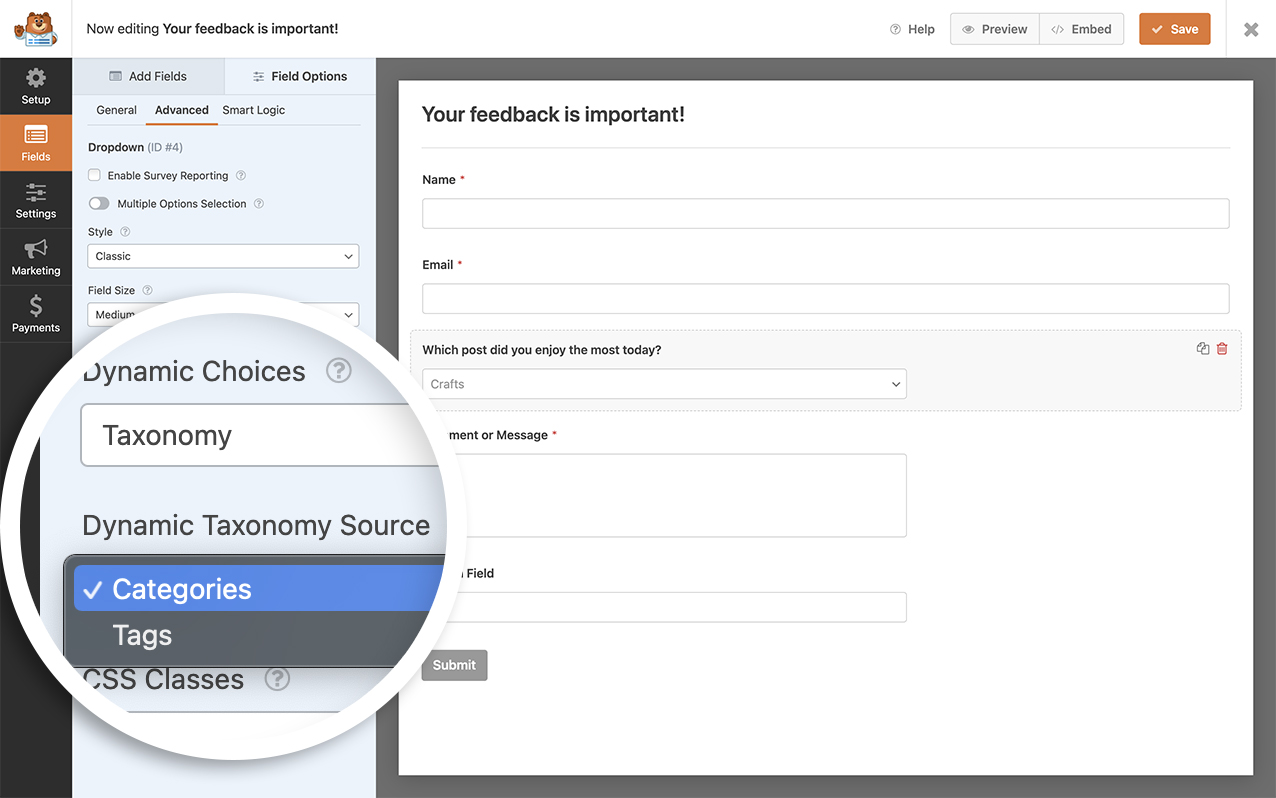
However, you can exclude specific categories using the snippet below.
/**
* Exclude categories from dynamic choices.
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dev_dynamic_choices_exclude( $args, $field, $form_id ) {
if ( is_array( $form_id ) ) {
$form_id = $form_id[ 'id' ];
}
// Only on form #212 and field #16
if ( $form_id == 212 && $field[ 'id' ] == 16 ) {
// Category IDs to exclude
$args[ 'exclude' ] = '4,5,6,11';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_taxonomy_args', 'wpf_dev_dynamic_choices_exclude', 10, 3 );
This snippet will exclude categories with IDs 4,5,6, and 11 from the dynamic choice options. If you replace exclude
with include
in the snippet above, it’ll only show categories with the IDs you’ve added.
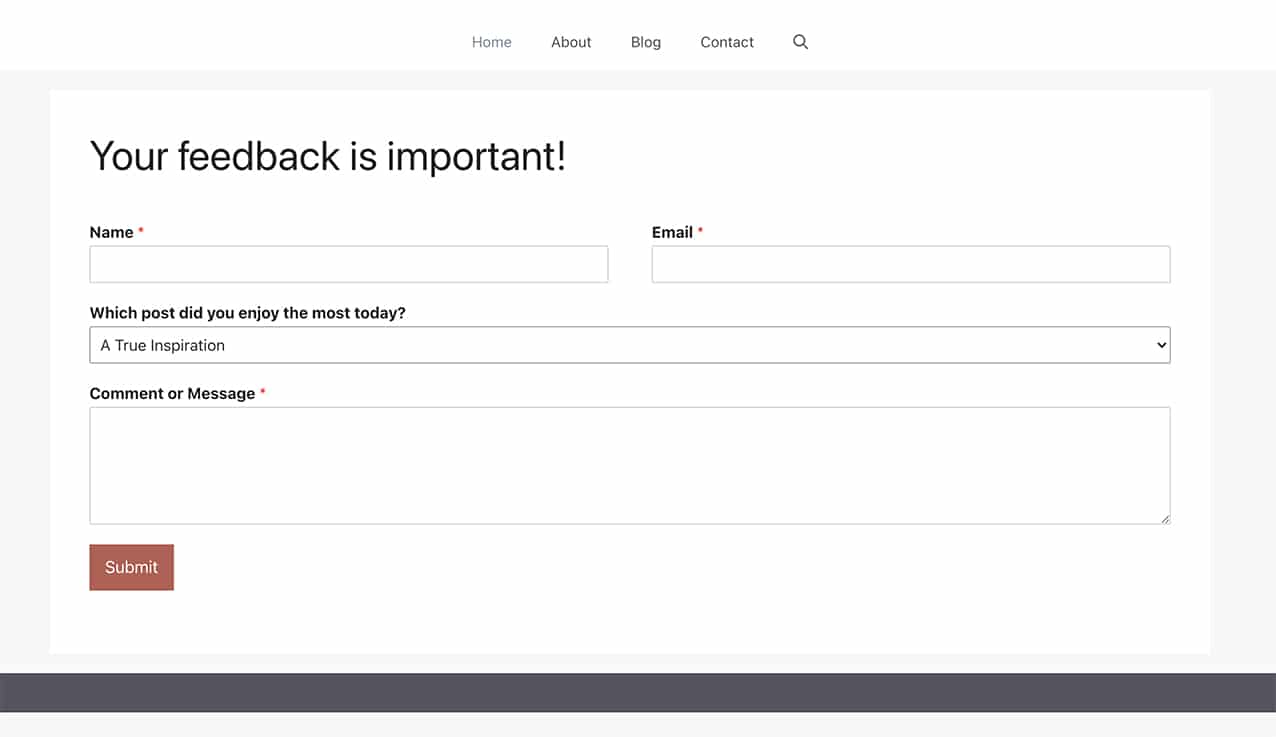
Displaying Only Future Posts
/*
* Only display future posts in the dynamic choices
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dev_only_future_posts( $args, $field, $form_id ) {
// Only run this snippet on form ID 2575 & only on field ID 19
if ( '2575' == $form_id && '19' == $field[ 'id' ] ) {
// If the Dynamic Choices is set to Post Type
// Look for and only display the posts that have been scheduled with a future date
$args[ 'post_status' ] = [ 'publish', 'future' ];
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_dev_only_future_posts', 10, 3 );
Displaying Only Posts From the Current Logged-in User
/*
* Set the number of days to delete partial entries when using the Save and Resume addon
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dev_only_loggedin_user_posts( $args, $field, $form_id ) {
// Only run this snippet on form ID 2519 & only on field ID 3
if ( '2519' == $form_id && '3' == $field[ 'id' ] ) {
// If the Dynamic Choices is set to Post Type
// Look for and only display the posts that have been scheduled with a future date
$args[ 'author' ] = get_current_user_id();
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_dev_only_loggedin_user_posts', 10, 3 );
Showing Posts from a Particular Author ID
/**
* Only show posts from a particular author ID
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dynamic_choices_author( $args, $field, $form_id ) {
// Only run on form ID 526
if ( '526' == $form_id ) {
// The author ID is equal to 9
$args[ 'author' ] = '9';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_dynamic_choices_author', 10, 3 );
Displaying Only Child Categories
/**
* Display only child categories
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dev_dynamic_choices_include( $args, $field, $form_id ) {
if ( is_array( $form_id ) ) {
$form_id = $form_id[ 'id' ];
}
// Only on form #122 and field #11
if ( $form_id == 122 && $field[ 'id' ] == 11 ) {
// Category IDs to include - 87 is parent; 88 and 89 are child categories of this parent
$args[ 'include' ] = '87,88,89';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_taxonomy_args', 'wpf_dev_dynamic_choices_include', 10, 3 );
FAQs
Q: How can I customize this for a Woocommerce category?
A: For Woocommerce taxonomies, on the Advanced tab of your Checkbox, Dropdown, or Multiple Choice field, set the Dynamic Choices to Product, and for the Dynamic Taxonomy Source, select Product categories.
Then if you only wanted to include specific categories, you would find the ID in the same manner as you did above. From the WordPress admin, click Categories found under Products in the WordPress admin menu on the left. Click each Product Category you want the ID. The URL will look something like this:?taxonomy=product_cat&tag_ID=90&post_type=product&wp_http_referer=%2Fwp-admin%2Fedit-tags.php%3Ftaxonomy%3Dproduct_cat%26post_type%3Dproduct
For this example, the ID number would be 90
Therefore, your snippet would be as follows.
/**
* Include certain Woocommerce categories from dynamic choices.
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dev_dynamic_choices_exclude( $args, $field, $form_id ) {
if ( is_array( $form_id ) ) {
$form_id = $form_id[ 'id' ];
}
// Only on form #2620 and field #11
if ( $form_id == 2620 && $field[ 'id' ] == 11 ) {
// Product Category IDs to include
$args[ 'include' ] = '90,95,98';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_taxonomy_args', 'wpf_dev_dynamic_choices_exclude', 10, 3 );
Q: How can I show only certain products from a Woocommerce category?
A: Of course. You’d set up your Dynamic Choices toProduct and for the Dynamic Taxonomy Source, select Product categories.
And instead of using the product category ID, you would use the name.
/**
* Include products from a specific category from dynamic choices.
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_dev_dynamic_choices_exclude( $args, $field, $form_id ) {
if ( is_array( $form_id ) ) {
$form_id = $form_id[ 'id' ];
}
// Only on form #2620 and field #11
if ( $form_id == 2620 && $field[ 'id' ] == 11 ) {
// Category IDs to exclude
$args[ 'product_cat' ] = 'Decor';
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_dev_dynamic_choices_exclude', 10, 3 );
Decor is the name of our Product Category. Remember to change this to match your own needs.
Q: How can I show posts from a custom taxonomy in a WPForms dropdown field?
A: To display posts from a custom taxonomy in a WPForms dropdown field, you need to modify the dynamic choice arguments. Currently, the provided examples in our documentation cover categories. Here’s an example that shows how to customize the code to fetch posts from a specific taxonomy.
/**
* Customize WPForms dynamic choices to show posts from a specific taxonomy.
*
* @link https://wpforms.com/developers/how-to-exclude-posts-pages-or-categories-from-dynamic-choices/
*/
function wpf_custom_dynamic_choices( $args, $field, $form_id ) {
// For field #4 in form #25107012, only show entries in taxonomy term ID 8
if ( '25107012' == $form_id && '4' == $field['id'] ) {
$args[ 'tax_query' ] = array(
array(
'taxonomy' => 'samples', // Replace with your custom taxonomy
'field' => 'term_id',
'terms' => array( 8 ), // Replace with your term ID
),
);
}
return $args;
}
add_filter( 'wpforms_dynamic_choice_post_type_args', 'wpf_custom_dynamic_choices', 10, 3 );
The tax_query
array is used to specify the custom taxonomy (samples in this example) and the term ID (8 in this example). You should replace ‘samples’ and 8 with your own taxonomy and term ID as needed. For more information on tax queries, please check out the WordPress documentation.
And that’s it! You’ve now learned the various ways to customize dynamic choices in WPForms.
Would you like to know how to add field values for these same form fields? Take a look at our article on How to Add Field Values for Dropdown, Checkboxes, and Multiple Choice Fields.