Would you like to display an image after your form? With a small PHP snippet, you can easily add your image, a video, or a message just under the Submit button.
In this tutorial, we’re going to walk you through the steps on how to achieve this.
Creating the form
First, you’ll need to create a new form or edit an existing one to access the form builder. In the form builder, go ahead and add the fields you need to the form.
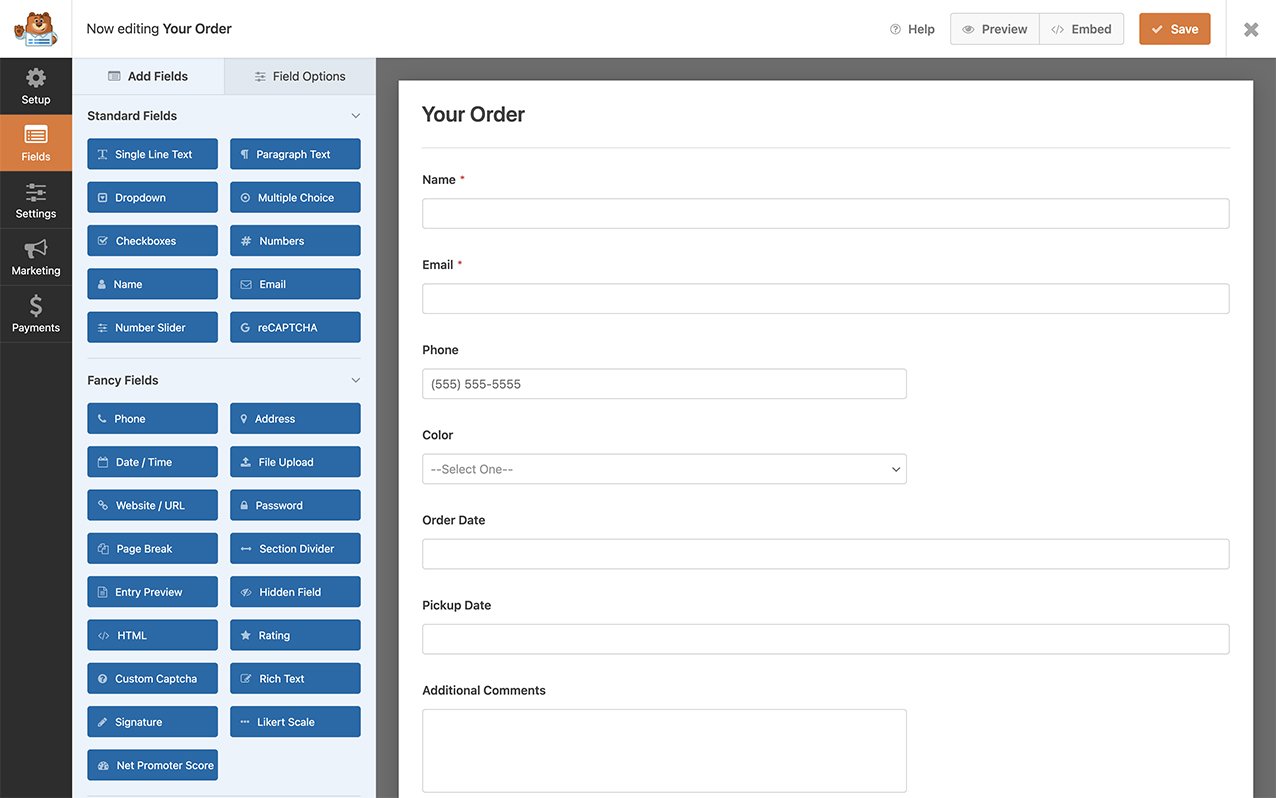
Adding the Snippet
This code snippet can be used to add anything below your form. You can add an image, a video, or just text. In order to add our image after the Submit button, you’ll need to copy and paste this snippet to your site.
If you need help in adding snippets to your site, please review this tutorial.
In our example, we’re limiting this snippet to only the form ID 999. You’ll need to replace this ID with the ID of the form you wish to target. If you’re not sure what your form ID is and need some help in finding it, please check out this tutorial.
/**
* Output something after your form(s).
*
* @link https://wpforms.com/developers/how-to-display-an-image-after-your-form/
*/
function wpf_dev_frontend_output_after( $form_data, $form ) {
// Optional, you can limit to specific forms. Below, we restrict output to
// form #999.
if ( absint( $form_data[ 'id' ] ) !== 999 ) {
return;
}
// Run code or see example echo statement below.
echo '<img src="'.get_template_directory_uri().'/images/secured-site.jpg" alt="Verified by Visa" width="80" height="80">';
}
add_action( 'wpforms_frontend_output_after', 'wpf_dev_frontend_output_after', 10, 2 );
The code above src="'.get_template_directory_uri().'/images/
looks for a directory (a folder) on your server called images inside your theme directory. So the image needs to be in the images folder on your server.
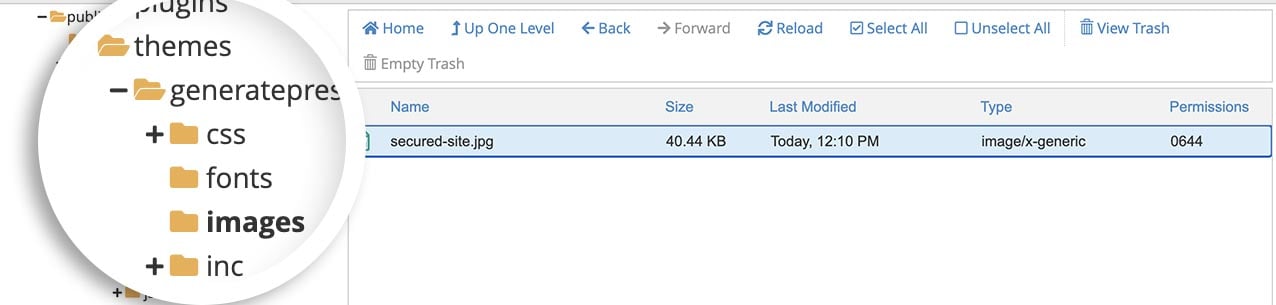
Alternatively, you can simply upload an image to your site media library and update the value after src= with the image URL. Here’s how the updated snippet will look if you use an image from the WordPress media library.
/**
* Output something after your form(s).
*
* @link https://wpforms.com/developers/how-to-display-an-image-after-your-form/
*/
function wpf_dev_frontend_output_after( $form_data, $form ) {
// Optional, you can limit to specific forms. Below, we restrict output to
// form #999.
if ( absint( $form_data[ 'id' ] ) !== 999 ) {
return;
}
// Run code or see example echo statement below.
echo '<img src="http://myexamplesite.com/wp-content/uploads/2021/01/image-name.jpg " alt="Verified by Visa" width="80" height="80">';
}
add_action( 'wpforms_frontend_output_after', 'wpf_dev_frontend_output_after', 10, 2 );
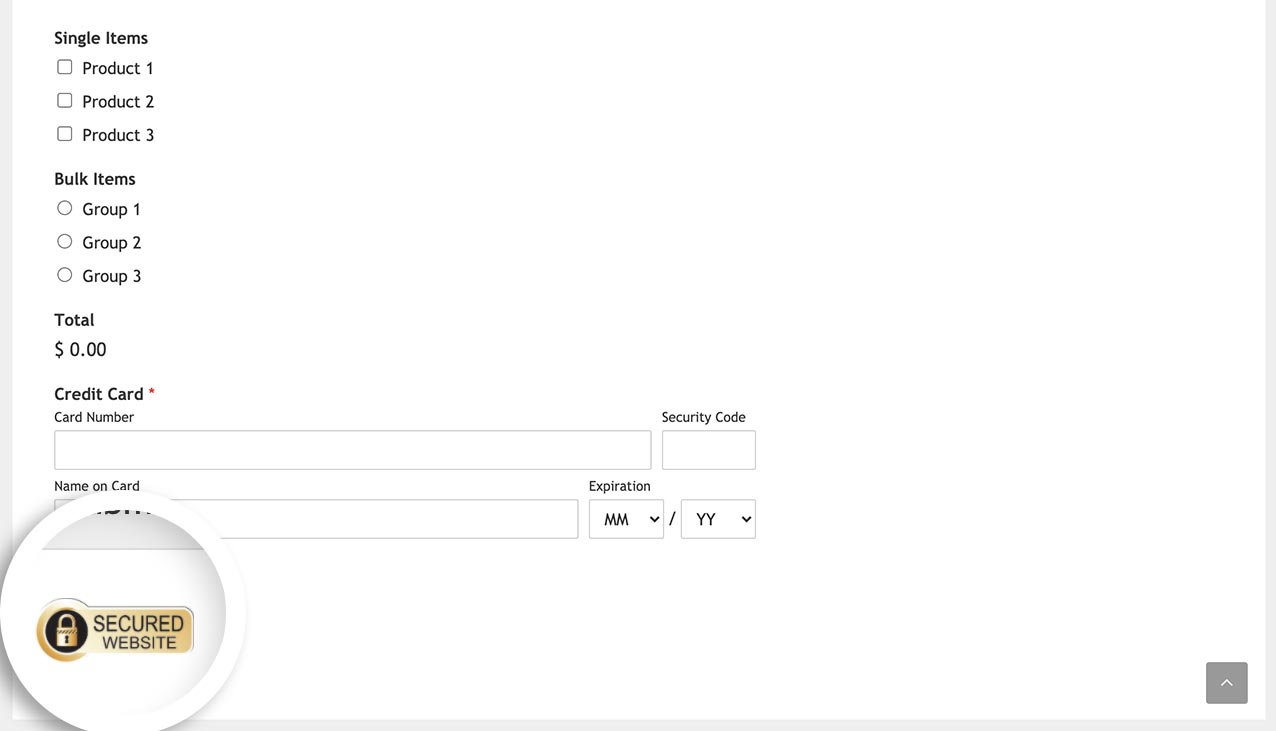
Frequently Asked Questions
These are answers to some of the top questions we see about adding images after the submit button in WPForms.
Q: How can I display text with a link after the form?
A: If you just want to display some text with a link, you can use this snippet.
In this example, we will display a “Powered by Stripe” message with a link that will open a new window to Stripe.
/**
* Output Text after your form(s).
*
* @link https://wpforms.com/developers/wpforms_frontend_output_after/
*/
function wpf_dev_frontend_output_after_display_text( $form_data, $form ) {
// Optional, you can limit to specific forms. Below, we restrict output to
// form #999.
if ( absint( $form_data[ 'id' ] ) !== 999 ) {
return;
}
// Run code or see example echo statement below.
echo _e( '<p> Powered by <strong> <a href="https://stripe.com/" target="_blank">Stripe</a> </strong> | <a href="link-to-terms" target="_blank">Terms and Privacy!</a>.', 'plugin-domain' );
}
add_action( 'wpforms_frontend_output_after', 'wpf_dev_frontend_output_after_display_text', 10, 2 );
That’s it! You’ve successfully added an image after your form.
Next, would you like to change the pre-loading image that shows when you click submit? Take a look at our article on how to change the pre-loader icon on submit.
Related
Action Reference: wpforms_frontend_output_after