Would you like to customize how dates appear in your forms? While WPForms provides three default date formats in the Date / Time field’s Date Picker, you can easily add more formats to match your needs.
This guide will show you how to create custom date formats using PHP.
By default, the Date / Time field’s Date Picker provides three different date formats to choose from.
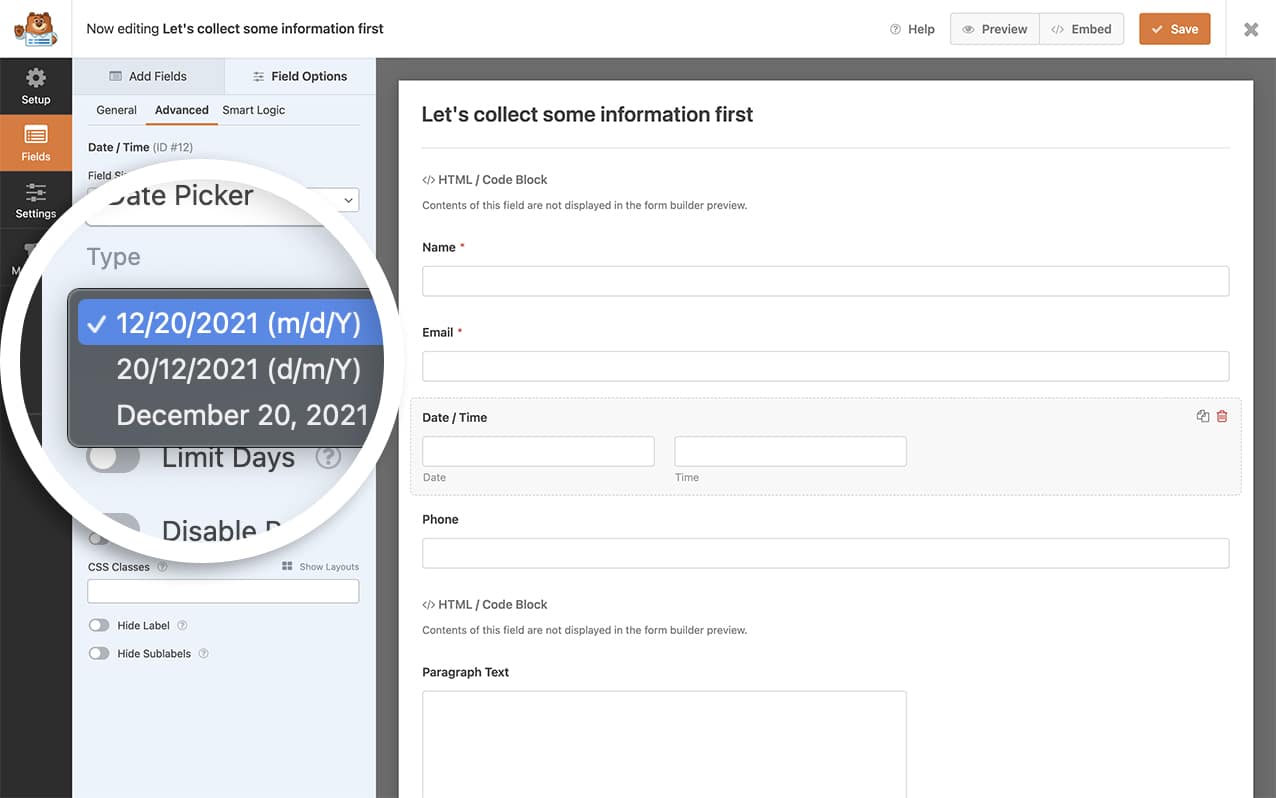
Setting Up Custom Date Formats
To add new date formats, you’ll need to add a code snippet to your site. If you’re not sure how to add custom code, please see our guide on how to add code snippets.
/**
* Add additional formats for the Date field Date Picker
*
* @link https://wpforms.com/developers/how-to-create-additional-formats-for-the-date-field/
*/
function wpf_dev_date_field_formats( $formats ) {
// Item key is JS date character - see https://flatpickr.js.org/formatting/
// Item value is in PHP format - see http://php.net/manual/en/function.date.php
// Adds new format Monday, 20th December 2021
$formats[ 'l, J F Y' ] = 'l, jS F Y';
return $formats;
}
add_filter( 'wpforms_datetime_date_formats', 'wpf_dev_date_field_formats', 10, 1 );
This date format will allow us to enter the full date in a longer format Monday, 20th December 2021. To find more formats such as this, see PHP’s official format documentation.
Using Custom Separators
To change the date separator (e.g., from / to -), use this code:
/**
* Change date separator format
*/
function wpf_dev_date_field_formats( $formats ) {
// Adds new format 24-07-2021
$formats[ 'j-m-y' ] = 'j-m-y';
return $formats;
}
add_filter( 'wpforms_datetime_date_formats', 'wpf_dev_date_field_formats', 10, 1 );
Adding Words to Date Formats
To include words in your date format (like “Monday, 20th of December 2021”), use this code:
/**
* Add words to date format
*/
function wpf_dev_date_field_formats( $formats ) {
// Adds new format Monday, 20th of December 2021
$formats[ 'l, J \of F Y' ] = 'l, jS \of F Y';
return $formats;
}
add_filter( 'wpforms_datetime_date_formats', 'wpf_dev_date_field_formats', 10, 1 );
Creating the Form
Next, we’re going to create our form and add our fields. This will include one Date / Time form field.
If you need any assistance in creating a form, please see this documentation.
Once you’ve added your Date / Time form field, click on the Field Options tab and select Advanced to select the Format and select your new format.
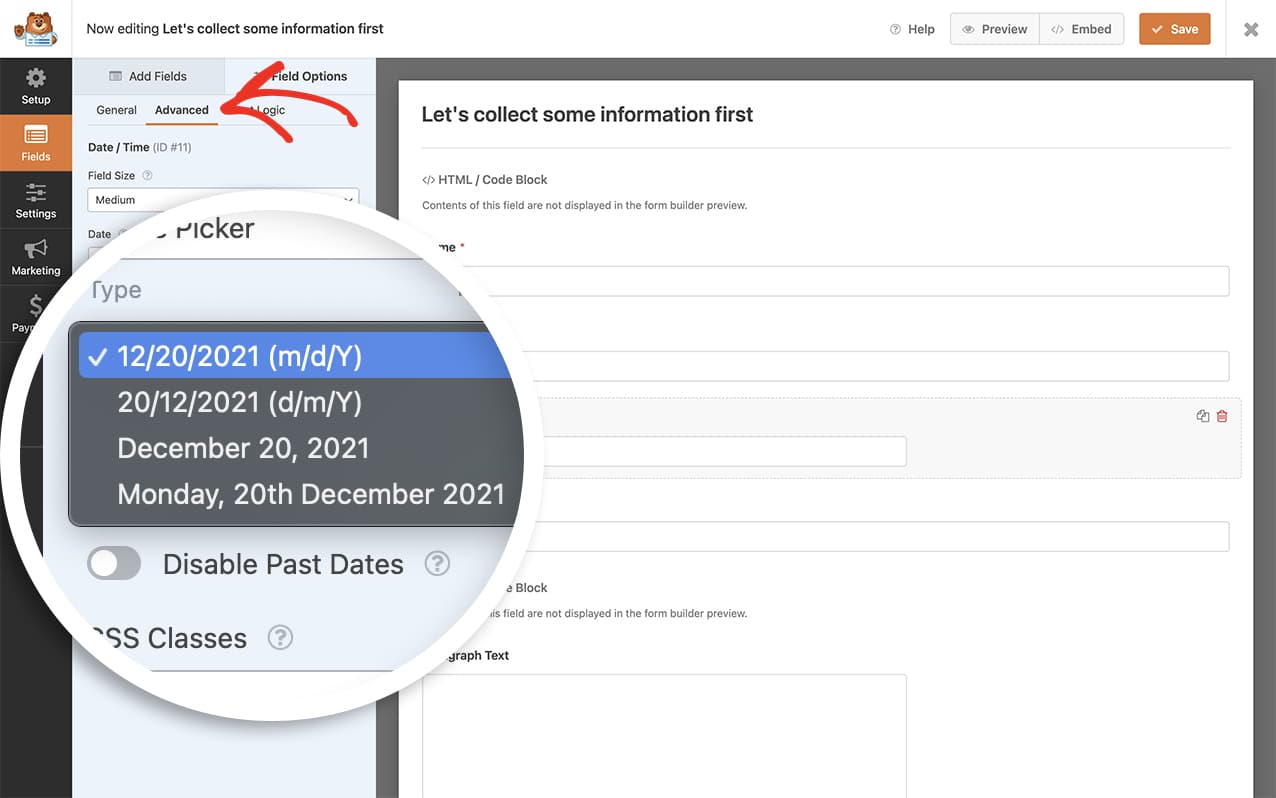
And that’s all you need to create additional date formats for your Date field Date Picker. Would you like to customize the Time Picker as well? Please review our tutorial on How to Customize the Date Time Field Time Picker.
Reference Filter
Filter Reference: wpforms_datetime_date_formats