Would you like to prevent specific names from submitting your forms? With a simple validation snippet, you can block particular names from completing your form.
This tutorial will show you how to implement name blocking in your forms step by step.
Setting Up Your Form
First, create a new form and add your Name field. For this tutorial, we’ll be using the First Last format for the Name field. If you need help creating your form, please review our form creation documentation.
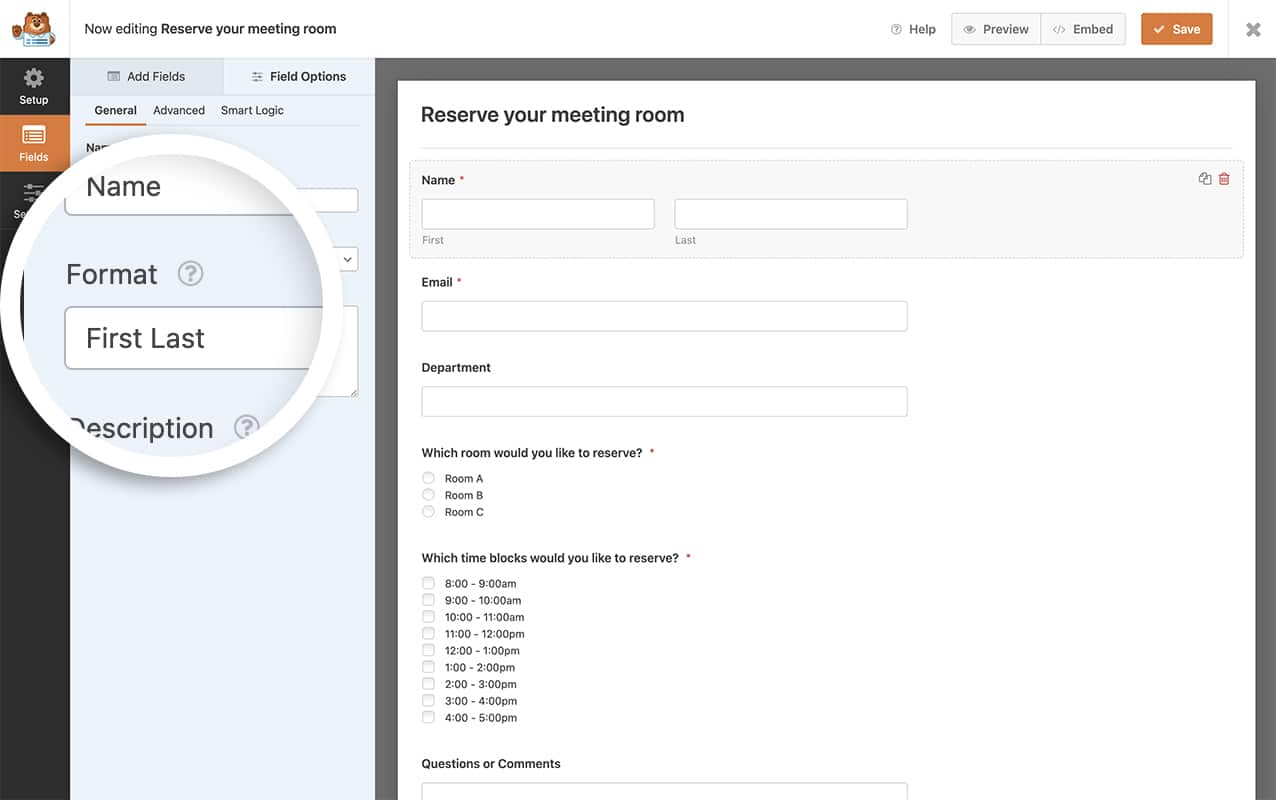
Adding the Name Blocking Code
Now let’s add the code that will prevent specific names from submitting the form. If you need help adding code snippets to your site, please check out this tutorial on adding custom code snippets.
Simple format
First Last and First Middle Last format
Customizing the Code Snippet
You’ll need to modify a few key values in the code:
- On line 10, replace 1000 with your form’s ID
- On line 10, replace 10 with your Name field’s ID
- On lines 19-21, update the blocked_names array with your list of names to block
If you need help finding these IDs, please review this tutorial on locating form and field IDs.
This code works by:
- Checking if the submission is from your specific form and field
- Combining the first and last name entries
- Comparing the submitted name against your blocked names list
- Displaying an error message if the name matches any blocked name
Frequently Asked Questions
Can I block partial names?
Yes! You can modify the preg_match pattern
in the code to match partial names. Contact our support team for specific examples.
And that’s all you need, would you like to also block profanity on your form? Take a look at our article on How to Block Form Submissions Containing Profanity.