Would you like to customize address formats for different countries? WPForms allows you to add custom address schemes beyond the default US and International formats.
This guide will show you how to create new address schemes for countries like Canada, Australia, or any custom format you need.
Setting Up Address Schemes
To begin, you’ll need to add a code snippet to your site. If you’re not sure how to add custom code, please see our guide on how to add code snippets to your site.
Here’s a code snippet for creating a Canadian address format:
/**
* Add new address field scheme for Canada
*
* @link https://wpforms.com/developers/create-additional-schemes-for-the-address-field
*/
function wpf_dev_new_address_scheme( $schemes ) {
$my_form_id = 1000; // Replace with your form ID
$schemes['canada'] = array(
'label' => 'Canada',
'address1_label' => 'Address Line 1',
'address2_label' => 'Address Line 2',
'city_label' => 'City',
'postal_label' => 'Code Postal',
'state_label' => 'Province',
'states' => array(
'BC' => 'British Columbia',
'ON' => 'Ontario',
'QC' => 'Quebec',
'AB' => 'Alberta',
'MB' => 'Manitoba',
'NB' => 'New Brunswick',
'NL' => 'Newfoundland and Labrador',
'NT' => 'Northwest Territories',
'NS' => 'Nova Scotia',
'NU' => 'Nunavut',
'PE' => 'Prince Edward Island',
'SK' => 'Saskatchewan',
'YT' => 'Yukon',
),
);
return $schemes;
}
add_filter( 'wpforms_address_schemes', 'wpf_dev_new_address_scheme', 10, 1 );
For an Australian address format, use this code:
/**
* Add new address field scheme for Australia
*
* @link https://wpforms.com/developers/create-additional-schemes-for-the-address-field
*/
function wpf_dev_new_address_scheme( $schemes ) {
$schemes['australia'] = array(
'label' => 'Australia',
'address1_label' => 'Address Line 1',
'address2_label' => 'Address Line 2',
'city_label' => 'City',
'postal_label' => 'Postal',
'state_label' => 'State / Territory',
'states' => array(
'NSW' => 'New South Wales',
'VIC' => 'Victoria',
'QLD' => 'Queensland',
'WA' => 'Western Australia',
'SA' => 'South Australia',
'TAS' => 'Tasmania',
'ACT' => 'Australia Capital Territory',
'NT' => 'Northern Territory',
),
);
return $schemes;
}
add_filter( 'wpforms_address_schemes', 'wpf_dev_new_address_scheme', 10, 1 );
Using Your New Address Scheme
Once you’ve added the code, create a new form or edit an existing one. Add an Address field to your form, then look for the Scheme dropdown in the field settings. Your new address scheme will appear as an option there.
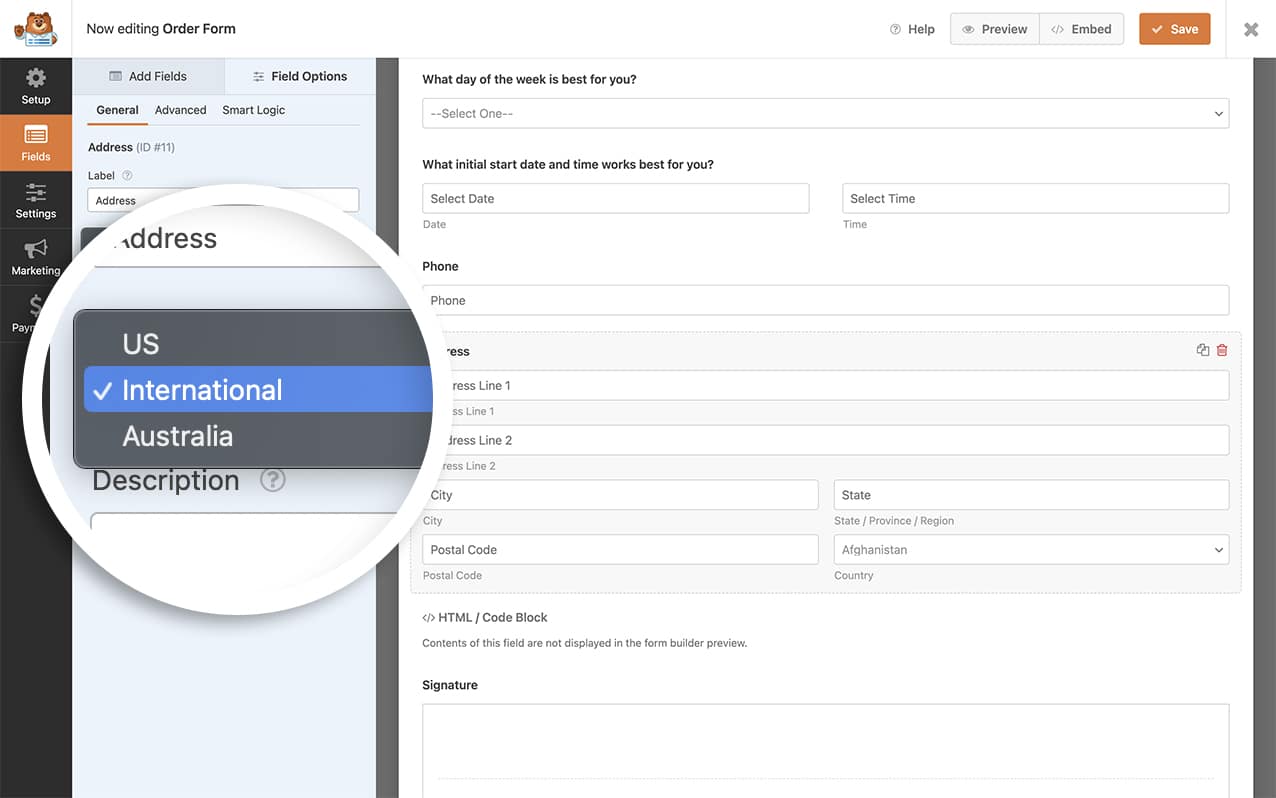
With either (or both) of the snippets above, you’ve now added new additional address schemes. Would you like to learn how to sublabels on the address field? Try out our article on How to Change the Address Field Sublabels.
Frequently Asked Questions
Q: Can I create a custom address scheme and list all the countries?
A: Absolutely! To create your own unique address scheme and list all the countries, you’d use the following code snippet. You would just customize this to your needs.
Q: How can I hide specific fields in the address schemes?
A: For US and International schemes, you can hide Address Line 2, Zip/Postal, and Country fields using the Advanced tab in the form builder.
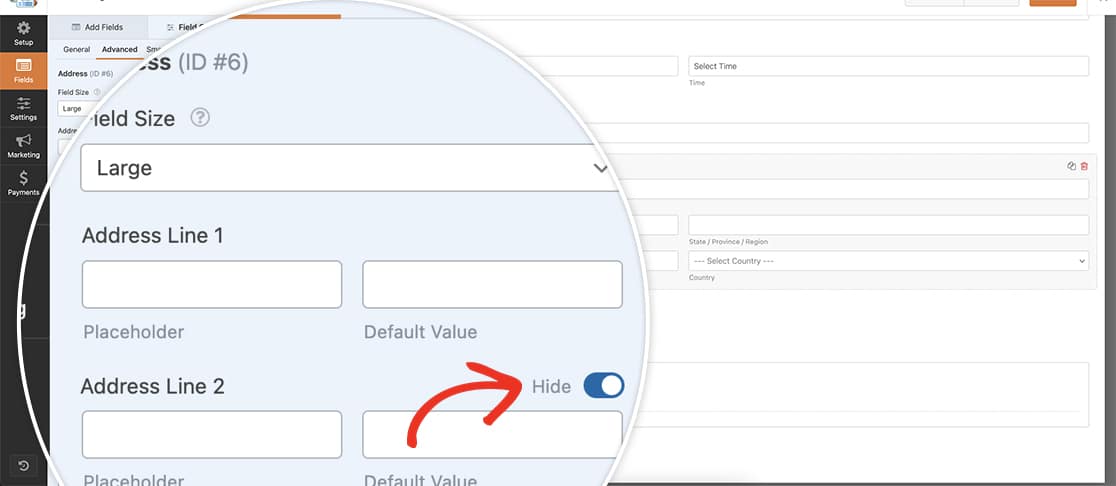
If you need to hide other fields, you can use CSS targeting your specific form and field IDs. For example:
input#wpforms-41-field_6-state,
label[for="wpforms-41-field_6-state"] {
visibility: hidden;
}
The example snippet shows that we’re hiding the State field and label for the form ID 41 and the field ID (for the Address field) 6. You’ll need to update these IDs to match your own IDs. If you need any help in where to find these IDs, please take a look at this handy guide.
If the Address field is a required field, we do not recommend hiding any fields with CSS as the address field would fail validation.
That’s it! Now you know how to create additional schemes for the address field. Next, would you like to learn how to customize address field labels? Check out our guide on changing address field sublabels for more details.