Would you like to enhance your form labels with interactive tooltips or dynamic content using shortcodes? While WPForms doesn’t support shortcodes in labels by default, you can easily add this functionality using PHP.
This guide will show you how to display any shortcode inside your form field labels.
Installing Shortcodes Ultimate Plugin (optional)
If you don’t already have a shortcodes plugin, you can install Shortcodes Ultimate from the WordPress plugin repository. This step is optional if you already have another shortcodes plugin or are using built-in shortcodes from your theme.
For help installing plugins, you can review WPBeginner’s guide on how to install a plugin.
Creating Your Form
You can create a new form or edit an existing form. For any help needed on how to create a form, please review this documentation.
We won’t be needing the Label for the Phone form field since we’re going to use the tooltip text as our label, so on the Advanced tab of the form field select the Hide Label option and then click Save on the form.
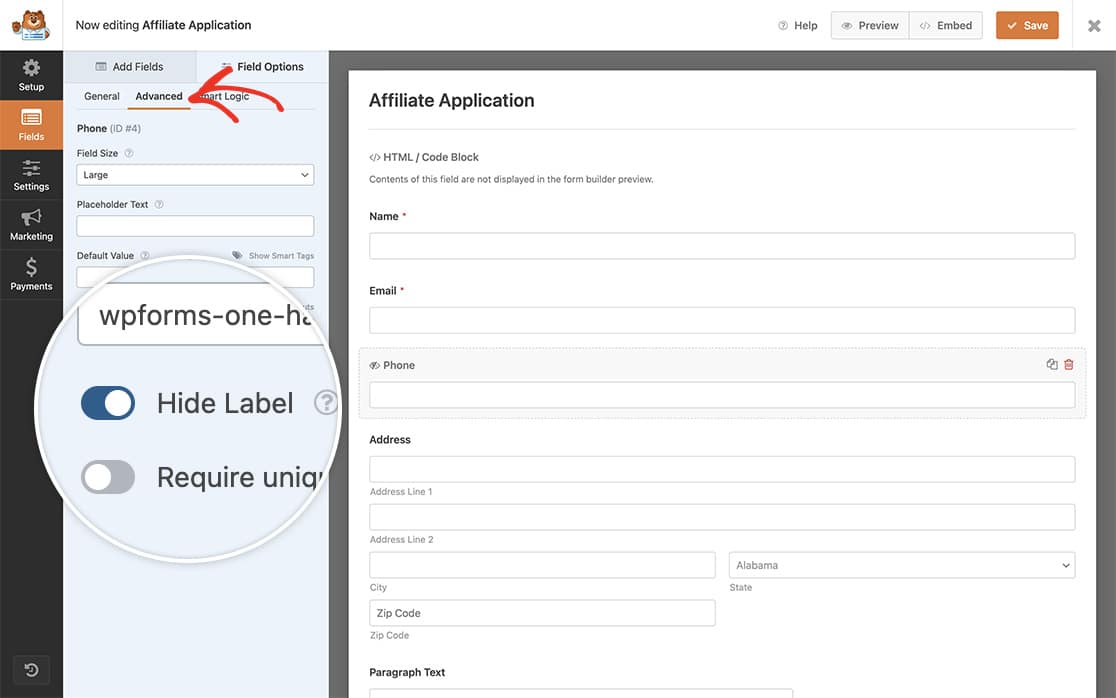
Adding the Code
To display shortcodes in your form labels, you’ll need to add a code snippet to your site. If you’re not sure how to add custom code, please review our guide on adding code snippets.
In the code snippet above, you’ll need to modify three key values:
- On line 10: Replace 1055 with your form ID
- On line 10: Replace 4 with your field ID
- On line 12: Replace the shortcode with your desired shortcode
When your visitors now see the form and click the tooltip, they’ll see your message.
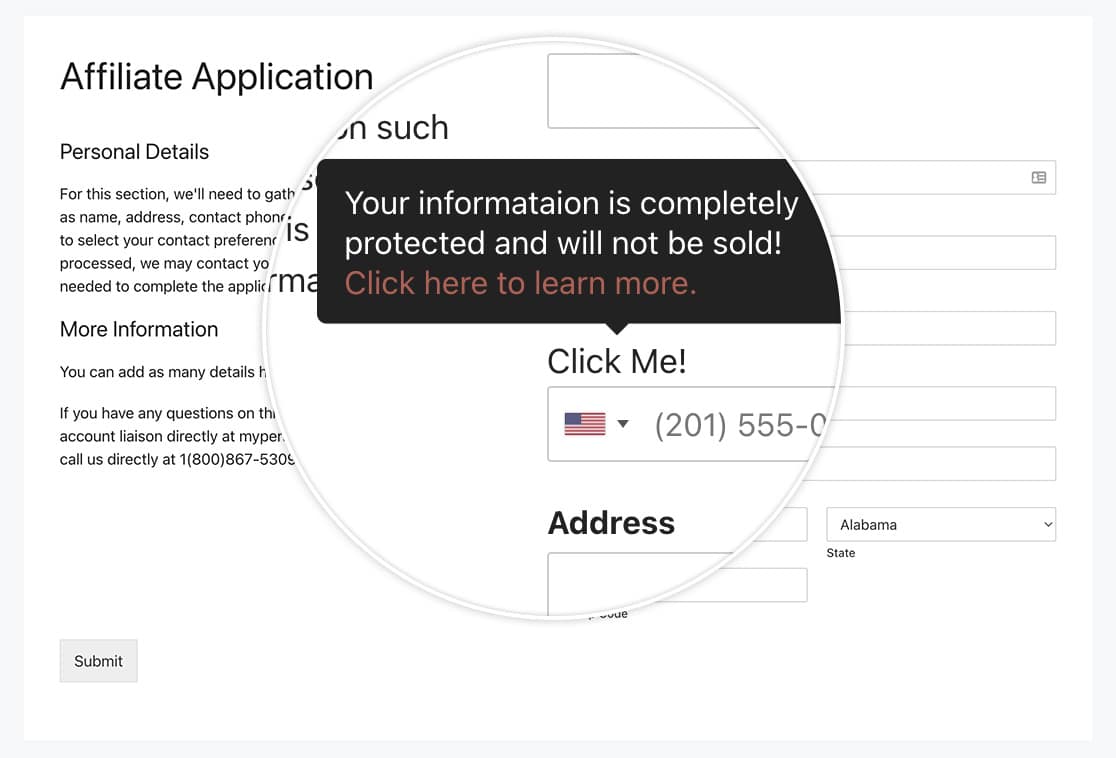
FAQ
Q: How do I add different shortcodes for different forms and labels?
A: If you’d like to re-use this snippet for other forms using different shortcodes, you certainly could do this.
/**
* Run shortcodes on the form label field.
*
* @link https://wpforms.com/developers/how-to-display-shortcodes-inside-the-label-of-the-form-field/
*/
function add_shortcode_to_label( $field, $form_data ) {
// Check that the form ID is 1055 and the field id is 4 for the Phone field
if ( 1055 === absint( $form_data[ 'id' ] ) && 4 === absint( $field[ 'id' ] ) ) {
echo do_shortcode( ' [su_tooltip text="Your information is completely protected and will not be sold!<br><a href=“https://myexamplesite.com/privacy/“>Click here to learn more.</a>" behavior="click" hide_delay="500"]Click Me![/su_tooltip] ' );
}
// Check that the form ID is 1055 and the field id is 2 for the Name field
if ( 1055 === absint( $form_data[ 'id' ] ) && 2 === absint( $field[ 'id' ] ) ) {
echo do_shortcode( ' [su_tooltip text="I am a different tooltip for a different field on the same form." behavior="click" hide_delay="500"]Click Me Too![/su_tooltip] ' );
}
// Check that the form ID is 1072 and the field id is 6 for the Comments field
if ( 1072 === absint( $form_data[ 'id' ] ) && 6 === absint( $field[ 'id' ] ) ) {
echo do_shortcode( ' [su_tooltip text="I am a different tooltip for a different field on a completely different form." behavior="click" hide_delay="500"]Click Me Too![/su_tooltip] ' );
}
}
add_action( 'wpforms_display_field_before', 'add_shortcode_to_label', 16, 2 );
And that’s all you need to display any shortcode inside a Label form field. Would you like to display shortcodes inside the HTML field? Take a look at our article on How to Display Shortcodes Inside the HTML Field.