Introduction
Would you like to capitalize form field inputs on your WPForms? You can easily use CSS and text-transform to format the input values, but the entry will be saved with exactly how it’s typed in. Using a small JavaScript snippet you can, in real-time, guarantee that these values are shown and stored with capitalization.
In this tutorial, we’ll walk you through each step on how to achieve this.
Creating the form
First, you’ll need to create your form. For the purpose of this tutorial, we’ve added a Paragraph Text and a Single Line Text form field to our form.
If you need any help in creating a form, please review this documentation.
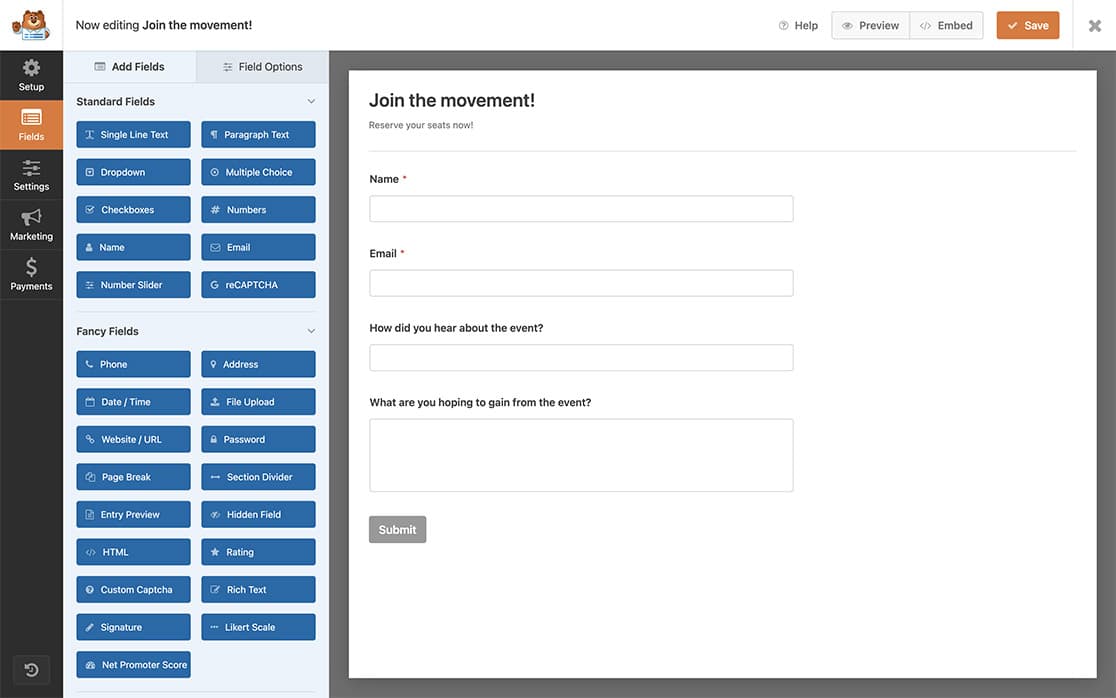
Adding the CSS class name
Next, we’re going to use a CSS class for each of these form fields to trigger the CSS to use the text-transform CSS rule that we’ll be adding in another step. To add the required CSS class, click on the Single Line Text field in your form builder and then click to open the Advanced.
Under the CSS Classes, add capitalize and repeat these steps for the Paragraph Text field as well.
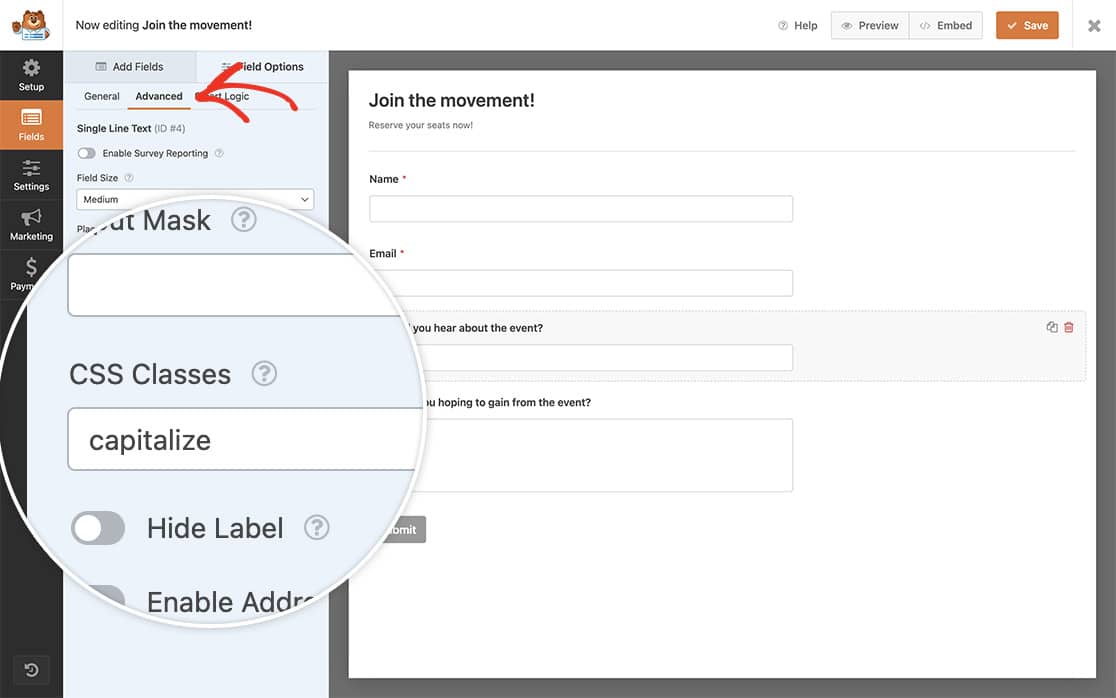
Implementation Options
Using only CSS (optional)
This custom CSS will mean that the form field will display uppercase, but the entry will still show the field values exactly as they are typed.
If you need help with how and where to add custom CSS, please review this tutorial.
Just copy and paste this CSS to your site.
.capitalize {
text-transform: capitalize;
}
Using only JavaScript (recommended)
To capitalize form field input values on the fly while the form is completed as well as make sure the entry information is stored with the capitalization, we’re going to add a small script that immediately capitalize the field as the text is being typed.
For help with adding JavaScript to your site, please take a look at this tutorial.
/*
* Capitalize form field text
*
* @link https://wpforms.com/developers/how-to-capitalize-form-field-inputs/
*/
function wpf_dev_capitalize() {
?>
<script type="text/javascript">
jQuery(document).ready(function() {
jQuery( '.wpforms-field.capitalize input' ).keyup(function() {
jQuery(this).val(jQuery(this).val().substr(0, 1).toUpperCase() + jQuery(this).val().substr(1).toLowerCase());
});
jQuery( '.wpforms-field.capitalize textarea' ).keyup(function() {
jQuery(this).val(jQuery(this).val().substr(0, 1).toUpperCase() + jQuery(this).val().substr(1).toLowerCase());
});
});
</script>
<?php
}
add_action( 'wpforms_wp_footer_end', 'wpf_dev_capitalize', 30 );
This snippet will look for any form field that has not only the default CSS class of wpforms-field but that also has the CSS class of capitalize so as the user is typing, the text will automatically be capitalized and when the form is submitted the entry will store those fields with the capital letters.
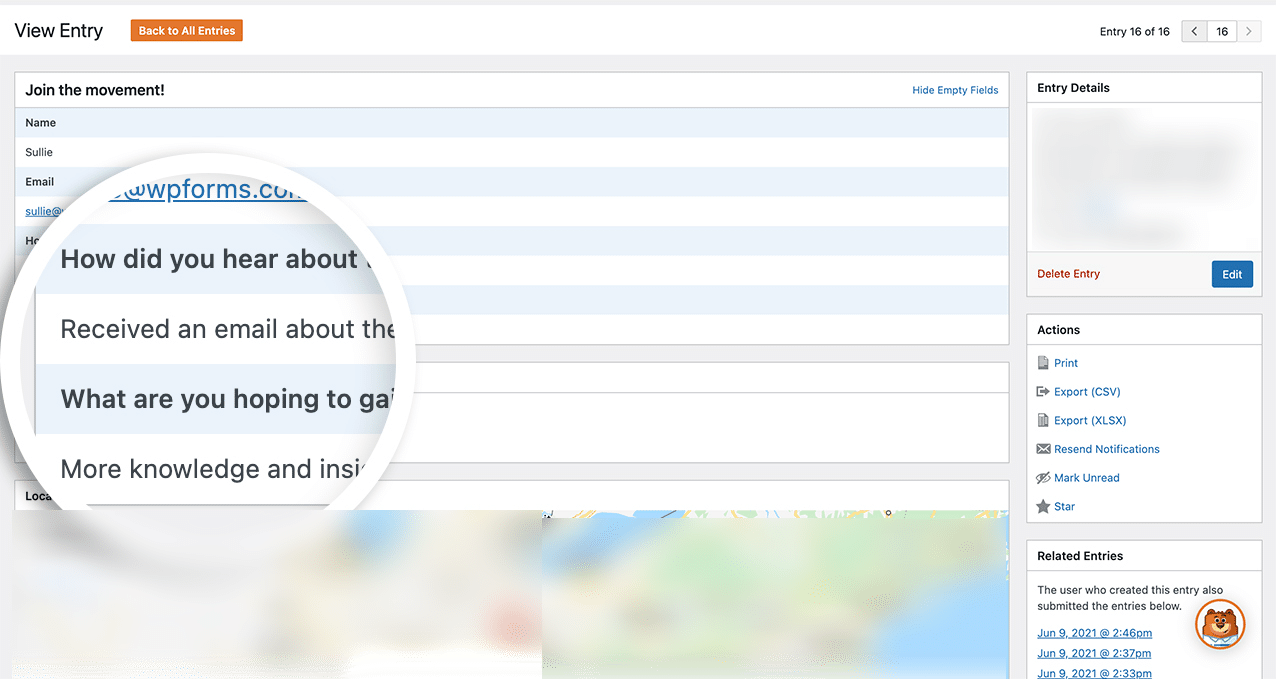
FAQ
What if I want to capitalize all text not just the first letter?
If you need the text to be fully uppercase (e.g., “hello” displays as “HELLO”), use text-transform: uppercase
instead of text-transform: capitalize
. For more details on the CSS properties for text transformation, you can refer to Mozilla’s documentation on text-transform.
And that’s all you need! Would you like to stop users from entering URLs into your form fields? Take a look at our article on How to Block URLs Inside the Form Fields.
Related
Action Reference: wpforms_wp_footer_end