Would you like to increment a count on each form submission? With a simple code snippet, you can add an auto-incrementing counter inside a Hidden field that increases with each new entry. This feature is particularly useful for tracking submissions sequentially or creating unique reference numbers.
This guide will show you how to set up an incremental counter that increases with each new entry.
Setting Up the Form
To begin, open the form builder by creating a new form or editing your existing one.
Then, for the counter to work, you’ll need to add a Hidden field – you can find this under the Fancy Fields section. Your users won’t see this field on the form, but it’ll store our counter value behind the scenes.
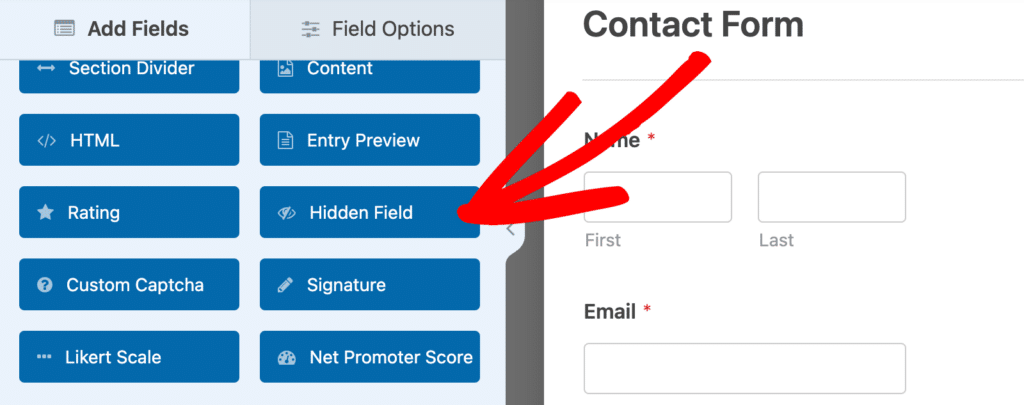
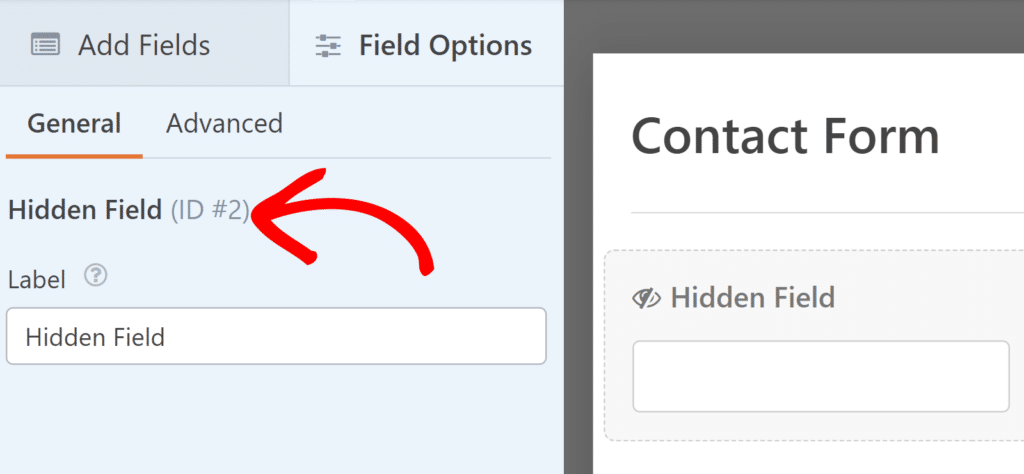
Adding the Code Snippet
The following code snippet will count your form entries and assign an incremental number to each new submission.
The snippet works by first checking if the submitted form matches your specified form ID. When it finds a match, it counts all existing entries for that form and adds 1 to create the new entry number.
This number then gets padded with leading zeros and stored in your Hidden field. The process happens automatically each time someone submits your form.
Customizing the Counter
You’ll need to customize three key values in the code snippet for your specific form:
- On line 9, you’ll need to change
1000
to your own form ID. - On line 16, replace
15
to match your Hidden Field’s ID. - On line 19, you’ll see
3
which sets how many digits appear in your numbers. The code adds zeros at the start to make all numbers the same length. For instance, with 3 digits, your numbers would look like: 001, 002, 003, and so on.
Testing Your Counter
Once you’ve added and customized the code snippet, it’s time to make sure everything works correctly. Submit a test entry through your form and check the entry in WPForms » Entries. You should see your incremental number stored in the Hidden field. Submit another test entry to confirm the number increases as expected.
Frequently Asked Questions
Q: Can I assign a prefix to this number?
A: Absolutely! Here’s an example of adding some letters in front of the count. We’re going to add order- in front of our count. You can do this by modifying line 23 in the code snippet.
$fields[$my_field_id]['value'] = 'ORDER-' . zeroise($new_total_entries, $min_digits);
This will display as ORDER-001, ORDER002
, and so on. You can change ‘ORDER
‘ to any prefix you want, like ‘REF
‘, ‘Ticket
‘, or whatever suits your needs.
That’s it! You now have a form that automatically assigns an incremental number to each new submission.
Would you like to assign a unique ID number on each form submission? Check out our guide on creating unique IDs for form entries.