Description
The deleteuploadedfiles
Class is designed to delete uploaded files subsequent to email notifications. This functionality is particularly useful when entry storage and saving to the Media Library are disabled.
WPForms strongly discourages modifying any parent theme files directly. Any alterations made directly to the parent theme are at risk of being overwritten during theme updates, resulting in the loss of customizations. It is advised to create and use a child theme for making any desired changes.
Methods
- Establish dependencies to support the Class by generating a file named
class-delete-uploaded-files.php
within the root directory of your theme. - After creating this file, open your
functions.php
and insert the following snippet. Save the changes to your functions file.
require __DIR__ . '/class-delete-uploaded-files.php';
( new \WPF\DeleteUploadedFiles() )->hooks();
Source
File: class-delete-uploaded-files.php
Usage
To utilize this Class, there are certain setup requirements.
Entries are disabled
To disable storing the entries, select Settings » General. Confirm the Disable storing entry information in WordPress is enabled.
Disable storing uploads to Media Library
Next, be sure the File Upload field has the Store file in WordPress Media Library disabled by clicking on the field and on the Advanced tab, be sure the setting is disabled.
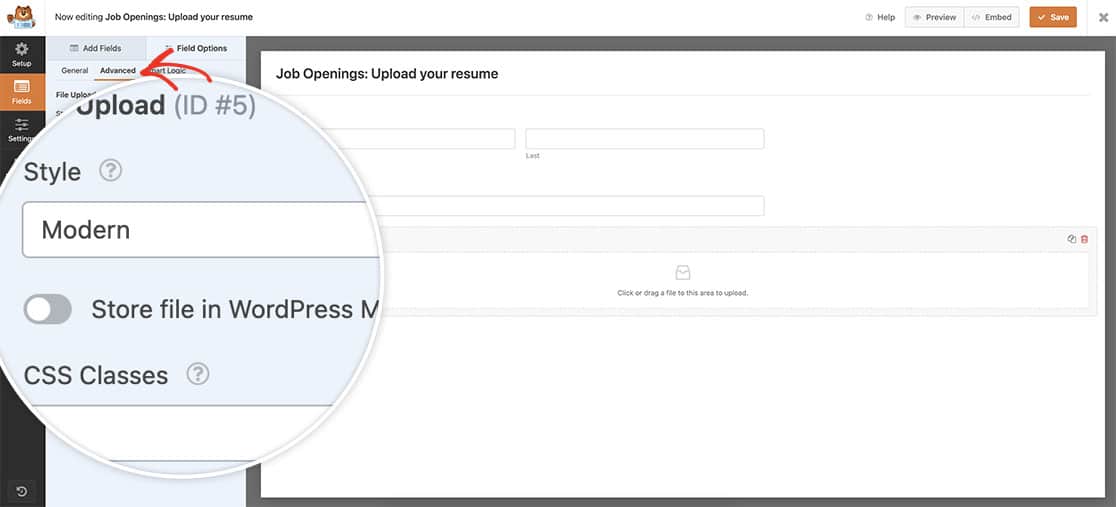
Attach file to email notifications
Finally, the last step is to be sure the file attachements are enabled to the email notification. To complete this step, navigate to Settings » Notifications and under the Advanced options, enable the Enable File Upload Attachments. From the File Upload Fields dropdown select your upload field.
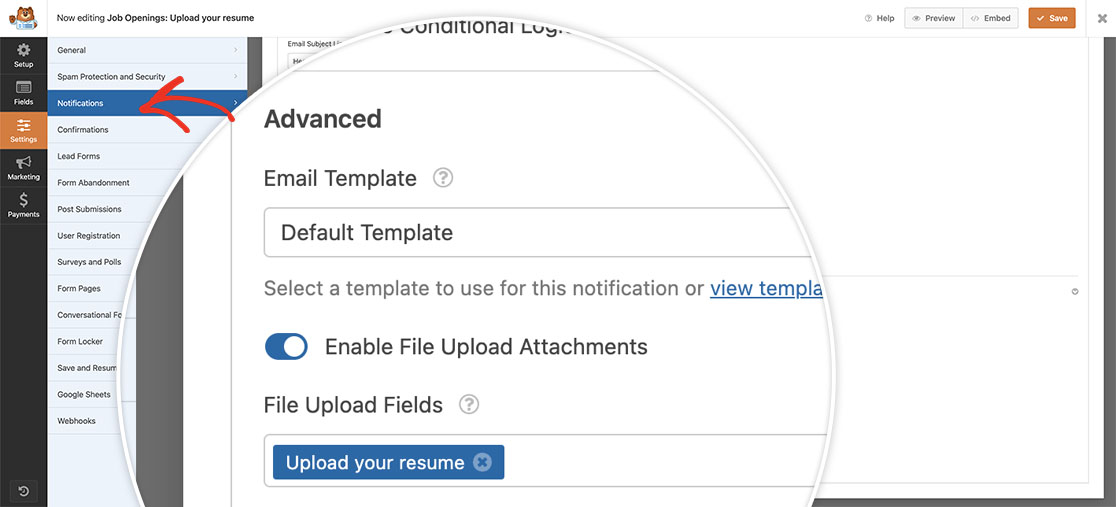
Once these settings are completed, any file upload on your forms will attach the files uploaded but not store them on your server.
Additional (optional)
Moreover, this Class permits the removal of uploaded files from the WP Media Library. This feature is turned off by default since certain users might prefer to retain uploaded files in the WP Media, especially when utilizing Post Submissions addon and requiring file storage for thumbnails. Nevertheless, if users wish to delete both uploaded files and associated files from the WP Media Library, please must modify the DELETE_MEDIA_FILES
constant in the class-delete-uploaded-files.php
file to true
.