Would you like to store field values for the Dropdown, Checkboxes, and Multiple Choice fields inside the WPForms entry? In this tutorial, we’ll show you how to use PHP to take the field value instead of the field label and store it inside the entry.
Storing the value inside the entry
For this tutorial, we’re going to actually add the snippets to our site first before creating the form. We do this simply because part of this snippet is to enable the option on the fields for Show Values for the Dropdown, Checkboxes, and Multiple Choice fields.
For assistance with how and where to add snippets, please check out this tutorial.
We’ve added two separate snippets. The first snippet is following this tutorial that will allow us to add field values for the Dropdown, Checkboxes, and Multiple Choice fields.
The second snippet takes the form submission and while processing it will grab the field value instead of the field label to store on the entry.
Important: If you’re using conditional logic in notifications that relies on the Dropdown field, this snippet may cause those rules to stop working.
This is because notification conditions compare the choice label, while this snippet stores and uses the choice value instead. Since labels and values are different, they won’t match and the condition will not be triggered.
Creating the form
Now it’s time to create your form and add your fields which will include at least one Dropdown, Checkboxes, or Multiple Choice.
If you need any help in creating forms, please review our detailed documentation.
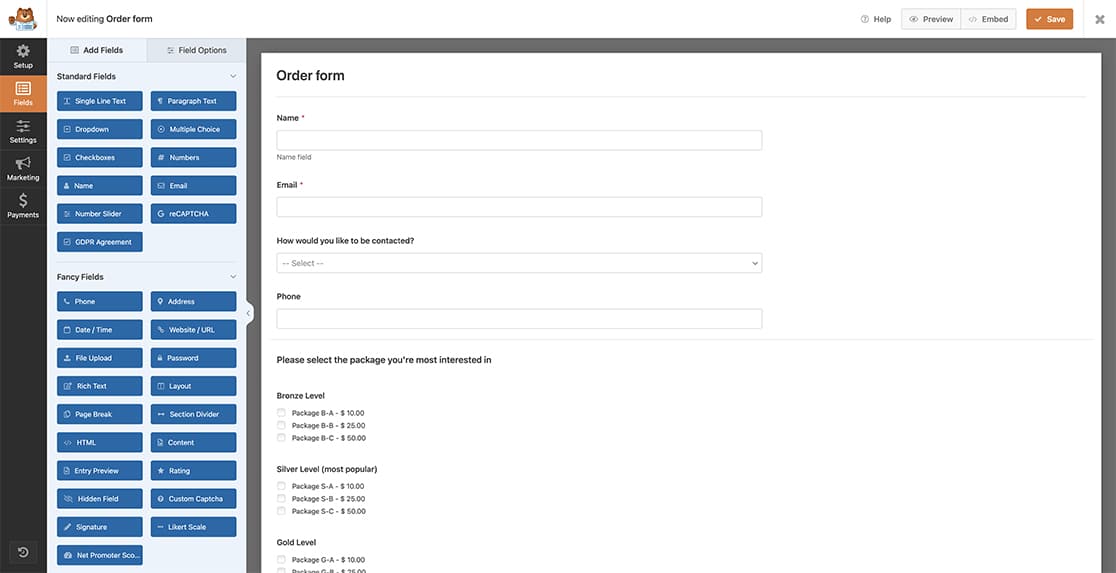
For the purpose of this documentation, we added a Dropdown field for the contact preference. In order to add the field values, just select the field and click the Advanced tab.
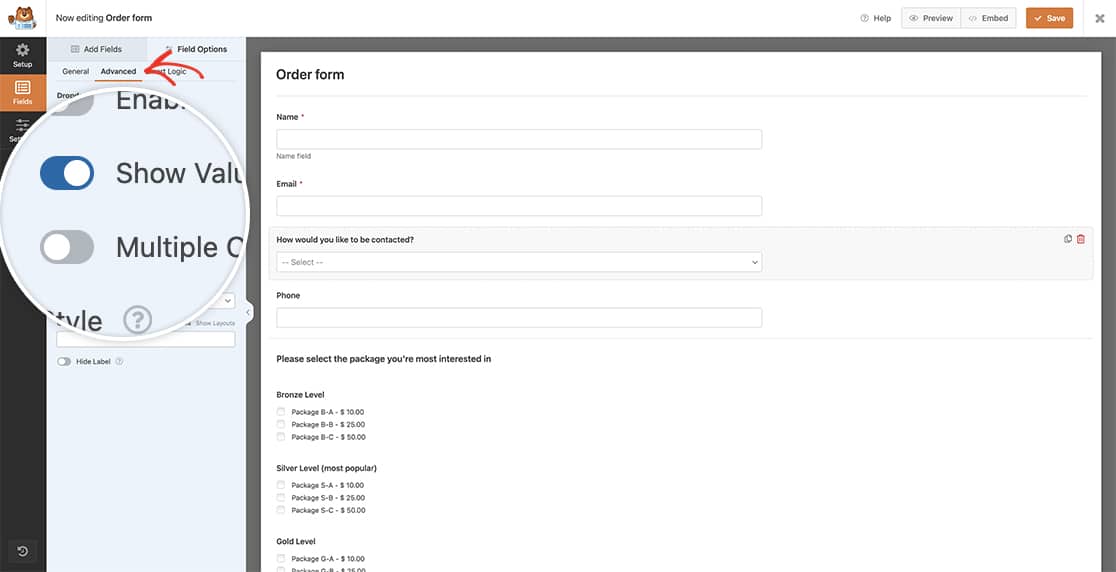
Click the button to toggle on the Show Values and then you click back to the General tab to add your field values.
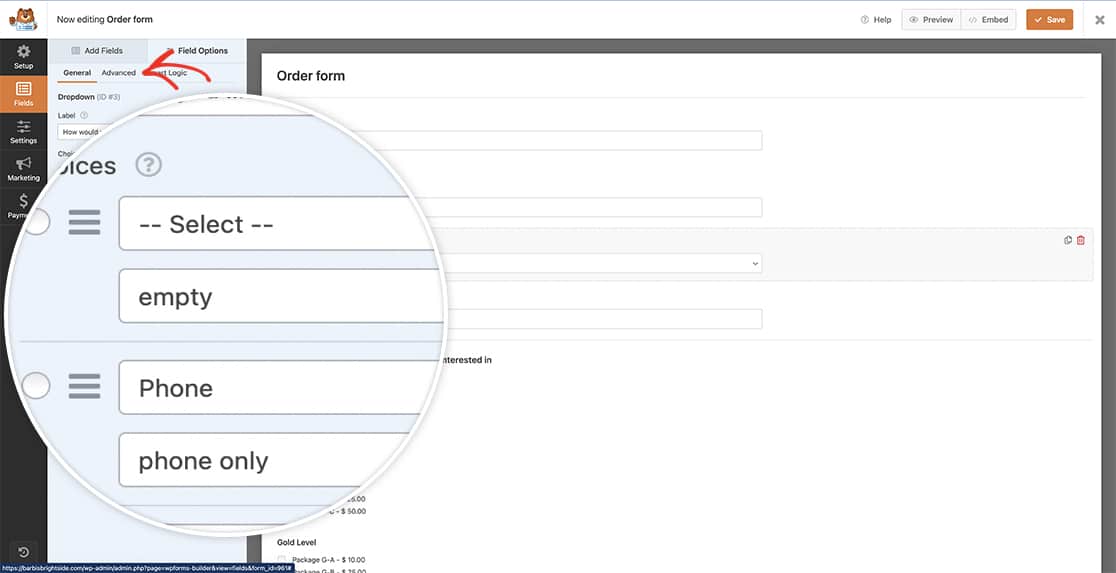
Once you’ve saved the form, you can now see that the field values are stored inside the form entry rather than the field label.
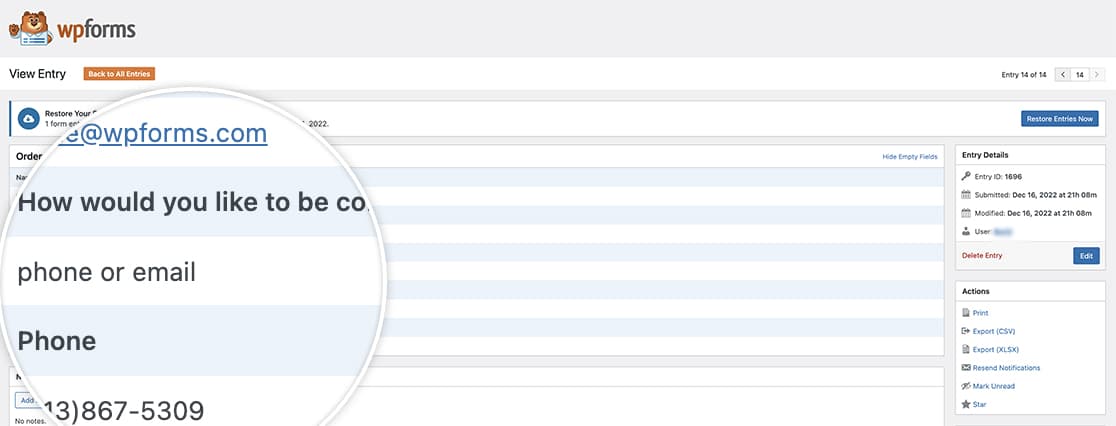
Would you like to also send the field values through using the Zapier addon? Take a look at our tutorial on How to Send Field Values to Excel Using Zapier.
Reference Filter
FAQ
Q: How can I target just a single form?
A: To target only 1 form, just use this snippet instead. You’ll need to update the 584 to match your own form ID. To find your form ID, please review this tutorial for assistance.
/**
* Show values in Dropdown, checkboxes, and Multiple Choice.
*
* @link https://wpforms.com/developers/add-field-values-for-dropdown-checkboxes-and-multiple-choice-fields/
*/
add_action( 'wpforms_fields_show_options_setting', '__return_true' );
/**
* Save choices 'values' instead of 'labels' for the fields with 'Show values' option enabled.
*
* @link https://wpforms.com/developers/how-to-store-field-values-in-the-wpforms-entry/
*/
function wpf_dev_process_filter_choices_values( $fields, $entry, $form_data ) {
// Optional, you can limit to specific forms. Below, we restrict output to
// form #584.
if ( absint( $form_data[ 'id' ] ) !== 584 ) {
return $fields;
}
if ( ! is_array( $fields ) ) {
return $fields;
}
foreach ( $fields as $field_id => $field ) {
if (
isset( $field[ 'type' ] ) &&
in_array( $field[ 'type' ], [ 'checkbox', 'radio', 'select' ], true ) &&
! empty( $form_data[ 'fields' ][ $field_id ][ 'show_values' ] )
) {
$value_raw = ! empty( $field[ 'value_raw' ] ) ? $field[ 'value_raw' ] : '';
$field[ 'value_raw' ] = $field[ 'value' ];
$field[ 'value' ] = $value_raw;
$fields[ $field_id ] = $field;
}
}
return $fields;
};
add_filter( 'wpforms_process_filter', 'wpf_dev_process_filter_choices_values', 10, 3 );