Volete visualizzare le voci di un modulo in una pagina del vostro sito per mostrare pubblicamente agli utenti gli invii di moduli completati? Questo tutorial vi spiega come creare e implementare questa funzionalità sul vostro sito WordPress.
Per impostazione predefinita, solo gli utenti professionisti possono visualizzare le voci dei moduli dall'amministrazione di WordPress, in base ai controlli di accesso assegnati al loro utente. Tuttavia, è possibile creare facilmente la funzionalità per visualizzare le voci dei moduli nel frontend del sito.
Creazione dello shortcode
Per prima cosa, vi forniremo uno snippet di codice che dovrà essere aggiunto al vostro sito, in modo che possiate facilmente riutilizzare questo stesso shortcode, con l'eccezione dell'aggiornamento dell'ID del modulo in base alle vostre esigenze.
È sufficiente aggiungere il seguente frammento di codice al vostro sito. Se non siete sicuri di come fare, consultate questo tutorial.
/**
* Custom shortcode to display WPForms form entries in table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exists, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<table class="wpforms-frontend-entries">';
echo '<thead><tr>';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<th>';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</th>';
}
echo '</tr></thead>';
echo '<tbody>';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<tr>';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<td>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</td>';
}
echo '</tr>';
}
echo '</tbody>';
echo '</table>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Questo snippet di codice consente di utilizzare questo shortcode in qualsiasi punto del sito che accetti shortcode, come pagine, post e aree widget.
Utilizzando semplicemente questo [wpforms_entries_table id="FORMID"]
i visitatori vedranno le voci inviate per il modulo specificato in id="FORMID".
Il numero ID di un modulo può essere trovato andando su WPForms " Tutti i moduli e guardando il numero nella colonna Shortcode per ogni modulo.
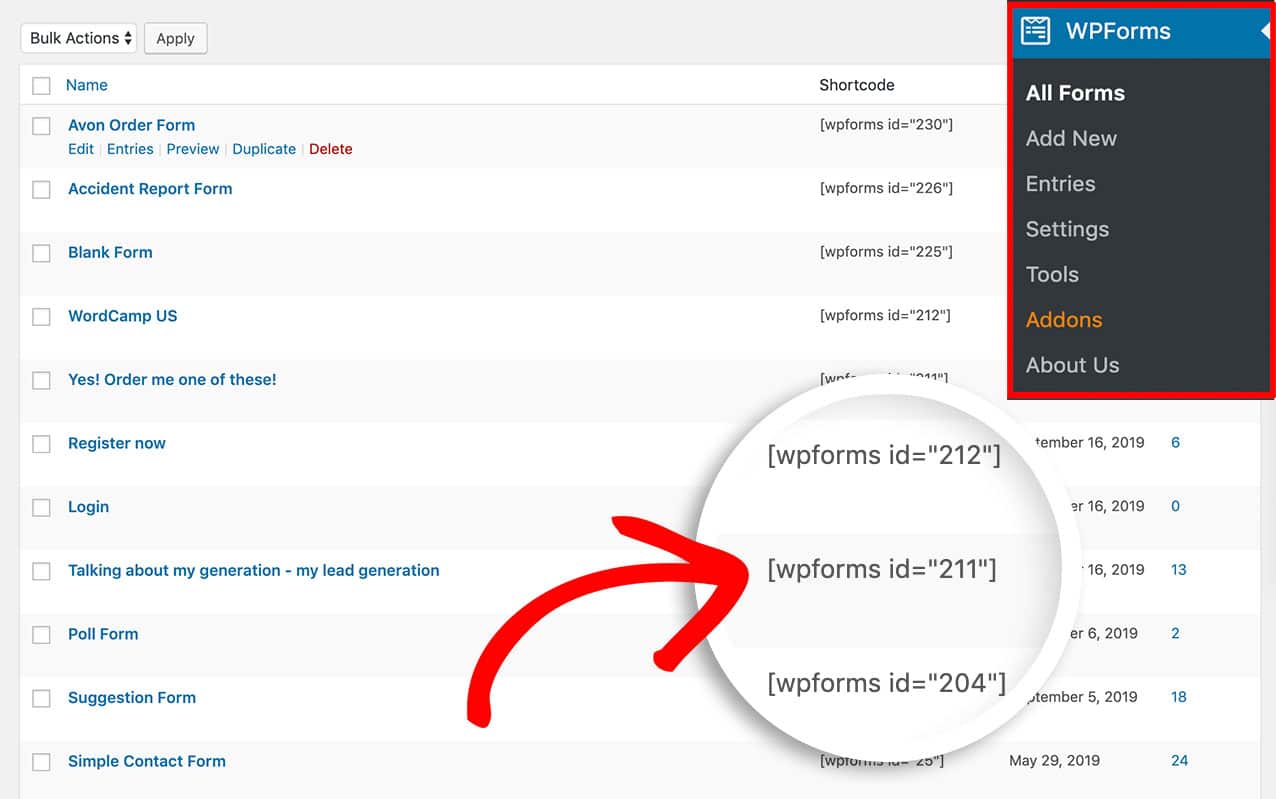
Visualizzazione delle voci del modulo
Dopo aver creato lo shortcode, è necessario aggiungerlo a una pagina o a un post del sito per visualizzare le voci.
Per prima cosa, è necessario determinare l'ID del modulo su cui si desidera visualizzare le voci. Nel nostro esempio, visualizzeremo le voci per l'ID 211 del modulo, per cui il nostro shortcode si presenta così.
[wpforms_entries_table id="211"]
L'unico attributo richiesto per utilizzare lo shortcode è l'id; senza definirlo nello shortcode, non verrà visualizzato nulla, quindi l'ID del modulo deve essere indicato all'interno dello shortcode.
Tuttavia, è possibile filtrare ulteriormente le voci definendo alcuni attributi dello shortcode; esamineremo ogni attributo disponibile.
Definizione dell'attributo tipo
L'attributo type può essere utilizzato se si vuole mostrare solo le voci con le stelle. Ecco un esempio.
[wpforms_entries_table id="211" type="starred"]
Di seguito sono riportati altri parametri di tipo disponibili che possono essere utilizzati:
- all - utilizzando questo attributo si visualizzano tutti i tipi di voce.
- read - questo attributo visualizza solo le voci che sono state visualizzate da un amministratore.
- non letto: utilizzando questo attributo, si vedranno solo le voci che non sono state aperte da un amministratore del sito.
- stellato - questo attributo visualizza solo le voci che sono state stellate da un amministratore
Utilizzo dell'attributo utente
Se un utente è attualmente collegato al vostro sito e volete che veda solo le voci del modulo che ha inviato, utilizzate questo shortcode.
[wpforms_entries_table id="FORMID" user="current"]
Se l'utente non è loggato, verranno visualizzate tutte le voci di questo modulo.
Se l'utente è connesso ma non ha completato il modulo (mentre è connesso), la pagina visualizzerà Nessun risultato trovato.
Definizione dell'attributo Campi
Per visualizzare solo alcuni campi, occorre innanzitutto individuare gli ID dei campi che si desidera visualizzare. Per trovare gli ID dei campi, consultare questo tutorial.
Una volta conosciuti gli ID dei campi, si utilizzerà questo come shortcode. In questo esempio, vogliamo solo il campo Nome, che è l'ID del campo 0, e il campo Commenti, che è l'ID del campo 2.
[wpforms_entries_table id="211" fields="0,2"]
Nota: gli ID di campi multipli sono separati da una virgola nel codice breve.
Utilizzo dell'attributo numero
Per impostazione predefinita, lo shortcode visualizza solo le prime 30 voci. Tuttavia, se si desidera visualizzare un numero inferiore di voci, è necessario utilizzare l'opzione number
parametro. Ad esempio, se si desidera mostrare le prime 20 voci, aggiungere lo shortcode in questo modo.
[wpforms_entries_table id="211" number="20"]
La variabile number="20″ passata all'interno dello shortcode determinerà il numero di voci da visualizzare nella tabella. Se si desidera mostrare tutte le voci, cambiare il numero in 9999.
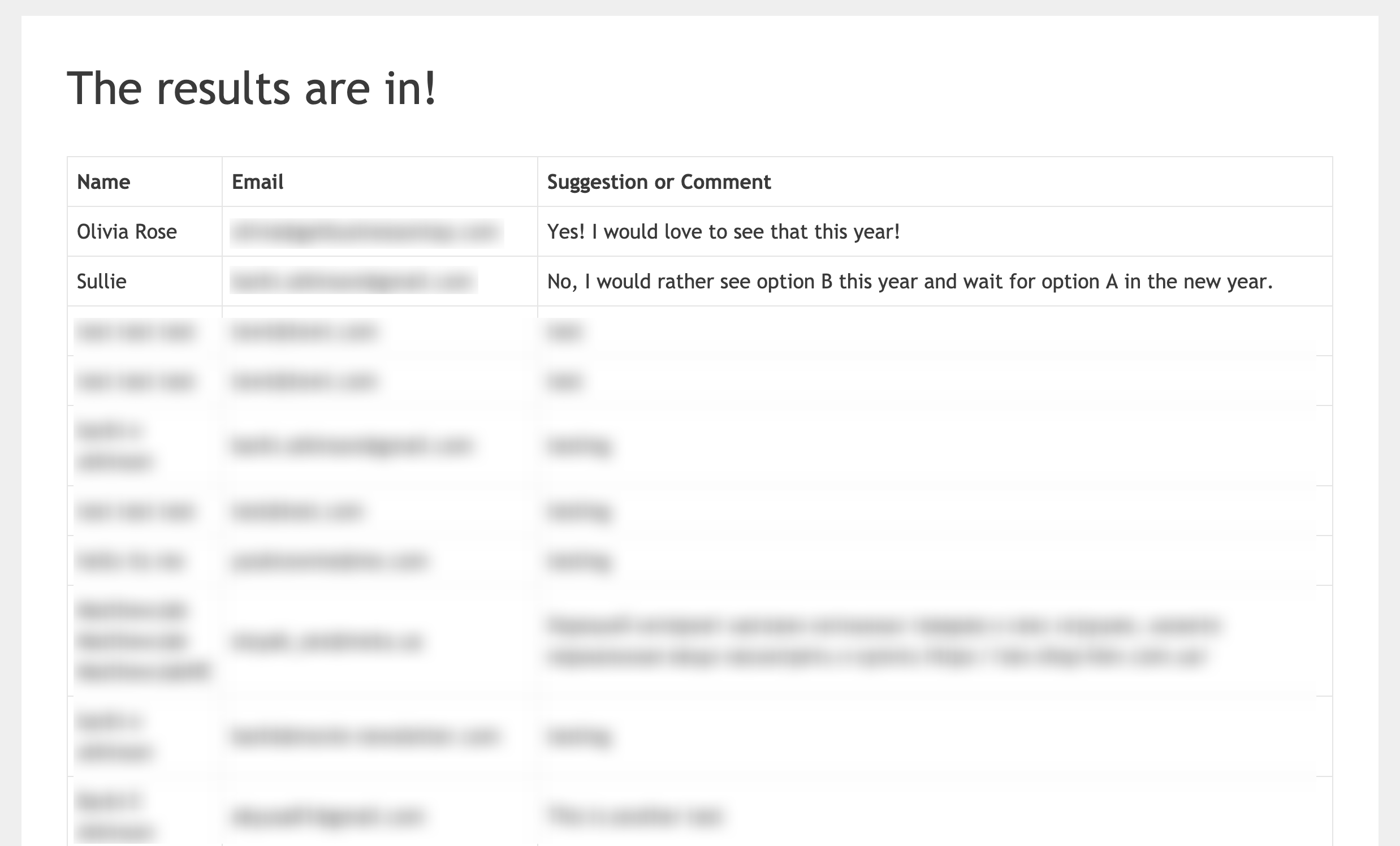
Utilizzo dell'attributo sort e order
Quando si utilizza lo shortcode, è possibile indicare allo shortcode il campo in base al quale si desidera ordinare la tabella e quindi definire se si desidera che sia ordinata con asc (ordine crescente) o desc (ordine decrescente). Un esempio di shortcode potrebbe essere il seguente.
[wpforms_entries_table id="211" sort="1" order="asc"]
In questo esempio, visualizzeremo una tabella di voci per l'ID 211 del modulo e ordineremo questa tabella in ordine asc (crescente) usando l'ID 1 del campo, che è l'ID del campo Nome del modulo.
Stilizzare il tavolo (facoltativo)
Lo stile della tabella delle voci è del tutto facoltativo, poiché la maggior parte dei temi ha uno stile predefinito per le tabelle, così come i browser. Tuttavia, abbiamo voluto iniziare con un esempio, quindi se volete cambiare lo stile predefinito della tabella, dovrete copiare e incollare questo CSS sul vostro sito.
Per qualsiasi assistenza su come e dove aggiungere i CSS al vostro sito, consultate questo tutorial.
Lo stile della tabella d'ingresso varia a seconda dello stile predefinito che il tema può avere o meno per le tabelle.
table {
border-collapse: collapse;
}
thead tr {
height: 60px;
}
table, th, td {
border: 1px solid #000000;
}
td {
white-space: normal;
max-width: 33%;
width: 33%;
word-break: break-all;
height: 60px;
padding: 10px;
}
tr:nth-child(even) {
background: #ccc
}
tr:nth-child(odd) {
background: #fff
}
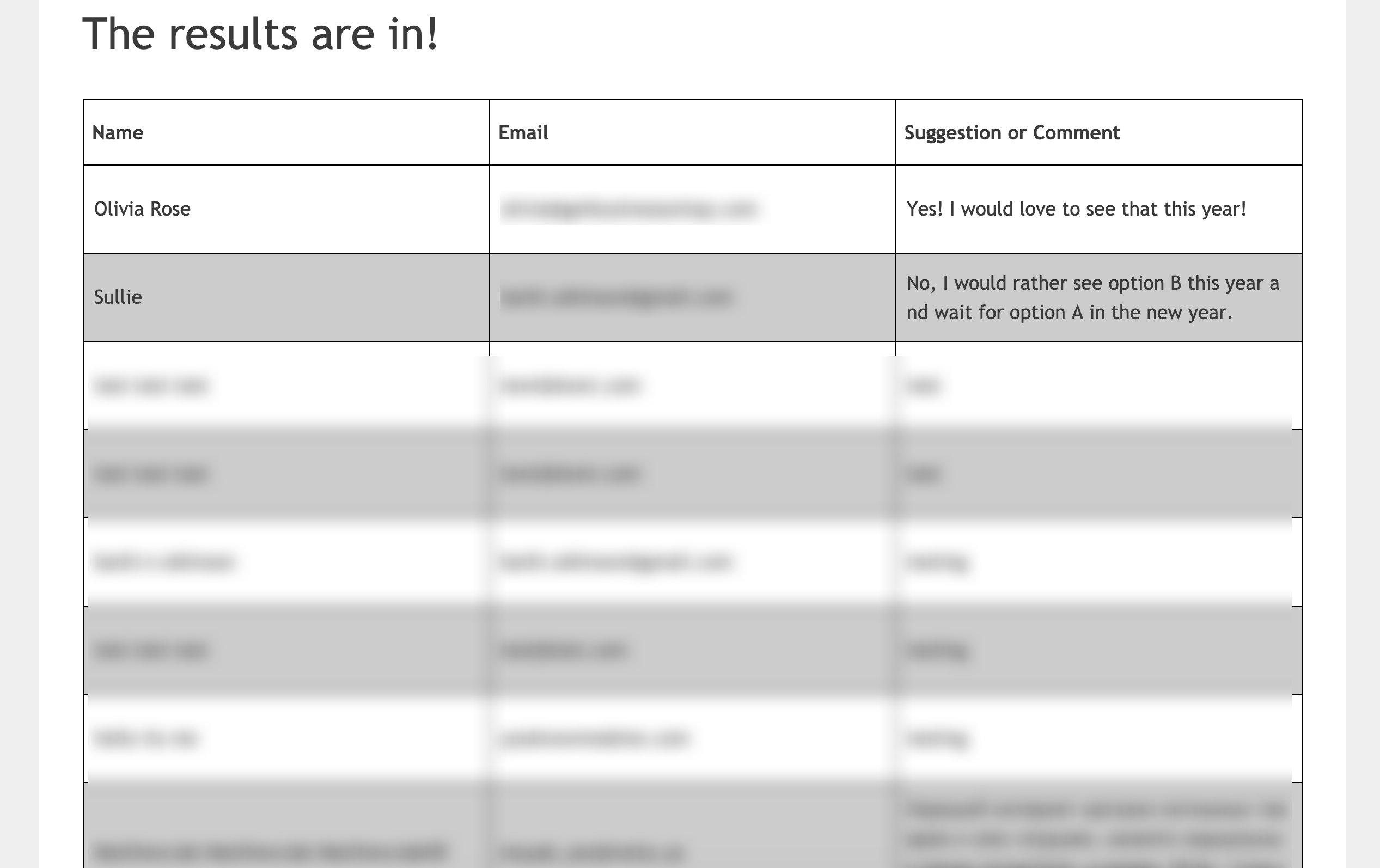
Aggiunta di una vista non tabellare
Se si preferisce non utilizzare una visualizzazione a tabella HTML per visualizzare le voci, abbiamo una soluzione alternativa anche per voi. Invece di creare il vostro shortcode con lo snippet di codice qui sopra, utilizzate questo snippet di codice.
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="header-row">';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<span class="column-label">';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</span>';
}
echo '</div>';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Tuttavia, è importante notare che, poiché non stiamo usando una tabella standard, non ci sarà alcuno stile predefinito quando si usa questa opzione; quindi il CSS nel prossimo passo è fondamentale, ma elencheremo le classi CSS usate in modo che possiate applicare il vostro stile a questa opzione.
Stile del non-tavolo
Di seguito è riportato un elenco dettagliato delle classi CSS utilizzate e del loro scopo (se necessario).
- .wrapper - si tratta di un involucro complessivo attorno all'intero blocco di voci e può essere utilizzato se si desidera ridurre la larghezza del blocco in modo che non si estenda al 100% della pagina.
- .header-row - si tratta di un altro involucro complessivo intorno alle etichette di ogni colonna (solo le etichette) se si desidera cambiare il colore di sfondo della sezione delle etichette.
- .column-label - questo wrapper avvolge ogni singolo titolo di etichetta. Ad esempio, Nome, Indirizzo e-mail e Suggerimento/Commento sono circondati da uno di questi wrapper e possono essere utilizzati per definire la dimensione del testo.
- .entries - si tratta di un involucro generale intorno alle voci stesse, se si desidera modificare il colore di sfondo di questa sezione per farlo apparire in modo diverso rispetto alle etichette.
- .entry-details - si tratta di un involucro complessivo attorno a ogni singola voce e può essere utile se si desidera fornire uno sfondo di colore diverso per ogni riga di voce.
- .details - ogni colonna di informazioni è racchiusa in questa classe, in modo da poter aggiungere qualsiasi altro stile desiderato, come la dimensione dei caratteri, il colore, ecc.
Si può usare il CSS qui sotto per aggiungere uno stile predefinito per la vista non tabellare.
Per qualsiasi assistenza su come e dove aggiungere i CSS al vostro sito, consultate questo tutorial.
.wrapper {
width: 100%;
clear: both;
display: block;
}
.header-row {
float: left;
width: 100%;
margin-bottom: 20px;
border-top: 1px solid #eee;
padding: 10px 0;
border-bottom: 1px solid #eee;
}
span.details, span.column-label {
display: inline-block;
float: left;
width: 33%;
margin-right: 2px;
text-align: center;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.header-row span.column-label {
text-transform: uppercase;
letter-spacing: 2px;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
float: left;
margin: 20px 0;
padding-bottom: 20px;
}
Nota: il CSS fornito sopra si basa sulla visualizzazione di soli 3 campi della voce, ovvero il campo Nome, Email e Commenti. Se si desidera visualizzare altri campi, ricordarsi di aggiornare il CSS di conseguenza.
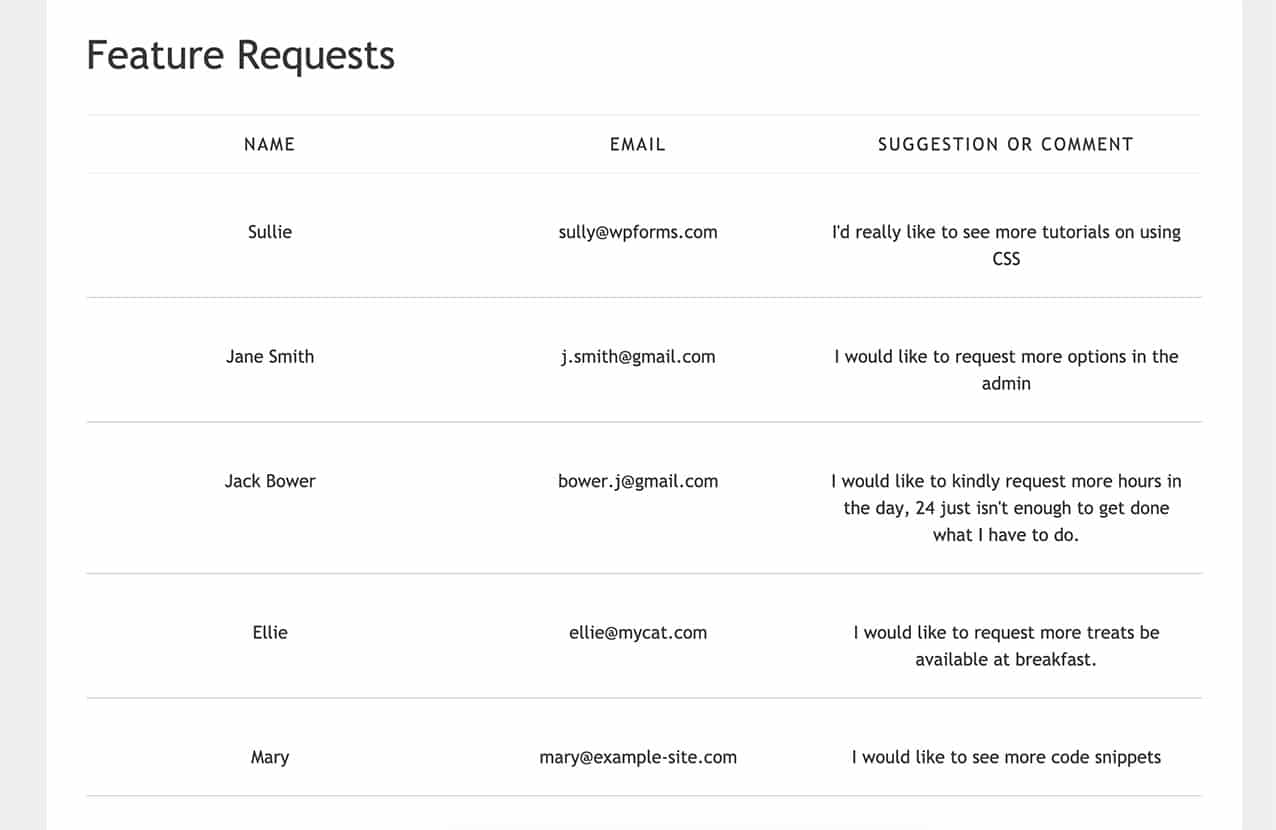
Ecco fatto, ora avete creato uno shortcode che può essere aggiunto in vari punti del vostro sito, in modo da poter visualizzare le voci del modulo agli utenti non amministratori. Volete anche visualizzare quante voci sono rimaste nel vostro modulo quando usate l'addon Form Locker? Provate il nostro frammento di codice su Come visualizzare il numero di iscrizioni rimanenti.
Filtro di riferimento
FAQ
Queste sono le risposte ad alcune delle domande più frequenti sulla visualizzazione dei moduli in una pagina o in un post di WordPress.
D: Come posso visualizzare solo il conteggio delle voci non lette?
R: Dovete aggiungere questo snippet al vostro sito.
<?php
/**
* Custom shortcode to display WPForms form entries count for a form.
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
*/
function wpf_dev_entries_count( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'type' => 'all', // all, unread, read, or starred.
],
$atts
);
if ( empty( $atts['id'] ) ) {
return;
}
$args = [
'form_id' => absint( $atts['id'] ),
];
if ( $atts['type'] === 'unread' ) {
$args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$args['starred'] = '1';
}
return wpforms()->entry->get_entries( $args, true );
}
add_shortcode( 'wpf_entries_count', 'wpf_dev_entries_count' );
Quindi siete liberi di utilizzare questo shortcode in qualsiasi punto del vostro sito WordPress in cui siano accettati gli shortcode.
[wpf_entries_count id="13" type="unread"]
È sufficiente cambiare il tipo di documento in non letto, letto o con le stelle.
D: Perché le mie voci più recenti non vengono visualizzate?
R: Il problema è probabilmente dovuto alla cache a livello di server, sito o browser. Verificare che la cache sia completamente libera.
D: Devo avere per forza delle colonne?
R: No, affatto. È possibile visualizzare le voci posizionando l'etichetta davanti al valore del campo e disponendo ogni campo su una riga separata utilizzando questo snippet e il CSS.
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts['id'] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts['id'] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts['fields'] ) && $atts['fields'] !== '' ? explode( ',', str_replace( ' ', '', $atts['fields'] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data['fields'];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data['fields'][$field_id] ) ) {
$form_fields[$field_id] = $form_data['fields'][$field_id];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field['type'], $form_fields_disallow, true ) ) {
unset( $form_fields[$field_id] );
}
}
$entries_args = [
'form_id' => absint( $atts['id'] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts['user'] ) ) {
if ( $atts['user'] === 'current' && is_user_logged_in() ) {
$entries_args['user_id'] = get_current_user_id();
} else {
$entries_args['user_id'] = absint( $atts['user'] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts['number'] ) ) {
$entries_args['number'] = absint( $atts['number'] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts['type'] === 'unread' ) {
$entries_args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$entries_args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$entries_args['starred'] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key]['fields'] = json_decode($entry['fields'], true);
$entries[$key]['meta'] = json_decode($entry['meta'], true);
}
if ( !empty($atts['sort']) && isset($entries[0]['fields'][$atts['sort']] ) ) {
if ( strtolower($atts['order']) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1['fields'][$atts['sort']]['value'], $entry2['fields'][$atts['sort']]['value']);
});
} elseif ( strtolower($atts['order']) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2['fields'][$atts['sort']]['value'], $entry1['fields'][$atts['sort']]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry['fields'];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
echo '<span class="label">' . esc_html( sanitize_text_field( $form_field['label'] ) ) . ':</span>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field['id'] ) === absint( $form_field['id'] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field['value'] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Utilizzare il CSS sottostante per lo stile della vista non tabellare.
Per qualsiasi assistenza su come e dove aggiungere i CSS al vostro sito, consultate questo tutorial.
.wrapper {
width: 100%;
clear: both;
display: block;
}
span.details {
display: block;
margin-right: 2px;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
margin: 20px 0;
padding-bottom: 20px;
}
span.label {
font-weight: bold;
margin-right: 5px;
}
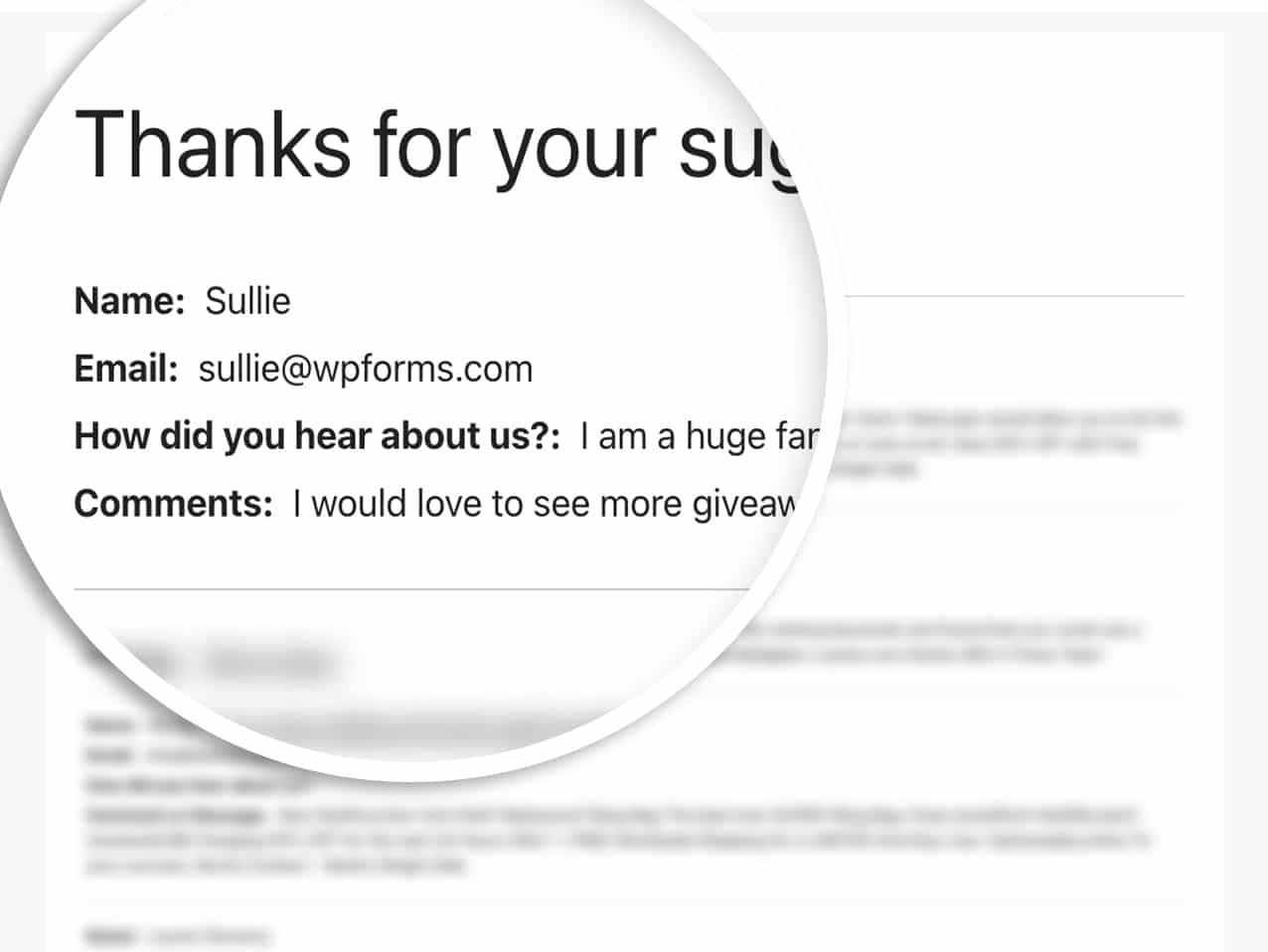