Möchten Sie Formulareinträge auf einer Seite innerhalb Ihrer Website anzeigen, um den Benutzern die ausgefüllten Formulareingaben öffentlich zu zeigen? In diesem Tutorial erfahren Sie, wie Sie diese Funktion auf Ihrer WordPress-Website erstellen und implementieren können.
Standardmäßig können nur Profi-Benutzer Formulareinträge in der WordPress-Verwaltung anzeigen, basierend auf den ihrem Benutzer zugewiesenen Zugriffskontrollen. Sie können jedoch ganz einfach die Funktionalität erstellen, um Formulareinträge auf dem Frontend Ihrer Website anzuzeigen.
Erstellen des Shortcodes
Zunächst stellen wir Ihnen einen Codeschnipsel zur Verfügung, der zu Ihrer Website hinzugefügt werden muss, damit Sie denselben Shortcode einfach wiederverwenden können, mit der Ausnahme, dass Sie nur die Formular-ID nach Bedarf aktualisieren müssen.
Fügen Sie einfach den folgenden Codeschnipsel in Ihre Website ein. Wenn Sie nicht sicher sind, wie das geht, lesen Sie bitte diese Anleitung.
/**
* Custom shortcode to display WPForms form entries in table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exists, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<table class="wpforms-frontend-entries">';
echo '<thead><tr>';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<th>';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</th>';
}
echo '</tr></thead>';
echo '<tbody>';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<tr>';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<td>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</td>';
}
echo '</tr>';
}
echo '</tbody>';
echo '</table>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Mit diesem Code-Snippet können Sie diesen Shortcode überall auf Ihrer Website verwenden, wo Shortcodes akzeptiert werden, z. B. auf Seiten, Beiträgen und Widget-Bereichen.
Allein durch die Verwendung dieser [wpforms_entries_table id="FORMID"]
werden Ihre Besucher die Einträge sehen, die für das Formular, das Sie in id="FORMID".
Die ID-Nummer eines Formulars finden Sie, indem Sie zu WPForms " All Forms gehen und sich die Nummer in der Spalte Shortcode für jedes Formular ansehen.
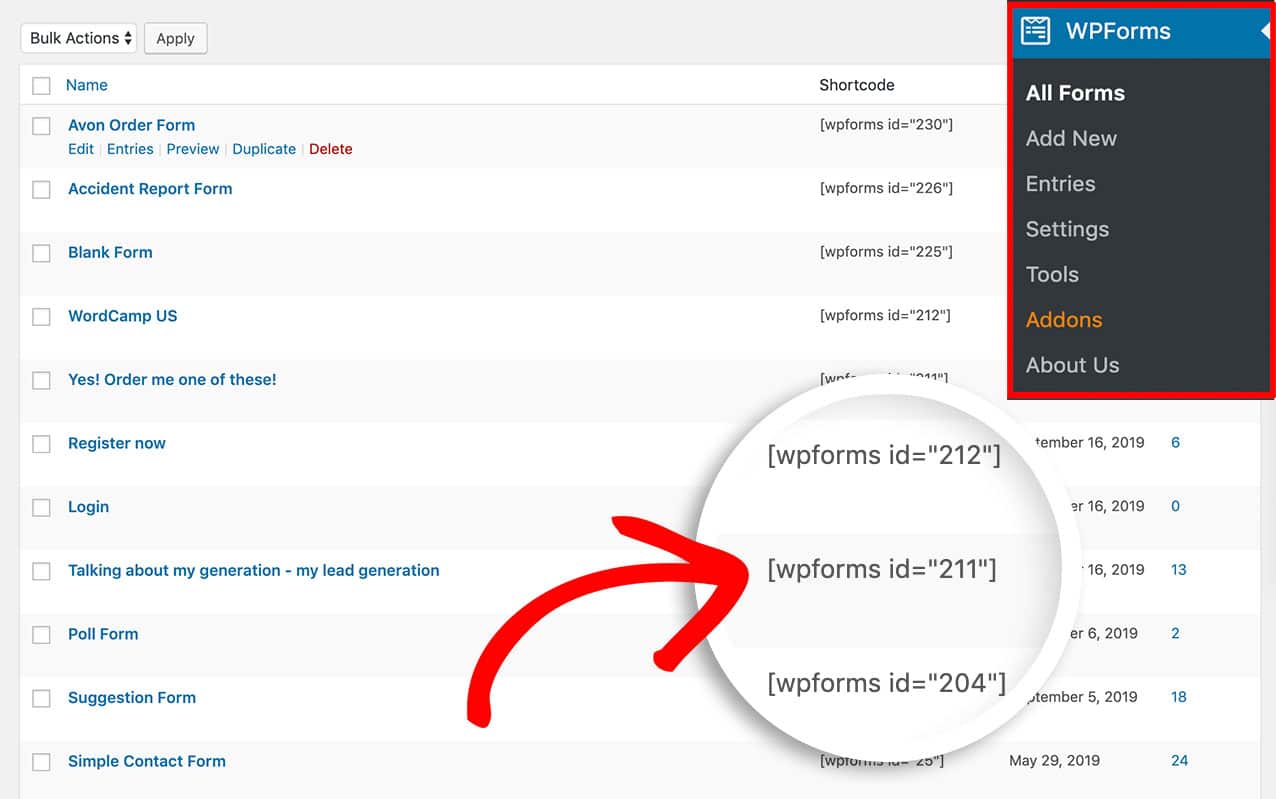
Anzeige von Formulareinträgen
Nachdem Sie den Shortcode erstellt haben, müssen Sie ihn zu einer Seite oder einem Beitrag auf Ihrer Website hinzufügen, um Ihre Einträge anzuzeigen.
Zunächst müssen Sie festlegen, für welche Formular-ID Sie diese Einträge anzeigen möchten. In unserem Beispiel werden wir die Einträge für die Formular-ID 211 anzeigen, so dass unser Shortcode wie folgt aussieht.
[wpforms_entries_table id="211"]
Das einzige erforderliche Attribut, um den Shortcode zu verwenden, ist die ID. Ohne diese Definition in Ihrem Shortcode wird nichts angezeigt, daher muss die Formular-ID im Shortcode aufgeführt werden.
Sie können Ihre Einträge jedoch noch weiter einschränken, indem Sie einige der Attribute des Shortcodes definieren, und wir werden jedes verfügbare Attribut überprüfen.
Definieren des Typs Attribut
Das Attribut type kann verwendet werden, wenn Sie nur mit Sternchen versehene Einträge anzeigen möchten. Hier ist ein Beispiel.
[wpforms_entries_table id="211" type="starred"]
Nachstehend finden Sie weitere verfügbare Typparameter, die Sie verwenden können:
- all - mit diesem Attribut werden alle Eintragsarten angezeigt.
- read - mit diesem Attribut werden nur Einträge angezeigt, die von einem Administrator eingesehen wurden.
- ungelesen - mit diesem Attribut werden nur Einträge angezeigt, die noch nicht von einem Administrator auf Ihrer Website geöffnet wurden.
- starred - mit diesem Attribut werden nur Einträge angezeigt, die von einem Administrator mit einem Sternchen versehen wurden
Verwendung des Benutzerattributs
Wenn ein Benutzer derzeit auf Ihrer Website angemeldet ist und Sie möchten, dass er nur die Formulareinträge sieht, die er eingereicht hat, verwenden Sie diesen Shortcode.
[wpforms_entries_table id="FORMID" user="current"]
Wenn der Benutzer nicht angemeldet ist, werden alle Einträge für dieses Formular angezeigt.
Wenn der Benutzer eingeloggt ist, aber das Formular nicht ausgefüllt hat (während er eingeloggt ist), wird auf der Seite "Keine Ergebnisse gefunden" angezeigt.
Definieren der Felder Attribut
Um nur bestimmte Felder anzuzeigen, müssen Sie zunächst die IDs der Felder herausfinden, die Sie anzeigen möchten. Um diese Feld-IDs herauszufinden, lesen Sie bitte diesen Leitfaden.
Sobald Sie wissen, wie die Feld-IDs lauten, verwenden Sie diese als Kurzcode. In diesem Beispiel wollen wir nur das Feld "Name", das die Feld-ID 0 hat, und das Feld " Kommentare", das die Feld-ID 2 hat.
[wpforms_entries_table id="211" fields="0,2"]
Hinweis: Mehrere Feld-IDs werden im Shortcode durch ein Komma getrennt.
Verwendung des Attributs Zahl
In der Standardeinstellung zeigt der Shortcode nur die ersten 30 Einträge an. Wenn Sie jedoch eine kleinere Anzahl anzeigen möchten, müssen Sie die Option number
Parameter. Wenn Sie zum Beispiel die ersten 20 Einträge anzeigen möchten, fügen Sie Ihren Shortcode wie folgt ein.
[wpforms_entries_table id="211" number="20"]
Die Variable number="20″, die innerhalb des Shortcodes übergeben wird, bestimmt, wie viele Einträge die Tabelle anzeigen wird. Wenn Sie alle Einträge anzeigen möchten, ändern Sie die Zahl in 9999.
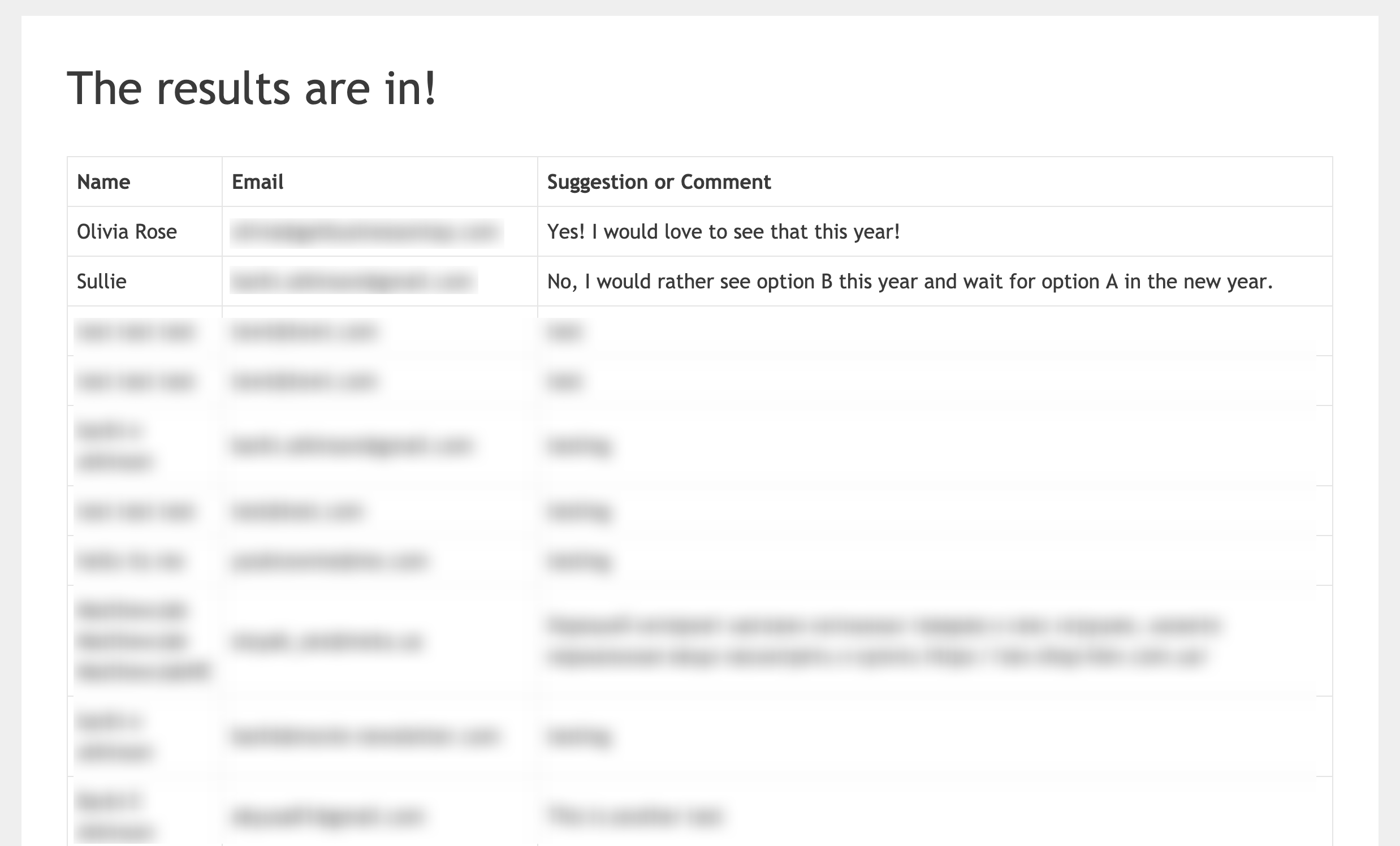
Verwendung des Attributs sort and order
Wenn Sie Ihren Shortcode verwenden, können Sie dem Shortcode mitteilen, nach welchem Feld Sie die Tabelle sortieren möchten, und dann festlegen, ob Sie sie nach asc (aufsteigende Reihenfolge) oder desc (absteigende Reihenfolge) sortieren möchten. Ein Beispiel für diesen Shortcode würde wie folgt aussehen.
[wpforms_entries_table id="211" sort="1" order="asc"]
In diesem Beispiel zeigen wir eine Tabelle mit Einträgen für die Formular-ID 211 an und sortieren diese Tabelle in aufsteigender Reihenfolge anhand der Feld-ID 1, der Feld-ID für das Feld Name des Formulars.
Gestalten des Tisches (optional)
Die Gestaltung der Tabelle für die Einträge ist völlig optional, da die meisten Themes und auch die Browser eine Standard-Tabellengestaltung eingebaut haben. Wir wollten Ihnen jedoch ein Beispiel geben. Wenn Sie also das Standarddesign der Tabelle ändern möchten, müssen Sie dieses CSS kopieren und in Ihre Website einfügen.
Wenn Sie wissen möchten, wie und wo Sie CSS zu Ihrer Website hinzufügen können, lesen Sie bitte dieses Tutorial.
Die Gestaltung der Eingangstabelle hängt von der Standardgestaltung ab, die Ihr Thema für Tabellen hat oder nicht hat.
table {
border-collapse: collapse;
}
thead tr {
height: 60px;
}
table, th, td {
border: 1px solid #000000;
}
td {
white-space: normal;
max-width: 33%;
width: 33%;
word-break: break-all;
height: 60px;
padding: 10px;
}
tr:nth-child(even) {
background: #ccc
}
tr:nth-child(odd) {
background: #fff
}
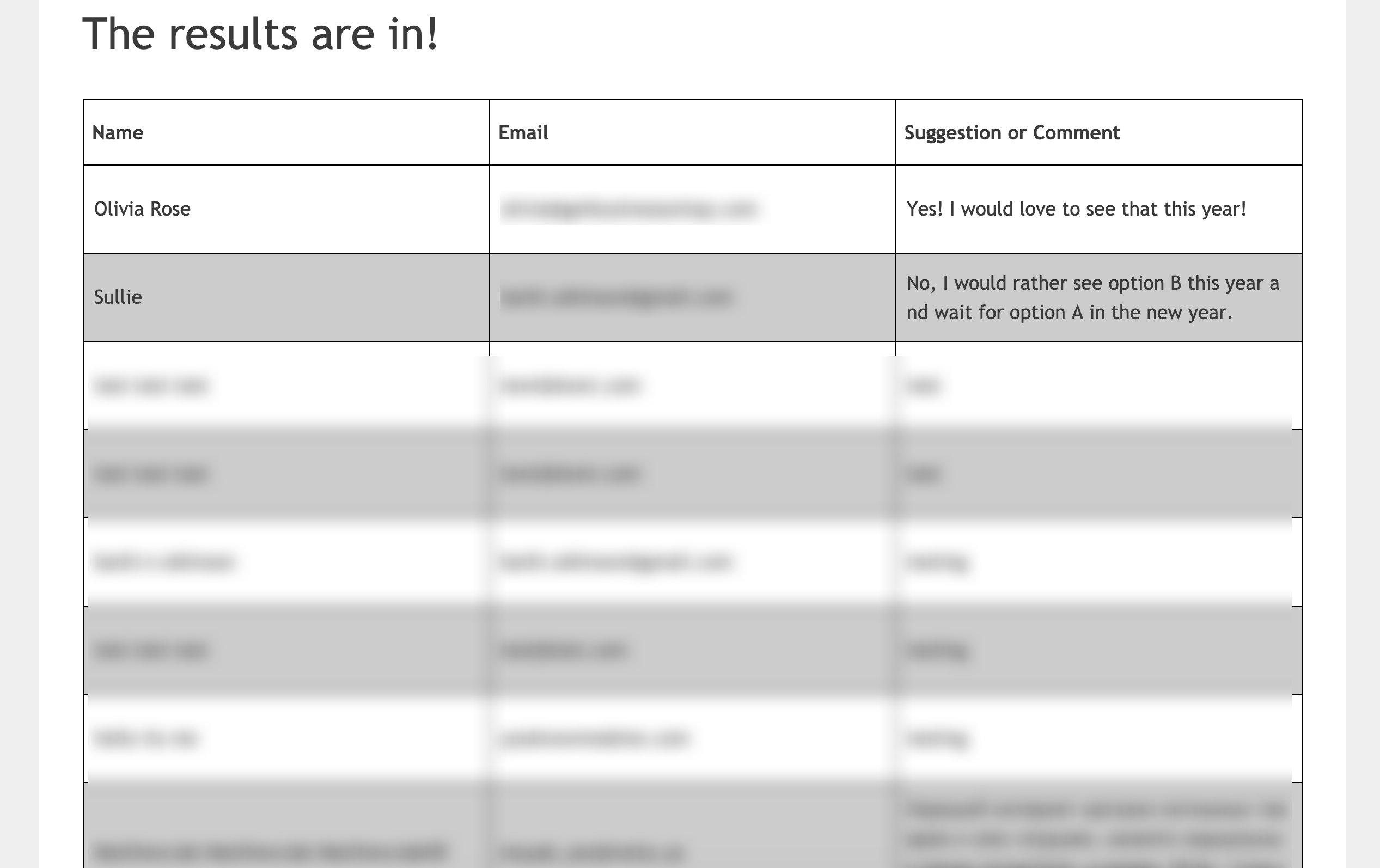
Hinzufügen einer Nicht-Tabellenansicht
Wenn Sie es vorziehen, keine HTML-Tabellenansicht zur Anzeige Ihrer Einträge zu verwenden, haben wir auch eine alternative Lösung für Sie. Anstatt Ihren Shortcode mit dem obigen Codeschnipsel zu erstellen, verwenden Sie stattdessen diesen Codeschnipsel.
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="header-row">';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<span class="column-label">';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</span>';
}
echo '</div>';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Da wir jedoch keine Standardtabelle verwenden, wird bei dieser Option kein Standard-Styling angewendet. Daher ist das CSS im nächsten Schritt von entscheidender Bedeutung, aber wir werden die verwendeten CSS-Klassen auflisten, damit Sie Ihr eigenes Styling auf diese Option anwenden können.
Styling des Nicht-Tisches
Nachfolgend finden Sie eine detaillierte Liste der verwendeten CSS-Klassen und deren Zweck (falls erforderlich).
- .wrapper - dies ist ein allgemeiner Wrapper um den gesamten Block von Einträgen und kann verwendet werden, wenn Sie die Breite des Blocks reduzieren möchten, so dass er nicht 100% der Seite einnimmt.
- .header-row - dies ist ein weiterer allgemeiner Wrapper um die Beschriftungen jeder Spalte (nur die Beschriftungen), wenn Sie die Hintergrundfarbe des Beschriftungsabschnitts ändern möchten.>
- .column-label - dieser Wrapper umgibt jeden einzelnen Label-Titel. Zum Beispiel haben Name, E-Mail-Adresse und Vorschlag/Kommentar jeweils einen dieser Wrapper um sich herum und können verwendet werden, um die Größe dieses Textes festzulegen.
- .entries - dies ist ein allgemeiner Wrapper um die Einträge selbst, wenn Sie die Hintergrundfarbe dieses Abschnitts ändern möchten, damit sie anders aussieht als die Beschriftungen.
- .entry-details - dies ist ein allgemeiner Wrapper um jeden einzelnen Eintrag und könnte nützlich sein, wenn Sie für jede Eintragszeile eine andere Hintergrundfarbe vorsehen möchten.
- .details - jede Informationsspalte ist in diese Klasse eingeschlossen, so dass Sie jede gewünschte zusätzliche Gestaltung wie Schriftgröße, Farbe usw. hinzufügen können.
Sie können das unten stehende CSS verwenden, um ein Standard-Styling für Ihre Nicht-Tabellenansicht hinzuzufügen.
Wenn Sie wissen möchten, wie und wo Sie CSS zu Ihrer Website hinzufügen können, lesen Sie bitte dieses Tutorial.
.wrapper {
width: 100%;
clear: both;
display: block;
}
.header-row {
float: left;
width: 100%;
margin-bottom: 20px;
border-top: 1px solid #eee;
padding: 10px 0;
border-bottom: 1px solid #eee;
}
span.details, span.column-label {
display: inline-block;
float: left;
width: 33%;
margin-right: 2px;
text-align: center;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.header-row span.column-label {
text-transform: uppercase;
letter-spacing: 2px;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
float: left;
margin: 20px 0;
padding-bottom: 20px;
}
Hinweis: Das oben angegebene CSS basiert auf der Anzeige von nur 3 Feldern Ihres Eintrags, dem Namen, der E-Mail und dem Kommentarfeld. Wenn Sie mehr Felder anzeigen möchten, denken Sie bitte daran, Ihr CSS entsprechend zu aktualisieren.
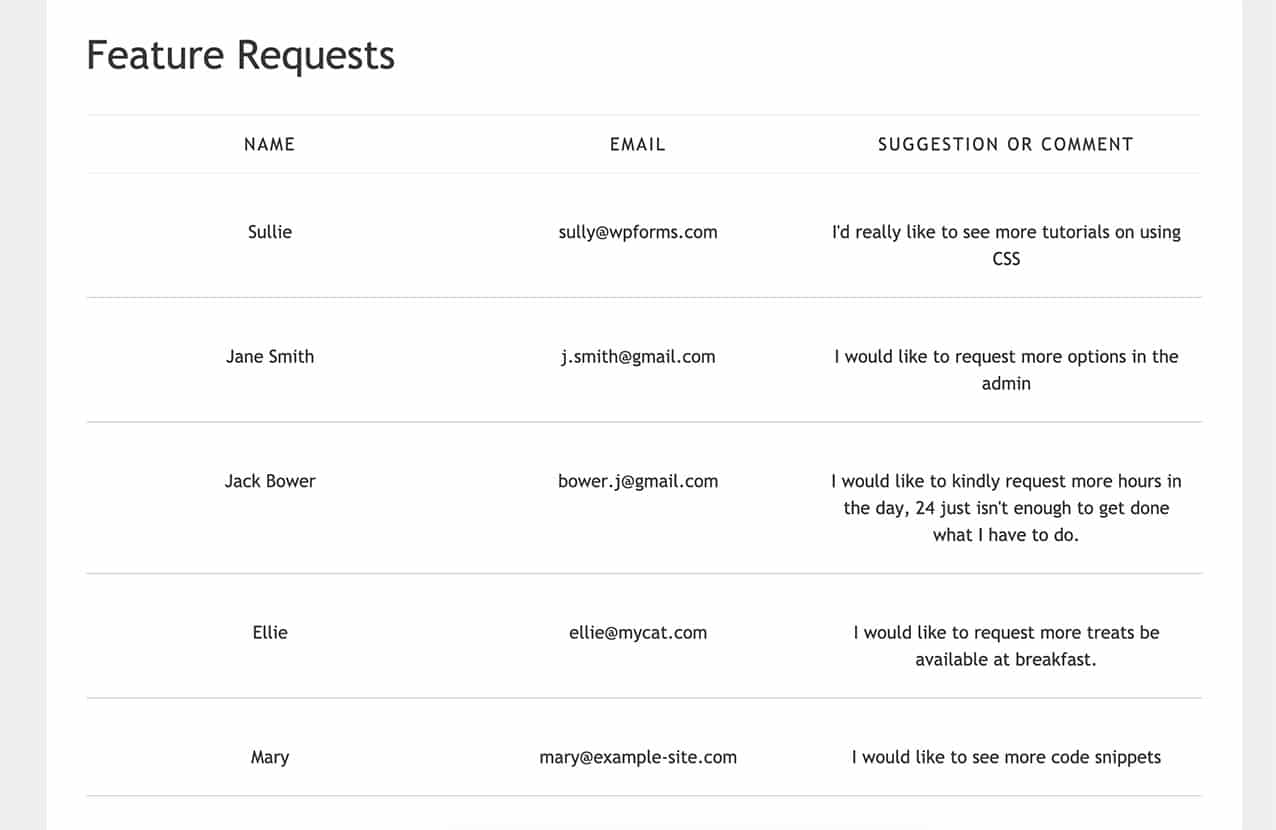
Und schon haben Sie einen Shortcode erstellt, den Sie an verschiedenen Stellen auf Ihrer Website einfügen können, um Ihre Formulareinträge für Nicht-Administratoren anzuzeigen. Möchten Sie auch anzeigen, wie viele Einträge in Ihrem Formular noch übrig sind, wenn Sie das Form Locker Addon verwenden? Probieren Sie unser Code-Snippet für die Anzeige der Anzahl der verbleibenden Einträge aus.
Referenzfilter
FAQ
Hier finden Sie Antworten auf einige der häufigsten Fragen, die wir zur Anzeige von Formulareinträgen auf einer Seite oder einem Beitrag in WordPress erhalten.
F: Wie kann ich nur die Anzahl der ungelesenen Einträge anzeigen?
A: Sie fügen dieses Snippet zu Ihrer Website hinzu.
<?php
/**
* Custom shortcode to display WPForms form entries count for a form.
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
*/
function wpf_dev_entries_count( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'type' => 'all', // all, unread, read, or starred.
],
$atts
);
if ( empty( $atts['id'] ) ) {
return;
}
$args = [
'form_id' => absint( $atts['id'] ),
];
if ( $atts['type'] === 'unread' ) {
$args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$args['starred'] = '1';
}
return wpforms()->entry->get_entries( $args, true );
}
add_shortcode( 'wpf_entries_count', 'wpf_dev_entries_count' );
Dann können Sie diesen Shortcode überall auf Ihrer WordPress-Website verwenden, wo Shortcodes akzeptiert werden.
[wpf_entries_count id="13" type="unread"]
Sie können einfach den Typ in ungelesen, gelesen oder mit Sternen versehen ändern.
F: Warum werden meine neueren Einträge nicht angezeigt?
A: Das liegt wahrscheinlich am Caching auf Server-, Site- oder Browser-Ebene. Bitte überprüfen Sie, ob Ihr Cache vollständig geleert ist.
F: Muss ich überhaupt Spalten haben?
A: Nein, ganz und gar nicht. Sie können Ihre Einträge anzeigen, indem Sie die Beschriftung vor dem Feldwert platzieren und jedes Feld mit diesem Snippet und CSS in einer separaten Zeile anzeigen lassen.
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts['id'] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts['id'] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts['fields'] ) && $atts['fields'] !== '' ? explode( ',', str_replace( ' ', '', $atts['fields'] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data['fields'];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data['fields'][$field_id] ) ) {
$form_fields[$field_id] = $form_data['fields'][$field_id];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field['type'], $form_fields_disallow, true ) ) {
unset( $form_fields[$field_id] );
}
}
$entries_args = [
'form_id' => absint( $atts['id'] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts['user'] ) ) {
if ( $atts['user'] === 'current' && is_user_logged_in() ) {
$entries_args['user_id'] = get_current_user_id();
} else {
$entries_args['user_id'] = absint( $atts['user'] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts['number'] ) ) {
$entries_args['number'] = absint( $atts['number'] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts['type'] === 'unread' ) {
$entries_args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$entries_args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$entries_args['starred'] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key]['fields'] = json_decode($entry['fields'], true);
$entries[$key]['meta'] = json_decode($entry['meta'], true);
}
if ( !empty($atts['sort']) && isset($entries[0]['fields'][$atts['sort']] ) ) {
if ( strtolower($atts['order']) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1['fields'][$atts['sort']]['value'], $entry2['fields'][$atts['sort']]['value']);
});
} elseif ( strtolower($atts['order']) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2['fields'][$atts['sort']]['value'], $entry1['fields'][$atts['sort']]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry['fields'];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
echo '<span class="label">' . esc_html( sanitize_text_field( $form_field['label'] ) ) . ':</span>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field['id'] ) === absint( $form_field['id'] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field['value'] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
Verwenden Sie das folgende CSS für die Gestaltung der Nicht-Tabellenansicht.
Wenn Sie wissen möchten, wie und wo Sie CSS zu Ihrer Website hinzufügen können, lesen Sie bitte dieses Tutorial.
.wrapper {
width: 100%;
clear: both;
display: block;
}
span.details {
display: block;
margin-right: 2px;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
margin: 20px 0;
padding-bottom: 20px;
}
span.label {
font-weight: bold;
margin-right: 5px;
}
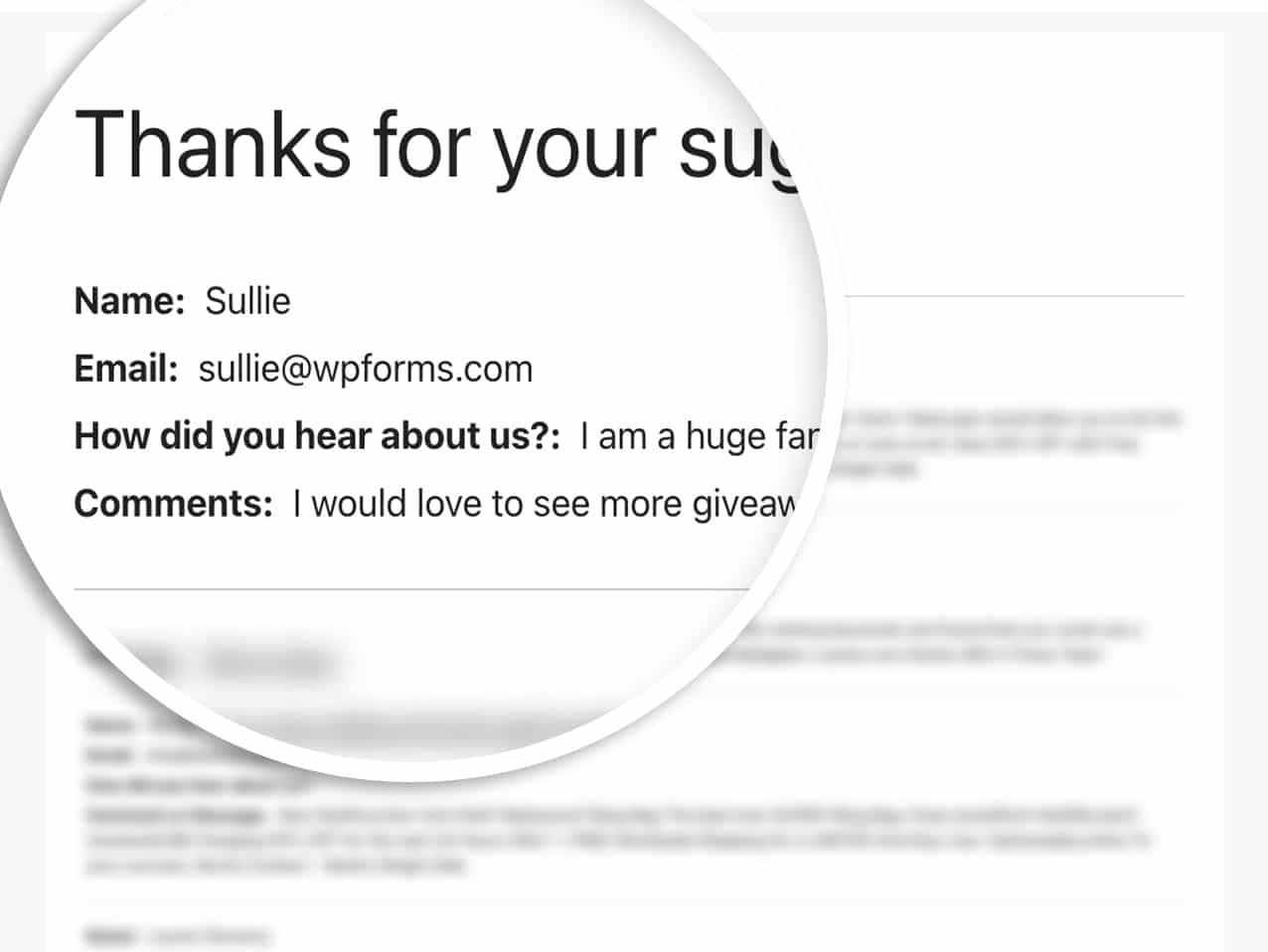