フォームに入力された内容をサイト内のページに表示し、入力が完了したフォームをユーザーに公開したいですか?このチュートリアルでは、WordPress サイトにこの機能を作成し実装する方法を説明します。
デフォルトでは、プロユーザーのみが、ユーザーに割り当てられたアクセスコントロールに基づいてWordPress 管理画面からフォームエントリーを表示できます。しかし、サイトのフロントエンドにフォームエントリーを表示する機能を簡単に作成することができます。
ショートコードの作成
最初に、あなたのサイトに追加する必要があるコードスニペットを提供しますので、必要に応じてフォームIDを更新するだけで、同じショートコードを簡単に再利用することができます。
以下のコード・スニペットをサイトに追加するだけです。やり方がわからない場合は、こちらのチュートリアルをご覧ください。
/**
* Custom shortcode to display WPForms form entries in table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exists, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<table class="wpforms-frontend-entries">';
echo '<thead><tr>';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<th>';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</th>';
}
echo '</tr></thead>';
echo '<tbody>';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<tr>';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<td>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</td>';
}
echo '</tr>';
}
echo '</tbody>';
echo '</table>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
このコードスニペットでできることは、ページ、投稿、ウィジェットエリアなど、ショートコードを使えるサイトであればどこでもこのショートコードを使えるようにすることです。
これを使うだけで [wpforms_entries_table id="FORMID"]
で指定したフォームに送信されたエントリーが表示されます。 id="FORMID".
フォームの ID 番号は WPForms " All Formsで各フォームのShortcodeカラムの番号を見ればわかります。
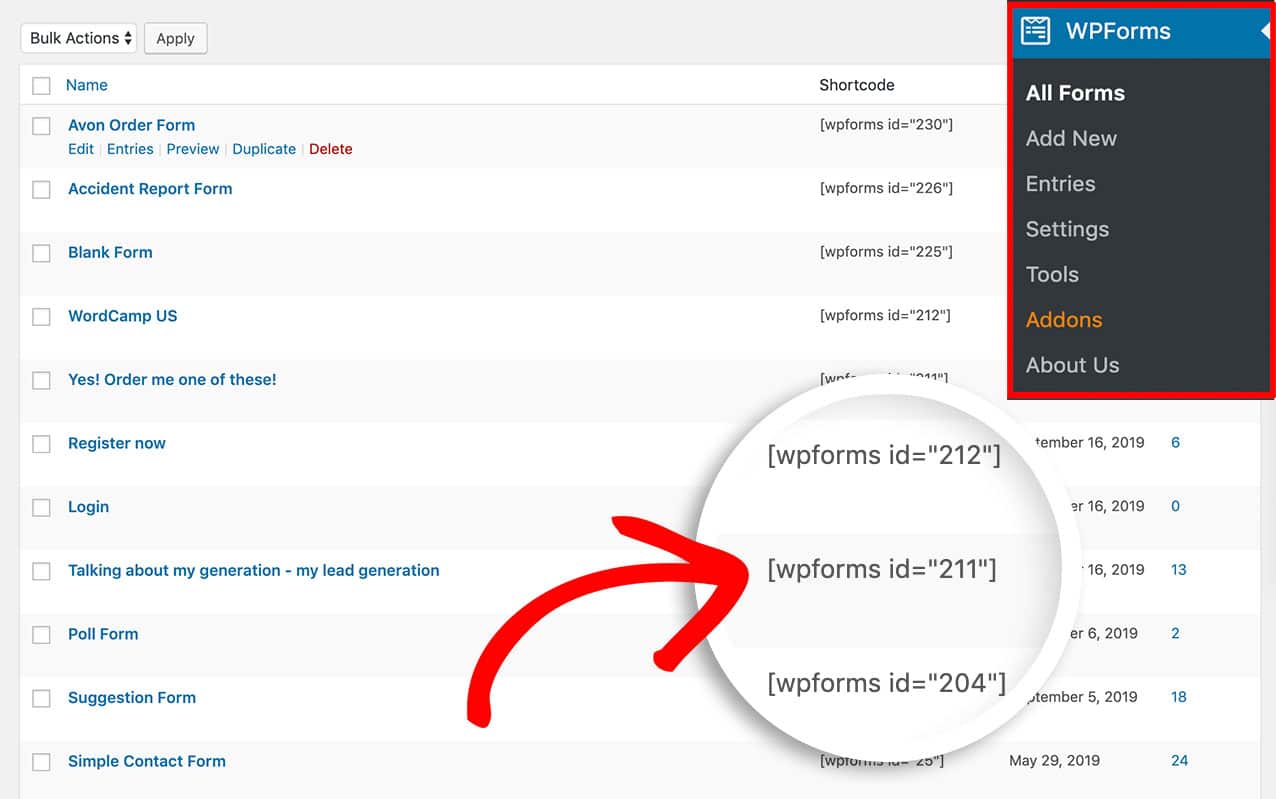
フォーム入力の表示
ショートコードを作成したら、エントリーを表示するためにサイトのページや投稿に追加する必要があります。
まず、これらのエントリーを表示したいフォームIDを決定する必要があります。この例では、フォームIDが211のエントリーを表示するので、ショートコードは次のようになります。
[wpforms_entries_table id="211"]
ショートコードを使用するために必要な唯一の属性はidで、これをショートコードで定義しないと何も表示されないので、フォームIDはショートコード内に記載する必要があります。
しかし、ショートコードの属性のいくつかを定義することによって、エントリーをさらに絞り込むことができます。
type属性の定義
type属性を使用すると、星印の付いたエントリーだけを表示することができます。以下はその例です。
[wpforms_entries_table id="211" type="starred"]
以下に、使用可能なその他の型パラメーターを示す:
- all- この属性を使用すると、すべてのエントリー・タイプが表示されます。
- read- この属性は、管理者によって閲覧されたエントリのみを表示します。
- unread- この属性を使用すると、サイトの管理者によって開かれていないエントリのみが表示されます。
- starred- この属性は、管理者によってスターが付けられたエントリのみを表示します。
ユーザー属性の活用
ユーザーが現在あなたのサイトにログインしていて、そのユーザーが送信したフォーム項目だけを表示したい場合は、このショートコードを使用してください。
[wpforms_entries_table id="FORMID" user="current"]
ユーザーがログインしていない場合、このフォームのすべてのエントリーが表示されます。
ユーザーがログインしているにもかかわらず、フォームを完了していない場合(ログイン中)、No results found.がページに表示されます。
fields属性の定義
特定のフィールドだけを表示するには、まず表示したいフィールドのIDを調べる必要があります。これらのフィールドIDを見つけるには、こちらのチュートリアルをご覧ください。
フィールドIDがわかったら、これをショートコードとして使います。この例では、フィールドID 0である「名前」フィールドと、フィールドID 2である「コメント」フィールドだけが必要です。
[wpforms_entries_table id="211" fields="0,2"]
注:複数のフィールドIDは、ショートコード内でカンマで区切ります。
番号属性の使用
デフォルトでは、ショートコードは最初の30件だけを表示します。しかし、もっと少ない量を表示したい場合は number
パラメータを追加します。例えば、最初の20件のエントリーを表示したい場合は、以下のようにショートコードを追加します。
[wpforms_entries_table id="211" number="20"]
ショートコード内で渡されるnumber="20″変数は、テーブルが表示するエントリーの数を決定する。すべてのエントリーを表示したい場合は、数値を 9999に変更してください。
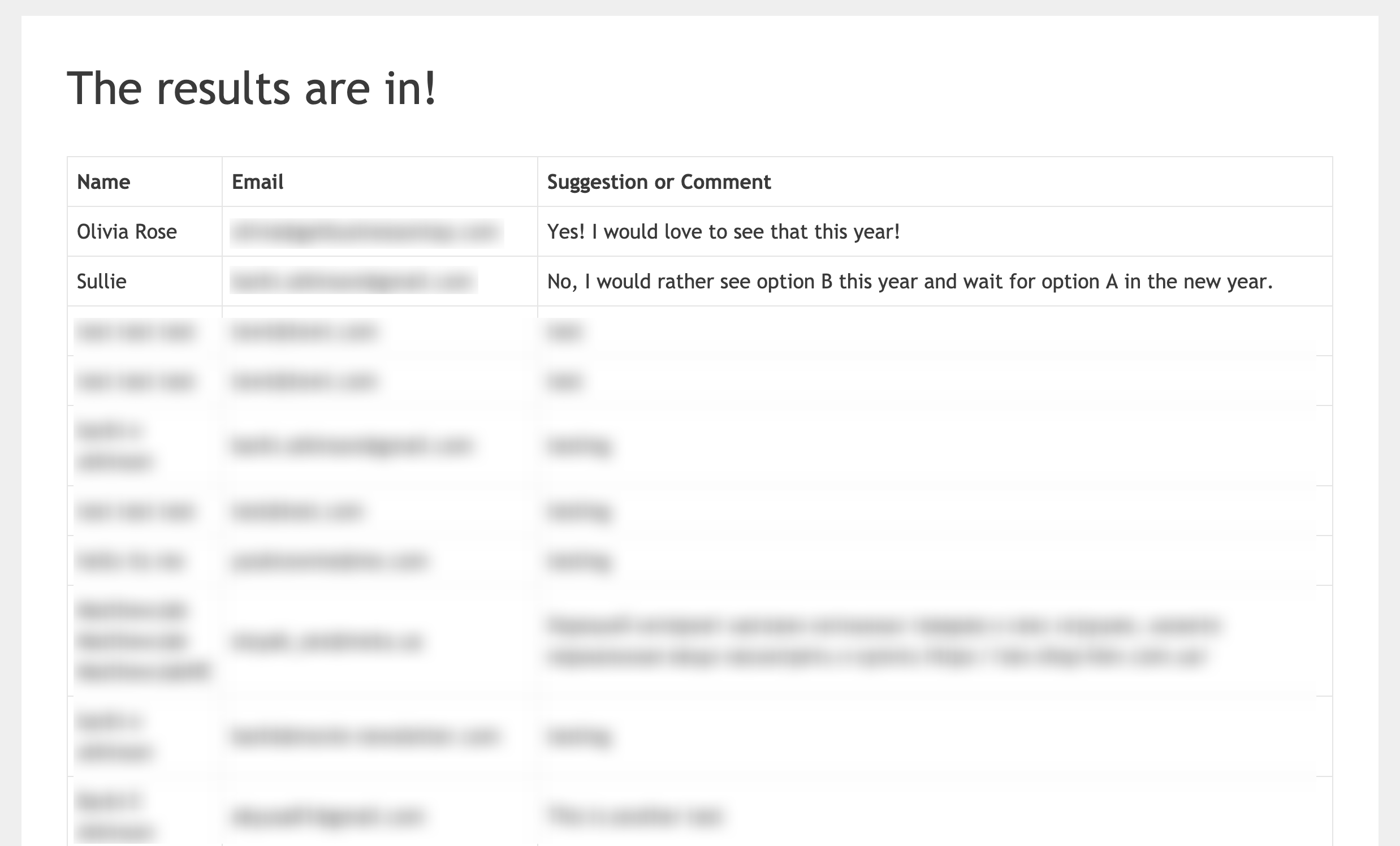
並べ替えと 順序属性の使用
ショートコードを使用する際、どのフィールドでテーブルをソートしたいかをショートコードに伝え、asc(昇順)かdesc(降順)でソートしたいかを定義することができます。ショートコードの例は次のようになります。
[wpforms_entries_table id="211" sort="1" order="asc"]
この例では、フォームID211のエントリーのテーブルを表示し、フォームのNameフィールドのフィールドIDであるフィールドID1を使ってテーブルをasc(昇順)にソートします。
テーブルのスタイリング(オプション)
ほとんどのテーマにはデフォルトのテーブルスタイルが組み込まれていますし、ブラウザにも組み込まれています。デフォルトのテーブルスタイルを変更したい場合は、このCSSをコピーしてあなたのサイトに貼り付けてください。
サイトにCSSを追加する方法と場所については、こちらのチュートリアルをご覧ください。
エントリーテーブルのスタイリングは、テーマがテーブルに対してデフォルトのスタイリングを持っているか持っていないかによって異なります。
table {
border-collapse: collapse;
}
thead tr {
height: 60px;
}
table, th, td {
border: 1px solid #000000;
}
td {
white-space: normal;
max-width: 33%;
width: 33%;
word-break: break-all;
height: 60px;
padding: 10px;
}
tr:nth-child(even) {
background: #ccc
}
tr:nth-child(odd) {
background: #fff
}
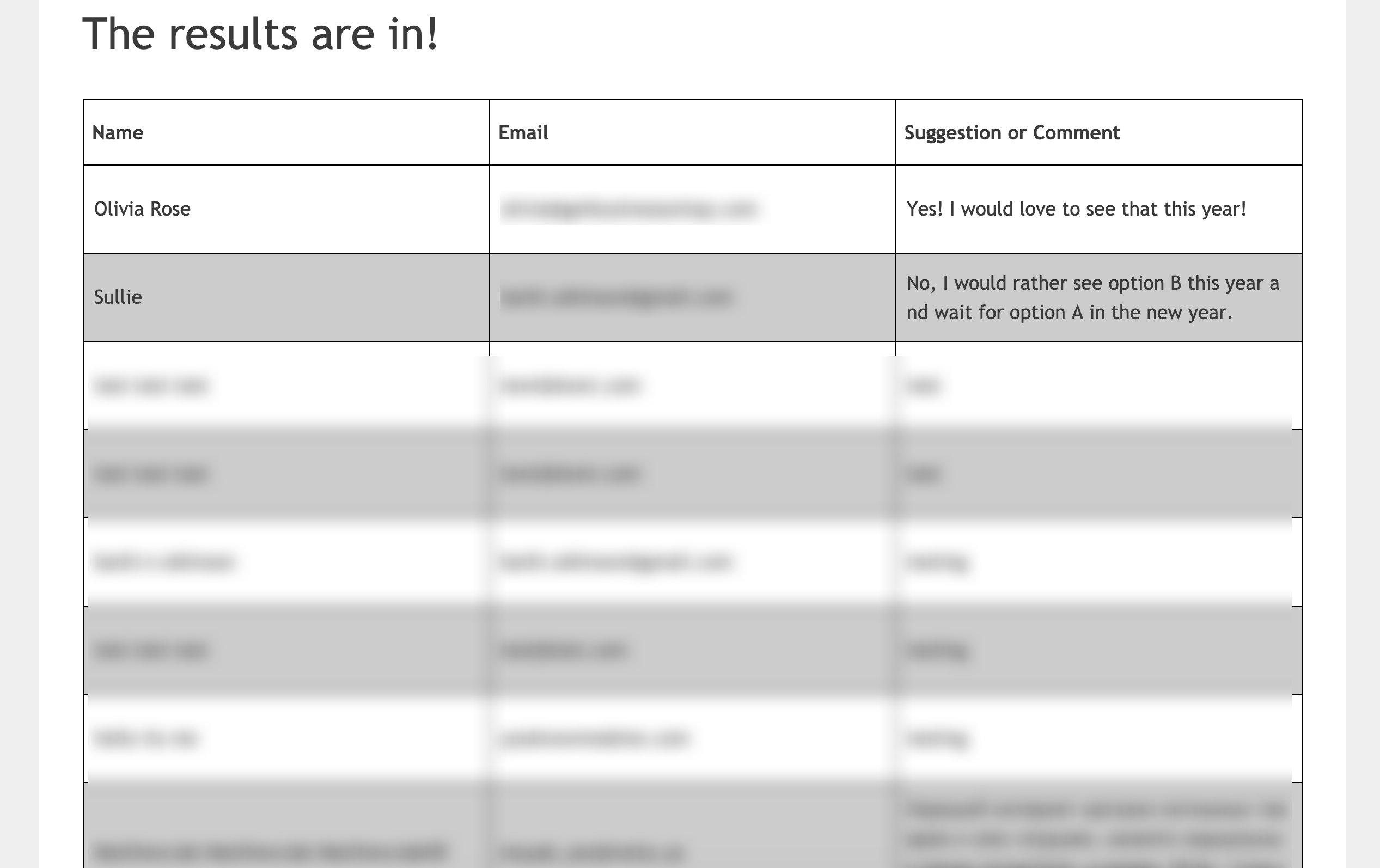
テーブル以外のビューを追加する
エントリーを表示するのにHTMLテーブル・ビューを使いたくない場合は、別の方法もあります。上記のコード・スニペットでショートコードを作成する代わりに、以下のコード・スニペットを使用してください。
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts[ 'id' ] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts[ 'id' ] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts[ 'fields' ] ) && $atts[ 'fields' ] !== '' ? explode( ',', str_replace( ' ', '', $atts[ 'fields' ] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data[ 'fields' ];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data[ 'fields' ][ $field_id ] ) ) {
$form_fields[ $field_id ] = $form_data[ 'fields' ][ $field_id ];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field[ 'type' ], $form_fields_disallow, true ) ) {
unset( $form_fields[ $field_id ] );
}
}
$entries_args = [
'form_id' => absint( $atts[ 'id' ] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts[ 'user' ] ) ) {
if ( $atts[ 'user' ] === 'current' && is_user_logged_in() ) {
$entries_args[ 'user_id' ] = get_current_user_id();
} else {
$entries_args[ 'user_id' ] = absint( $atts[ 'user' ] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts[ 'number' ] ) ) {
$entries_args[ 'number' ] = absint( $atts[ 'number' ] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts[ 'type' ] === 'unread' ) {
$entries_args[ 'viewed' ] = '0';
} elseif( $atts[ 'type' ] === 'read' ) {
$entries_args[ 'viewed' ] = '1';
} elseif ( $atts[ 'type' ] === 'starred' ) {
$entries_args[ 'starred' ] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key][ 'fields' ] = json_decode($entry[ 'fields' ], true);
$entries[$key][ 'meta' ] = json_decode($entry[ 'meta' ], true);
}
if ( !empty($atts[ 'sort' ]) && isset($entries[0][ 'fields' ][$atts[ 'sort' ]] ) ) {
if ( strtolower($atts[ 'order' ]) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ]);
});
} elseif ( strtolower($atts[ 'order' ]) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2[ 'fields' ][$atts[ 'sort' ]][ 'value' ], $entry1[ 'fields' ][$atts[ 'sort' ]]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="header-row">';
// Loop through the form data so we can output form field names in
// the table header.
foreach ( $form_fields as $form_field ) {
// Output the form field name/label.
echo '<span class="column-label">';
echo esc_html( sanitize_text_field( $form_field[ 'label' ] ) );
echo '</span>';
}
echo '</div>';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry[ 'fields' ];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field[ 'id' ] ) === absint( $form_field[ 'id' ] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field[ 'value' ] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
しかし、ここで重要なことは、標準的なテーブルを使用していないので、このオプションを使用してもデフォルトのスタイルは適用されないということです。したがって、次のステップのCSSが重要ですが、このオプションに独自のスタイルを適用できるように、使用されているCSSクラスを列挙します。
テーブル以外のスタイリング
以下は、使用されているCSSクラスとその目的(必要な場合)の詳細リストです。
- .wrapper- これはエントリーのブロック全体を包むもので、ブロックの幅を狭めてページの100%を引き伸ばさないようにしたい場合に使用します。
- .header-row-これは、各列のラベル(ラベルのみ)を囲むもう一つの全体的なラッパーで、ラベル部分の背景色を変更したい場合に使用する。
- .column-label- このラッパーは個々のラベルタイトルを囲みます。例えば、Name、Email Address、Suggestion/Commentはそれぞれこのラッパーで囲まれており、このテキストのサイズをターゲットにするために使用することができます。
- .entries- このセクションの背景色をラベルとは異なる色に変更したい場合、これはエントリー自体の全体的なラッパーです。
- .entry-details-これは各エントリーを包む全体的なラッパーで、各エントリー行に異なる色の背景を提供したい場合に便利です。
- .details- 各カラムの情報はこのクラスでラップされ、フォントサイズや色など、お好みのスタイルを追加することができます。
以下のCSSを使って、テーブル以外のビューにデフォルトのスタイルを追加することができます。
サイトにCSSを追加する方法と場所については、こちらのチュートリアルをご覧ください。
.wrapper {
width: 100%;
clear: both;
display: block;
}
.header-row {
float: left;
width: 100%;
margin-bottom: 20px;
border-top: 1px solid #eee;
padding: 10px 0;
border-bottom: 1px solid #eee;
}
span.details, span.column-label {
display: inline-block;
float: left;
width: 33%;
margin-right: 2px;
text-align: center;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.header-row span.column-label {
text-transform: uppercase;
letter-spacing: 2px;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
float: left;
margin: 20px 0;
padding-bottom: 20px;
}
注:上記のCSSは、お名前、メールアドレス、コメントの3つのフィールドのみを表示することを前提としています。より多くのフィールドを表示したい場合は、CSSを適宜更新してください。
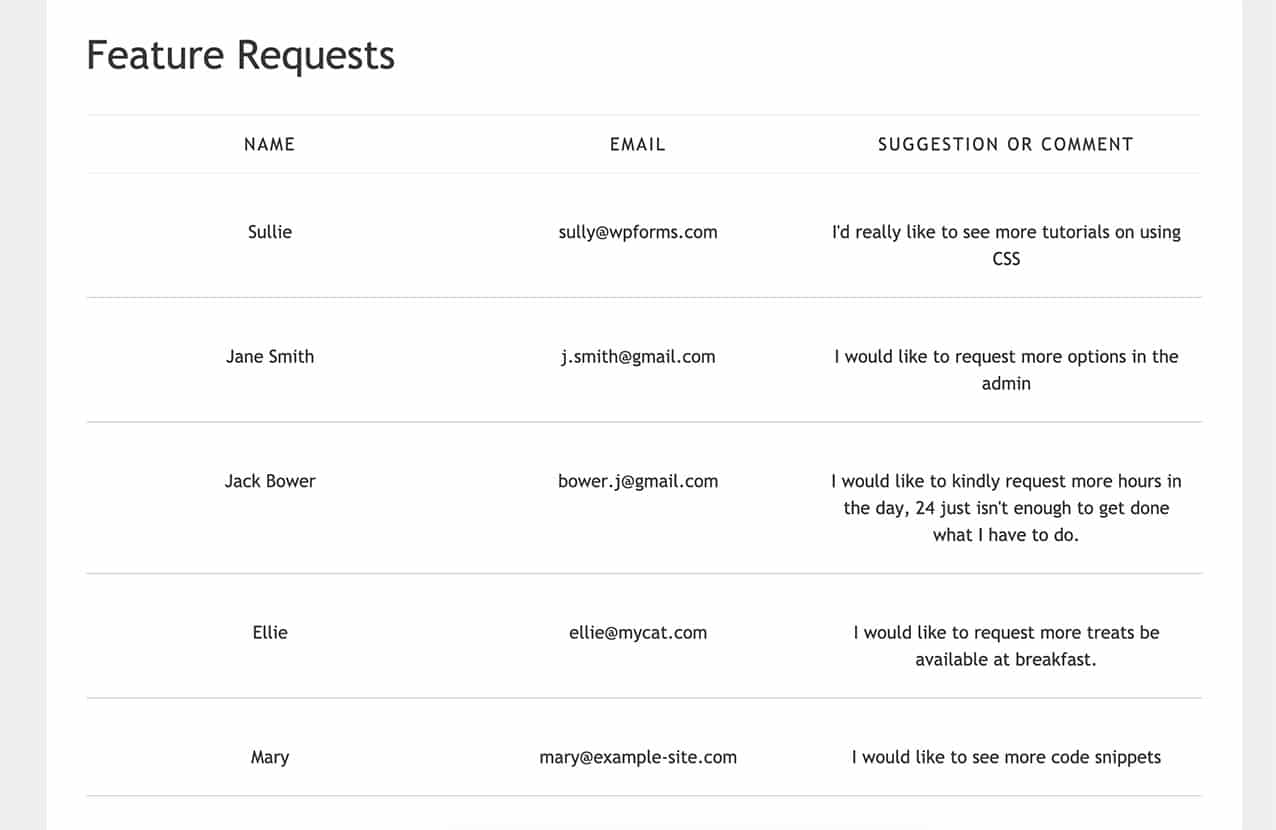
これで、管理者以外のユーザーにもフォームの入力内容を表示できるように、サイトのさまざまな場所に追加できるショートコードが作成できました。Form Locker アドオンを使っているときにフォームに残っているエントリの数を表示したいですか?残りのエントリー数を表示する方法」のコードスニペットを試してみてください。
リファレンス・フィルター
よくあるご質問
WordPressのページや投稿にフォームを表示する方法について、よくある質問にお答えします。
Q: 未読エントリーの数だけを表示するにはどうしたらいいですか?
A:このスニペットをサイトに追加します。
<?php
/**
* Custom shortcode to display WPForms form entries count for a form.
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
*/
function wpf_dev_entries_count( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'type' => 'all', // all, unread, read, or starred.
],
$atts
);
if ( empty( $atts['id'] ) ) {
return;
}
$args = [
'form_id' => absint( $atts['id'] ),
];
if ( $atts['type'] === 'unread' ) {
$args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$args['starred'] = '1';
}
return wpforms()->entry->get_entries( $args, true );
}
add_shortcode( 'wpf_entries_count', 'wpf_dev_entries_count' );
このショートコードは、あなたのWordPressサイトでショートコードが使える場所ならどこでも自由に使えます。
[wpf_entries_count id="13" type="unread"]
タイプは、未読、既読、スター付きに変更するだけです。
Q: 新しいエントリーが表示されないのはなぜですか?
A:これは、サーバーレベル、サイトレベル、またはブラウザレベルのいずれかのキャッシュが原因である可能性があります。キャッシュが完全にクリアされているかご確認ください。
Q:コラムは必要ですか?
A:そんなことはありません。このスニペットとCSSを使って、ラベルをフィールドの値の前に置き、各フィールドを別々の行にすることで、エントリーを表示することができます。
/**
* Custom shortcode to display WPForms form entries in non-table view.
*
* Basic usage: [wpforms_entries_table id="FORMID"].
*
* Possible shortcode attributes:
* id (required) Form ID of which to show entries.
* user User ID, or "current" to default to current logged in user.
* fields Comma separated list of form field IDs.
* number Number of entries to show, defaults to 30.
*
* @link https://wpforms.com/developers/how-to-display-form-entries/
*
* Realtime counts could be delayed due to any caching setup on the site
*
* @param array $atts Shortcode attributes.
*
* @return string
*/
function wpf_entries_table( $atts ) {
// Pull ID shortcode attributes.
$atts = shortcode_atts(
[
'id' => '',
'user' => '',
'fields' => '',
'number' => '',
'type' => 'all', // all, unread, read, or starred.
'sort' => '',
'order' => 'asc',
],
$atts
);
// Check for an ID attribute (required) and that WPForms is in fact
// installed and activated.
if ( empty( $atts['id'] ) || ! function_exists( 'wpforms' ) ) {
return;
}
// Get the form, from the ID provided in the shortcode.
$form = wpforms()->form->get( absint( $atts['id'] ) );
// If the form doesn't exist, abort.
if ( empty( $form ) ) {
return;
}
// Pull and format the form data out of the form object.
$form_data = ! empty( $form->post_content ) ? wpforms_decode( $form->post_content ) : '';
// Check to see if we are showing all allowed fields, or only specific ones.
$form_field_ids = isset( $atts['fields'] ) && $atts['fields'] !== '' ? explode( ',', str_replace( ' ', '', $atts['fields'] ) ) : [];
// Setup the form fields.
if ( empty( $form_field_ids ) ) {
$form_fields = $form_data['fields'];
} else {
$form_fields = [];
foreach ( $form_field_ids as $field_id ) {
if ( isset( $form_data['fields'][$field_id] ) ) {
$form_fields[$field_id] = $form_data['fields'][$field_id];
}
}
}
if ( empty( $form_fields ) ) {
return;
}
// Here we define what the types of form fields we do NOT want to include,
// instead they should be ignored entirely.
$form_fields_disallow = apply_filters( 'wpforms_frontend_entries_table_disallow', [ 'divider', 'html', 'pagebreak', 'captcha' ] );
// Loop through all form fields and remove any field types not allowed.
foreach ( $form_fields as $field_id => $form_field ) {
if ( in_array( $form_field['type'], $form_fields_disallow, true ) ) {
unset( $form_fields[$field_id] );
}
}
$entries_args = [
'form_id' => absint( $atts['id'] ),
];
// Narrow entries by user if user_id shortcode attribute was used.
if ( ! empty( $atts['user'] ) ) {
if ( $atts['user'] === 'current' && is_user_logged_in() ) {
$entries_args['user_id'] = get_current_user_id();
} else {
$entries_args['user_id'] = absint( $atts['user'] );
}
}
// Number of entries to show. If empty, defaults to 30.
if ( ! empty( $atts['number'] ) ) {
$entries_args['number'] = absint( $atts['number'] );
}
// Filter the type of entries: all, unread, read, or starred
if ( $atts['type'] === 'unread' ) {
$entries_args['viewed'] = '0';
} elseif( $atts['type'] === 'read' ) {
$entries_args['viewed'] = '1';
} elseif ( $atts['type'] === 'starred' ) {
$entries_args['starred'] = '1';
}
// Get all entries for the form, according to arguments defined.
// There are many options available to query entries. To see more, check out
// the get_entries() function inside class-entry.php (https://a.cl.ly/bLuGnkGx).
$entries = json_decode(json_encode(wpforms()->entry->get_entries( $entries_args )), true);
if ( empty( $entries ) ) {
return '<p>No entries found.</p>';
}
foreach($entries as $key => $entry) {
$entries[$key]['fields'] = json_decode($entry['fields'], true);
$entries[$key]['meta'] = json_decode($entry['meta'], true);
}
if ( !empty($atts['sort']) && isset($entries[0]['fields'][$atts['sort']] ) ) {
if ( strtolower($atts['order']) == 'asc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry1['fields'][$atts['sort']]['value'], $entry2['fields'][$atts['sort']]['value']);
});
} elseif ( strtolower($atts['order']) == 'desc' ) {
usort($entries, function ($entry1, $entry2) use ($atts) {
return strcmp($entry2['fields'][$atts['sort']]['value'], $entry1['fields'][$atts['sort']]['value']);
});
}
}
ob_start();
echo '<div class="wrapper">';
echo '<div class="entries">';
// Now, loop through all the form entries.
foreach ( $entries as $entry ) {
echo '<div class="entry-details">';
$entry_fields = $entry['fields'];
foreach ( $form_fields as $form_field ) {
echo '<span class="details">';
echo '<span class="label">' . esc_html( sanitize_text_field( $form_field['label'] ) ) . ':</span>';
foreach ( $entry_fields as $entry_field ) {
if ( absint( $entry_field['id'] ) === absint( $form_field['id'] ) ) {
echo apply_filters( 'wpforms_html_field_value', wp_strip_all_tags( $entry_field['value'] ), $entry_field, $form_data, 'entry-frontend-table' );
break;
}
}
echo '</span>';
}
echo '</div>';
}
echo '</div>';
echo '</div>';
$output = ob_get_clean();
return $output;
}
add_shortcode( 'wpforms_entries_table', 'wpf_entries_table' );
非テーブル・ビューのスタイリングには、以下のCSSを使用する。
サイトにCSSを追加する方法と場所については、こちらのチュートリアルをご覧ください。
.wrapper {
width: 100%;
clear: both;
display: block;
}
span.details {
display: block;
margin-right: 2px;
margin: 5px 0;
}
.entries {
display: block;
clear: both;
width: 100%;
}
.entry-details {
border-bottom: 1px solid #ccc;
width: 100%;
display: block;
margin: 20px 0;
padding-bottom: 20px;
}
span.label {
font-weight: bold;
margin-right: 5px;
}
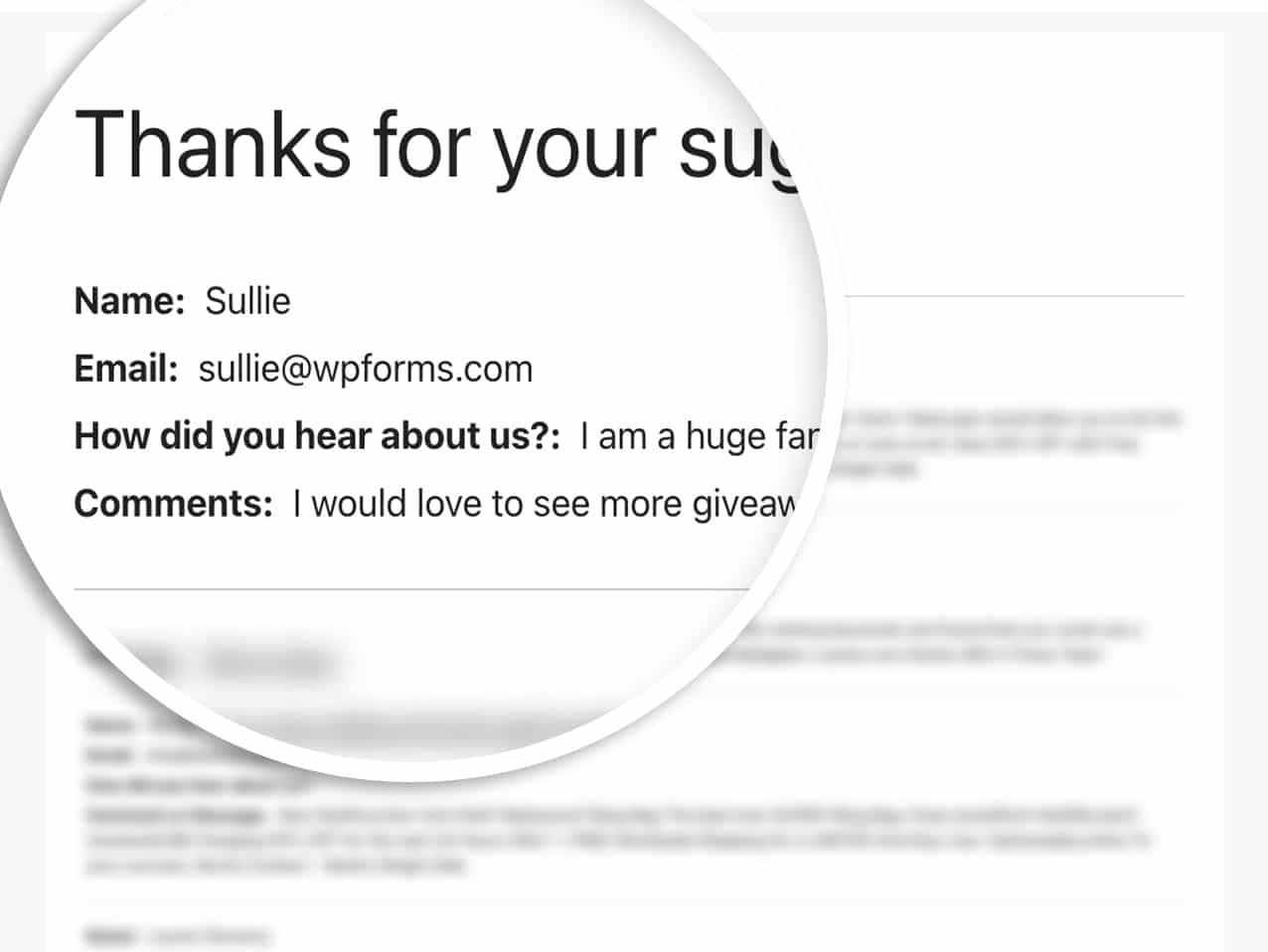