フォームの日付ピッカーに年齢制限を追加したいですか?このガイドでは、年齢を検証し、日付が要件を満たさない場合にカスタムメッセージを表示する方法を紹介します。このガイドでは、12歳から18歳までの子供だけを受け付ける子供向けバレエ教室の登録フォームを例にして説明します。
フォームの設定
最初のステップでは、新規フォームを作成し、フォームに日付/時刻フォームフィールドを追加するだけです。
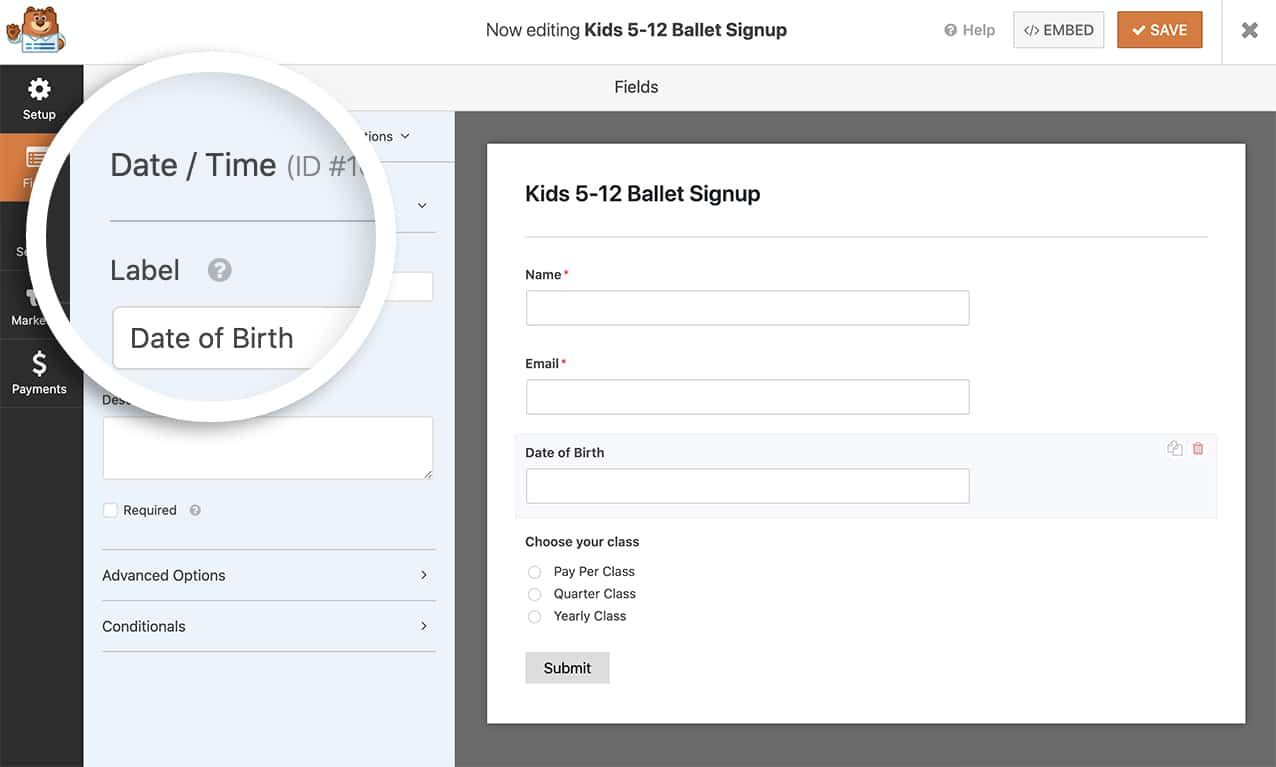
年齢制限バリデーションの追加
年齢を検証し、フォームフィールドの下にエラーメッセージを表示するには、このコードをサイトに追加してください。カスタムコードの追加方法がわからない場合は、コードスニペットの追加方法のガイドをご覧ください。
/**
* Display an error message on submission of the form if the date doesn't fall within the guidelines.
*
* @link https://wpforms.com/developers/how-to-provide-an-age-restriction-on-the-datepicker-form-field/ *
*/
function wpf_dev_process( $fields, $entry, $form_data ) {
// Optional, you can limit to specific forms. Below, we restrict output to
// form #1000.
if ( absint( $form_data[ 'id' ] ) !== 1000 ) {
return $fields;
}
if ( isset( $fields[25][ 'value' ] ) && !empty( $fields[25][ 'value' ] ) ) {
$timestamp = strtotime( $fields[25][ 'value' ] );
if ($timestamp === false) {
// Invalid date format
wpforms()->process->errors[ $form_data[ 'id' ] ][ '25' ] = esc_html__( 'Invalid date format', 'plugin-domain' );
} else {
$birth_year = date('Y', $timestamp);
$current_year = date('Y');
$age = $current_year - $birth_year;
if ($age < 12 || $age > 18) {
// Show an error message at the top of the form and under the specific field
wpforms()->process->errors[ $form_data[ 'id' ] ][ '25' ] = esc_html__( 'Minimum age requirement is 12 and maximum age requirement is 18', 'plugin-domain' );
}
}
} else {
// Date field is empty
wpforms()->process->errors[ $form_data[ 'id' ] ][ '25' ] = esc_html__( 'Date field is required', 'plugin-domain' );
}
return $fields;
}
add_action( 'wpforms_process', 'wpf_dev_process', 10, 3 );
フォームID(1000)とフィールドID(25)を自分のフォームに合わせて更新してください。これらのIDを見つけるのに助けが必要な場合は、フォームとフィールドのIDを見つけるガイドをご覧ください。
このコード・スニペットでは、日付が年齢制限に失敗した場合、Submitボタンがクリックされると、フィールドの下にメッセージが表示されます。
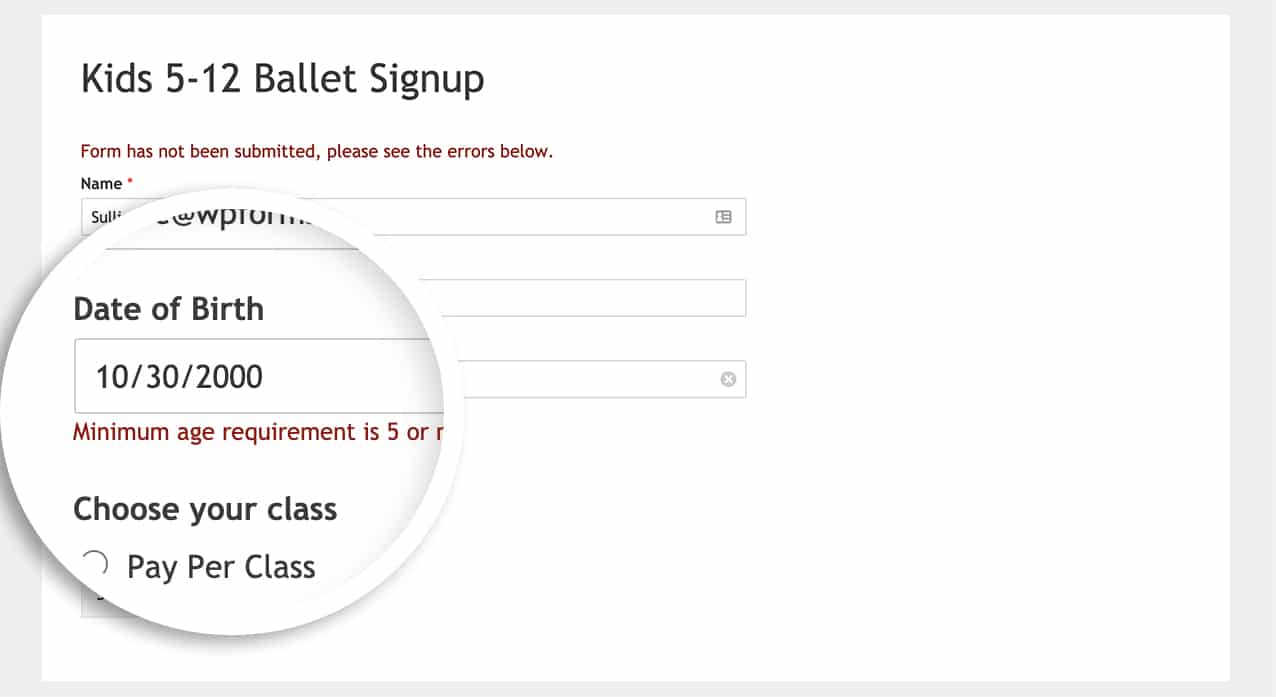
リピーター・フィールドによる年齢制限の使用
日付フィールドがリピータ・フィールドの中にある場合は、この修正版を使用してください:
/**
* Age restriction validation for Repeater fields
*/
function wpf_dev_process( $fields, $entry, $form_data ) {
if ( absint( $form_data[ 'id' ] ) !== 1000 ) {
return $fields;
}
foreach ( $fields as $field_id => $field ) {
if ( preg_match( '/^25(_\d+)?$/', $field_id ) ) {
if ( isset( $field[ 'value' ] ) && !empty( $field[ 'value' ] ) ) {
$timestamp = strtotime( $field[ 'value' ] );
if ( $timestamp === false ) {
wpforms()->process->errors[ $form_data[ 'id' ] ][ $field_id ] = esc_html__( 'Invalid date format', 'plugin-domain' );
} else {
$birth_year = date('Y', $timestamp);
$current_year = date('Y');
$age = $current_year - $birth_year;
if ( $age < 12 || $age > 18 ) {
wpforms()->process->errors[ $form_data[ 'id' ] ][ $field_id ] = esc_html__( 'Minimum age requirement is 12 and maximum age requirement is 18', 'plugin-domain' );
}
}
}
}
}
return $fields;
}
add_action( 'wpforms_process', 'wpf_dev_process', 10, 3 );
正確な日付比較の使用
ユーザーが正確に18歳以上であることを確認するなど、より正確な年齢認証を行う:
/**
* Check if the user is 18 years or older using exact date comparison
*/
function wpf_dev_compare_dates( $fields, $entry, $form_data ) {
if ( absint( $form_data[ 'id' ] ) !== 1000 ) {
return $fields;
}
$age = 18;
$date_1 = $fields[25][ 'unix' ];
if( is_string( $date_1 ) ) {
$date_1 = strtotime( $date_1 );
}
if( time() - $date_1 < $age * 31536000 )
wpforms()->process->errors[ $form_data[ 'id' ] ][ 'header' ] = esc_html__( 'Apologies, you need to be 18 or older to submit this form.', 'plugin-domain' );
}
add_action( 'wpforms_process', 'wpf_dev_compare_dates', 10, 3 );
これで完了です!これで日付ピッカー・フィールドに年齢制限を実装することができました。次に、他の方法で日付ピッカーフィールドをカスタマイズしたいですか?詳しくはチュートリアルの日付時間フィールドオプションのカスタマイズをご覧ください。